The flyweight pattern is used to decrease the number of objects created, therefore reducing memory usage and increasing performance. For example, we have a BuildingMaker that is able to build with four types of materials: brick, stone, wood, and concrete. If we want to build 500 buildings, without using the flyweight pattern, 500 BuildingMaker objects are going to be created, however only four BuildingMaker objects are needed when the flyweight pattern is used.
public class BuildingMaker {
private String material;
public BuildingMaker(String m) {
material = m;
}
public void build(int length, int width, int height) {
System.out.println("Building house with " + material);
System.out.println("The size of the house is: " + length + "x" + width + "x" + height);
}
}
public class Builder {
private numberOfBuidings = 500;
private String[] materials = {"brick", "stone", "wood", "concrete"};
private int minLength = 100;
private int minWidth = 50;
private int minHeight = 25;
public void construct() {
int len, wid, hei;
String material;
for (int i=0; i<numberOfBuidings; i++) {
len = minLength + Math.random()*20;
wid = minWidth + Math.random()*15;
hei = minHeight + Math.random()*10;
material = Math.random()*materials.length;
BuildingMaker bm = new BuildingMaker(material);//500 BuildingMakers created
bm.build(len, wid, hei);
}
}
}
//Create a BuildingMakerFactory to reuse the BuildingMaker set for a material type
public class BuildingMakerFactory {
//maps material to BuildingMaker
private HashMap<String, BuildingMaker> buildingMakerMap = new HashMap<String, BuildingMaker>();
public BuildingMaker getBuildingMaker(String material) {
BuildingMaker bm = buildingMakerMap.get(material);
if (bm == null) {
bm = new BuildingMaker(material);
buildingMakerMap.put(material, bm);
}
return bm;
}
}
public class Builder {
private numberOfBuidings = 500;
private String[] materials = {"brick", "stone", "wood", "concrete"};
private int minLength = 100;
private int minWidth = 50;
private int minHeight = 25;
private BuildingMakerFactory bmf = new BuildingMakerFactory();
public void construct() {
int len, wid, hei;
String material;
for (int i=0; i<numberOfBuidings; i++) {
len = minLength + Math.random()*20;
wid = minWidth + Math.random()*15;
hei = minHeight + Math.random()*10;
material = Math.random()*materials.length;
BuildingMaker bm = bmf.getBuildingMaker( material);//maximum 4 BuildingMakers created
bm.build(len, wid, hei);
}
}
}
---------------------------------------------------------------------------------------------------------------
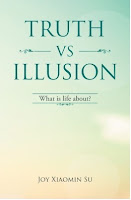
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
public class BuildingMaker {
private String material;
public BuildingMaker(String m) {
material = m;
}
public void build(int length, int width, int height) {
System.out.println("Building house with " + material);
System.out.println("The size of the house is: " + length + "x" + width + "x" + height);
}
}
1. Without using the flyweight pattern
public class Builder {
private numberOfBuidings = 500;
private String[] materials = {"brick", "stone", "wood", "concrete"};
private int minLength = 100;
private int minWidth = 50;
private int minHeight = 25;
public void construct() {
int len, wid, hei;
String material;
for (int i=0; i<numberOfBuidings; i++) {
len = minLength + Math.random()*20;
wid = minWidth + Math.random()*15;
hei = minHeight + Math.random()*10;
material = Math.random()*materials.length;
BuildingMaker bm = new BuildingMaker(material);//500 BuildingMakers created
bm.build(len, wid, hei);
}
}
}
2. Using the flyweight pattern
//Create a BuildingMakerFactory to reuse the BuildingMaker set for a material type
public class BuildingMakerFactory {
//maps material to BuildingMaker
private HashMap<String, BuildingMaker> buildingMakerMap = new HashMap<String, BuildingMaker>();
public BuildingMaker getBuildingMaker(String material) {
BuildingMaker bm = buildingMakerMap.get(material);
if (bm == null) {
bm = new BuildingMaker(material);
buildingMakerMap.put(material, bm);
}
return bm;
}
}
public class Builder {
private numberOfBuidings = 500;
private String[] materials = {"brick", "stone", "wood", "concrete"};
private int minLength = 100;
private int minWidth = 50;
private int minHeight = 25;
private BuildingMakerFactory bmf = new BuildingMakerFactory();
public void construct() {
int len, wid, hei;
String material;
for (int i=0; i<numberOfBuidings; i++) {
len = minLength + Math.random()*20;
wid = minWidth + Math.random()*15;
hei = minHeight + Math.random()*10;
material = Math.random()*materials.length;
BuildingMaker bm = bmf.getBuildingMaker( material);//maximum 4 BuildingMakers created
bm.build(len, wid, hei);
}
}
}
---------------------------------------------------------------------------------------------------------------
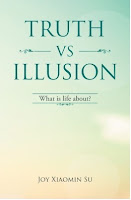
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment