A. The bits of the primitives are different in c and in Java.
Java:
byte: 8 bits/1 byte
short/char: 16 bits/2 bytes
int/float: 32 bits/4 bytes
long/double: 64 bits/8 bytes
c:
char: 8 bits/1 byte
short: 16 bits/2 bytes
int/long/float: 32 bits/4 bytes
double: 64 bits/8 bytes
B. The byte order in Unix and Windows are different. In general Unix uses the big endian and windows uses the little endian.
C. If a binary file is prepared by a c program and you are reading it in a java program, you need to adjust the read method to ensure that you read the right amount of bytes of that data type in c. If the binary file is prepared on a Unix machine and is read in a Windows system or vise versa, you need to adjust the byte order accordingly.
File theFile = new File("<fileName>");
FileInputStream fis = new FileInputStream(theFile);
byte[] byteArray = new byte[(int)theFile.length()];
fis.read(byteArray);
ByteBuffer buffer = ByteBuffer.wrap(byteArray);
//Add the following line if the binary file is prepared on a Unix machine and read on a Windows machine
buffer.order(ByteOrder.LITTLE_ENDIAN);
//Add this line if it is prepared on a Windows system and read on a Unix machine
buffer.order(ByteOrder.BIG_ENDIAN);
//Add the following line if the binary file is prepared on a Unix machine and read on a Windows machine
buffer.order(ByteOrder.LITTLE_ENDIAN);
//Add this line if it is prepared on a Windows system and read on a Unix machine
buffer.order(ByteOrder.BIG_ENDIAN);
To read a char, instead of using buffer.getChar() which reads two bytes in java, you need to use (char)buffer.get().
To read a long, instead of using buffer.getLong() which reads 8 bytes in java, you need to use (new Integer(buffer.readInt())).longValue().
References:
2. How to convert byte array or char array to string in Java
----------------------------------------------------------------------------------------------------------------
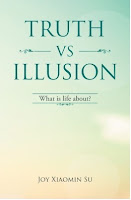
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
----------------------------------------------------------------------------------------------------------------
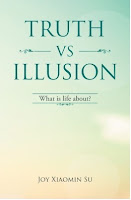
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment