A. Write to a binary stream
Assume that you have the following data that you would like to write to a binary stream.char aChar = 'T';
long aLong = 109876L;
int aInt = 98;
String aString = "Nice"; //4 chars
1. Create the ByteBuffer
In java, char is 2 bytes, long is 8 bytes, int is 4 bytes. For the above data, we need in total 22 bytes.
ByteBuffer buffer = ByteBuffer.allocate(22);
2. Put the data to the ByteBuffer
buffer.putChar(aChar);
buffer.putLong(aLong);
buffer.putInt(aInt);
buffer.put(aString.getBytes());
3. Write to the OutputStream
The code here is an example of how to write to a FileOutputStream.
FileOutputStream fos = new FileOutputStream("<fileName>");
fos.write(buffer.array());
B. Read from a binary stream
The sample code shows how to read from the binary file created above.1. Create/get the InputStream
File theFile = new File("<fileName>");
FileInputStream fis = new FileInputStream(theFile);
2. Read data into a byte array
byte[] byteArray = new byte[(int)theFile.length()];
fis.read(byteArray);
3. Get the data
ByteBuffer buffer = ByteBuffer.wrap(byteArray);
char aChar = buffer.getChar();
long aLong = buffer.getLong();
int aInt = buffer.getInt();
String aString = "";
for (int i=0; i<4; i++) {
sString += buffer.getChar();
}
References:
1. How to convert byte array or char array to string in Java
2. Java how to read a binary file prepared by a c program
------------------------------------------------------------------------------------------------------------------
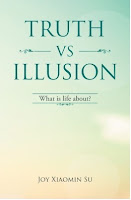
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment