Following is a sample code of creating a subreport that includes the name and price in the parent column Objects.
import static net.sf.dynamicreports.report.builder.DynamicReports.*;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import net.sf.dynamicreports.examples.Templates;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import net.sf.dynamicreports.report.base.expression.AbstractSimpleExpression;
import net.sf.dynamicreports.report.builder.DynamicReports;
import net.sf.dynamicreports.report.builder.component.SubreportBuilder;
import net.sf.dynamicreports.report.builder.style.Styles;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
import net.sf.dynamicreports.report.definition.ReportParameters;
import net.sf.dynamicreports.report.exception.DRException;
import net.sf.jasperreports.engine.JRDataSource;
import net.sf.jasperreports.engine.data.JRBeanCollectionDataSource;
import net.sf.jasperreports.engine.data.JRMapCollectionDataSource;
public class ColumnSubreportTest {
public ColumnSubreportTest() {
SubreportBuilder subreport = cmp.subreport(new SubreportDesign())
.setDataSource(new SubreportData());
JasperReportBuilder report = DynamicReports.report();
try {
report.fields(field("objects", List.class))
.columns(
col.column("Category", "category", type.stringType()),
col.column("Health Index", "healthIndex", type.integerType()),
col.componentColumn("Objects", subreport)
.setFixedWidth(240)
.setStyle(Styles.style()
.setBottomPadding(15))
.setTitleStyle(Styles.style()
.bold().setHorizontalAlignment(HorizontalAlignment.LEFT)));
report.setColumnTitleStyle(Styles.style().bold()
.setHorizontalAlignment(HorizontalAlignment.LEFT));
report.setColumnStyle(Styles.style()
.setHorizontalAlignment(HorizontalAlignment.LEFT));
report.title(cmp.text("List of real food")
.setStyle(Styles.style().bold().setFontSize(14)
.setHorizontalAlignment(HorizontalAlignment.CENTER)
.setBottomPadding(20)))
.pageFooter(Templates.footerComponent);
report.setDataSource(createDataSource());
report.show();
} catch (DRException e) {
e.printStackTrace();
}
}
private class SubreportDesign extends AbstractSimpleExpression<JasperReportBuilder> {
private static final long serialVersionUID = 1L;
@Override
public JasperReportBuilder evaluate(ReportParameters reportParameters) {
JasperReportBuilder report = report()
.columns(col.column("name", type.stringType()),
col.column("price", type.stringType()));
return report;
}
}
private class SubreportData extends AbstractSimpleExpression<JRDataSource> {
@Override
public JRDataSource evaluate(ReportParameters reportParameters) {
Collection<Map<String, ?>> value = reportParameters.getValue("objects");
return new JRMapCollectionDataSource(value);
}
}
private JRDataSource createDataSource() {
ArrayList<ReportData> datasource = new ArrayList<ReportData>();
ReportData data = new ReportData();
data.setCategory("Fruit");
data.setHealthIndex(95);
List<Map<String, Object>> objects = new ArrayList<Map<String, Object>>();
Map<String, Object> values = new HashMap<String, Object>();
values.put("name", "Apple");
values.put("price", "$1.65/lb");
objects.add(values);
values = new HashMap<String, Object>();
values.put("name", "Papaya");
values.put("price", "$1.98/lb");
objects.add(values);
data.setObjects(objects);
datasource.add(data);
data = new ReportData();
data.setCategory("Rice");
data.setHealthIndex(70);
objects = new ArrayList<Map<String, Object>>();
values = new HashMap<String, Object>();
values.put("name", "Brown Rice");
values.put("price", "$0.35/lb");
objects.add(values);
values = new HashMap<String, Object>();
values.put("name", "White Rice");
values.put("price", "$0.15/lb");
objects.add(values);
data.setObjects(objects);
datasource.add(data);
return new JRBeanCollectionDataSource(datasource);
}
//It is important to let this inner class to be public
public class ReportData {
private String category;
private Integer hi;
private List<Map<String, Object>> objects;
public String getCategory() {
return category;
}
public void setCategory(String c) {
category = c;
}
public Integer getHealthIndex() {
return hi;
}
public void setHealthIndex(Integer i) {
hi = i;
}
public List<Map<String, Object>> getObjects() {
return objects;
}
public void setObjects(List<Map<String, Object>> o) {
objects = o;
}
}
public static void main(String[] args) {
new ColumnSubreportTest();
}
}
> Next
------------------------------------------------------------------------------------------------------------------
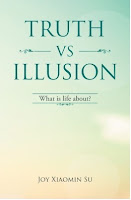
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
----------------------------------------------------------------------------------------------------------------------
2. DynamicReports Page subreport - create subreport in a page of the parent report and set report/subreport/component border
import static net.sf.dynamicreports.report.builder.DynamicReports.*;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import net.sf.dynamicreports.examples.Templates;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import net.sf.dynamicreports.report.base.expression.AbstractSimpleExpression;
import net.sf.dynamicreports.report.builder.DynamicReports;
import net.sf.dynamicreports.report.builder.component.SubreportBuilder;
import net.sf.dynamicreports.report.builder.style.Styles;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
import net.sf.dynamicreports.report.definition.ReportParameters;
import net.sf.dynamicreports.report.exception.DRException;
import net.sf.jasperreports.engine.JRDataSource;
import net.sf.jasperreports.engine.data.JRBeanCollectionDataSource;
import net.sf.jasperreports.engine.data.JRMapCollectionDataSource;
public class ColumnSubreportTest {
public ColumnSubreportTest() {
SubreportBuilder subreport = cmp.subreport(new SubreportDesign())
.setDataSource(new SubreportData());
JasperReportBuilder report = DynamicReports.report();
try {
report.fields(field("objects", List.class))
.columns(
col.column("Category", "category", type.stringType()),
col.column("Health Index", "healthIndex", type.integerType()),
col.componentColumn("Objects", subreport)
.setFixedWidth(240)
.setStyle(Styles.style()
.setBottomPadding(15))
.setTitleStyle(Styles.style()
.bold().setHorizontalAlignment(HorizontalAlignment.LEFT)));
report.setColumnTitleStyle(Styles.style().bold()
.setHorizontalAlignment(HorizontalAlignment.LEFT));
report.setColumnStyle(Styles.style()
.setHorizontalAlignment(HorizontalAlignment.LEFT));
report.title(cmp.text("List of real food")
.setStyle(Styles.style().bold().setFontSize(14)
.setHorizontalAlignment(HorizontalAlignment.CENTER)
.setBottomPadding(20)))
.pageFooter(Templates.footerComponent);
report.setDataSource(createDataSource());
report.show();
} catch (DRException e) {
e.printStackTrace();
}
}
private class SubreportDesign extends AbstractSimpleExpression<JasperReportBuilder> {
private static final long serialVersionUID = 1L;
@Override
public JasperReportBuilder evaluate(ReportParameters reportParameters) {
JasperReportBuilder report = report()
.columns(col.column("name", type.stringType()),
col.column("price", type.stringType()));
return report;
}
}
private class SubreportData extends AbstractSimpleExpression<JRDataSource> {
@Override
public JRDataSource evaluate(ReportParameters reportParameters) {
Collection<Map<String, ?>> value = reportParameters.getValue("objects");
return new JRMapCollectionDataSource(value);
}
}
private JRDataSource createDataSource() {
ArrayList<ReportData> datasource = new ArrayList<ReportData>();
ReportData data = new ReportData();
data.setCategory("Fruit");
data.setHealthIndex(95);
List<Map<String, Object>> objects = new ArrayList<Map<String, Object>>();
Map<String, Object> values = new HashMap<String, Object>();
values.put("name", "Apple");
values.put("price", "$1.65/lb");
objects.add(values);
values = new HashMap<String, Object>();
values.put("name", "Papaya");
values.put("price", "$1.98/lb");
objects.add(values);
data.setObjects(objects);
datasource.add(data);
data = new ReportData();
data.setCategory("Rice");
data.setHealthIndex(70);
objects = new ArrayList<Map<String, Object>>();
values = new HashMap<String, Object>();
values.put("name", "Brown Rice");
values.put("price", "$0.35/lb");
objects.add(values);
values = new HashMap<String, Object>();
values.put("name", "White Rice");
values.put("price", "$0.15/lb");
objects.add(values);
data.setObjects(objects);
datasource.add(data);
return new JRBeanCollectionDataSource(datasource);
}
//It is important to let this inner class to be public
public class ReportData {
private String category;
private Integer hi;
private List<Map<String, Object>> objects;
public String getCategory() {
return category;
}
public void setCategory(String c) {
category = c;
}
public Integer getHealthIndex() {
return hi;
}
public void setHealthIndex(Integer i) {
hi = i;
}
public List<Map<String, Object>> getObjects() {
return objects;
}
public void setObjects(List<Map<String, Object>> o) {
objects = o;
}
}
public static void main(String[] args) {
new ColumnSubreportTest();
}
}
> Next
------------------------------------------------------------------------------------------------------------------
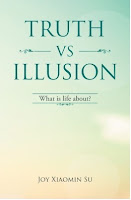
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
----------------------------------------------------------------------------------------------------------------------
References:
1. Row Subreport - create subreport in a row of the parent report2. DynamicReports Page subreport - create subreport in a page of the parent report and set report/subreport/component border
No comments:
Post a Comment