Assume that you have two tables, Fruit and Sales, in your database.
Table Fruit
name price popularIndex freshDays
Apple 1.68 10 20
Orange 0,96 5 8
Table Sales
saleDate fruitName amount customer charge payment balance
01/02/2013 Apple 1000 uphill fruit store 1680 1680 0
05/12/2014 Orange 2000 delicious 1920 1000 920
Now, you would like to have a report to list all the data in the Fruit table, and under each fruit list 10 of the most recent sales
Following is a sample code for creating the report.
public class FruitReportTest {
private JasperReport buildFruitReport() {
JasperReportBuilder report = DynamicReports.report();
report.columns(col.column("Name", "name", type.stringType()),
col.column("Price", "price", type.doubleType()),
col.columns("Popular Index", "popIndx", type.integerType()),
col.columns("Fresh Days", "freshDays", type.integerType()));
return report;
}
private JasperReportBuilder buildSalesReport() {
JasperReportBuilder report = DynamicReports.report();
report.columns(col.column("Sale Date", "saleDate", type.stringType()),
col.column("Amount", "amount", type.doubleType()),
col.columns("Customer", "customer", type.stringType()),
col.columns("Charge", "charge", type.doubleType()),
col.columns("Payment", "payment", type.doubleType()),
col.columns("Balance", "balance", type.doubleType()));
return report;
}
public class ReportData {
private String name;
private Double price;
private Integer popIndx;
private Integer freshDays;
private List<Map<String, Object>> salesData;
//getters and setters for all the above fields
.............
}
private JRDataSource createDataSource() {
Connection conn = <your method of getting database connection>
String sql1 = "Select * from Fruit";
PreparedStatement ps1 = conn.prepareStatement(sql1);
ResultSet rs = ps1.executeQuery();
String sql2 = "select * from Sales where fruitName = ?";
preparedStatement ps2 = conn.prepareStatement(sql2)
List<ReportData> datasource = new ArrayList<ReportData>();
try {
while (rs.next()) {
ReportData data = new ReportData();
data.setName(rs.getString(1));
data.setPrice(rs.getDouble(2));
data.setPopIndx(rs.getInt(3));
data.setFreshDays(rs.getInt(4));
ps2.setString(1, rs.getString(1));
ResultSet rs2 = ps2.executeQuery();
List<Map<String, Object>> salesData = new ArrayList<Map<String, Object>>();
Map<String, Object> sd = new HashMap<String, Object>();
while (rs2.next()){
ds.put("saleDate", rs2.getString(1));
ds.put("amount", rs2.getDouble(3));
ds.put("customer", rs2.getString(4));
ds.put("charge", rs2.getDouble(5));
ds.put("payment", rs2.getDouble(6));
ds.put("balance", rs2.getDouble(7));
salesData.add(ds);
}
data.setSalesData(salesData);
rs2.close();
}
ps2.close();
rs.close();
ps1.close();
} catch (SQLException se) {
se.printStackTrace();
}
conn.close();
return new JRBeanCollectionDataSource(datasource);
}
public static void main(String[] args) {
FruitReportTest test = new FruitReportTest();
JasperReportBuilder report = test.buildFruitReport();
SubreportBuilder subreport = cmp.subreport(test.buildSalesReport())
.setDataSource(exp.subDatasourceBeanCollection("salesData"));
report.detailFooter(cmp.horizontalList(cmp.horizontalGap(10), subreport, cmp.horizontalGap(10)));
report.setDataSource(createDataSource());
report.show();
}
}
Previous < > Next
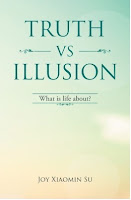
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
2. DynamicReports Page subreport - create subreport in a page of the parent report and set report/subreport/component border
Table Fruit
name price popularIndex freshDays
Apple 1.68 10 20
Orange 0,96 5 8
Table Sales
saleDate fruitName amount customer charge payment balance
01/02/2013 Apple 1000 uphill fruit store 1680 1680 0
05/12/2014 Orange 2000 delicious 1920 1000 920
Now, you would like to have a report to list all the data in the Fruit table, and under each fruit list 10 of the most recent sales
Following is a sample code for creating the report.
public class FruitReportTest {
private JasperReport buildFruitReport() {
JasperReportBuilder report = DynamicReports.report();
report.columns(col.column("Name", "name", type.stringType()),
col.column("Price", "price", type.doubleType()),
col.columns("Popular Index", "popIndx", type.integerType()),
col.columns("Fresh Days", "freshDays", type.integerType()));
return report;
}
private JasperReportBuilder buildSalesReport() {
JasperReportBuilder report = DynamicReports.report();
report.columns(col.column("Sale Date", "saleDate", type.stringType()),
col.column("Amount", "amount", type.doubleType()),
col.columns("Customer", "customer", type.stringType()),
col.columns("Charge", "charge", type.doubleType()),
col.columns("Payment", "payment", type.doubleType()),
col.columns("Balance", "balance", type.doubleType()));
return report;
}
public class ReportData {
private String name;
private Double price;
private Integer popIndx;
private Integer freshDays;
private List<Map<String, Object>> salesData;
//getters and setters for all the above fields
.............
}
private JRDataSource createDataSource() {
Connection conn = <your method of getting database connection>
String sql1 = "Select * from Fruit";
PreparedStatement ps1 = conn.prepareStatement(sql1);
ResultSet rs = ps1.executeQuery();
String sql2 = "select * from Sales where fruitName = ?";
preparedStatement ps2 = conn.prepareStatement(sql2)
List<ReportData> datasource = new ArrayList<ReportData>();
try {
while (rs.next()) {
ReportData data = new ReportData();
data.setName(rs.getString(1));
data.setPrice(rs.getDouble(2));
data.setPopIndx(rs.getInt(3));
data.setFreshDays(rs.getInt(4));
ps2.setString(1, rs.getString(1));
ResultSet rs2 = ps2.executeQuery();
List<Map<String, Object>> salesData = new ArrayList<Map<String, Object>>();
Map<String, Object> sd = new HashMap<String, Object>();
while (rs2.next()){
ds.put("saleDate", rs2.getString(1));
ds.put("amount", rs2.getDouble(3));
ds.put("customer", rs2.getString(4));
ds.put("charge", rs2.getDouble(5));
ds.put("payment", rs2.getDouble(6));
ds.put("balance", rs2.getDouble(7));
salesData.add(ds);
}
data.setSalesData(salesData);
rs2.close();
}
ps2.close();
rs.close();
ps1.close();
} catch (SQLException se) {
se.printStackTrace();
}
conn.close();
return new JRBeanCollectionDataSource(datasource);
}
public static void main(String[] args) {
FruitReportTest test = new FruitReportTest();
JasperReportBuilder report = test.buildFruitReport();
SubreportBuilder subreport = cmp.subreport(test.buildSalesReport())
.setDataSource(exp.subDatasourceBeanCollection("salesData"));
report.detailFooter(cmp.horizontalList(cmp.horizontalGap(10), subreport, cmp.horizontalGap(10)));
report.setDataSource(createDataSource());
report.show();
}
}
Previous < > Next
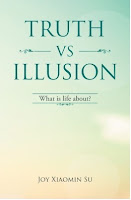
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Reference:
1. DynamicReports: Column Subreport - create subreport in a column of the parent report2. DynamicReports Page subreport - create subreport in a page of the parent report and set report/subreport/component border
No comments:
Post a Comment