There are two ways to make a currency column to automatically display the currency symbol. One is to create a new currency type and set the column's data type to that currency type. The other way is to define a pattern of how you would like the column to display the data. The advantage of doing it in these ways instead of converting the data to a string and attach the money symbol in front of it is that it not only shows that the column is holding an amount of money, what type of money it is and you can at the same time use the column to do mathematical calculations.
import java.awt.Color;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.math.BigDecimal;
import java.text.SimpleDateFormat;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import net.sf.dynamicreports.report.builder.DynamicReports;
import net.sf.dynamicreports.report.builder.column.Columns;
import net.sf.dynamicreports.report.builder.column.TextColumnBuilder;
import net.sf.dynamicreports.report.builder.component.Components;
import net.sf.dynamicreports.report.builder.datatype.BigDecimalType;
import net.sf.dynamicreports.report.builder.datatype.DataTypes;
import net.sf.dynamicreports.report.builder.style.StyleBuilder;
import net.sf.dynamicreports.report.builder.style.Styles;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
import net.sf.dynamicreports.report.constant.PageType;
import net.sf.dynamicreports.report.datasource.DRDataSource;
import net.sf.dynamicreports.report.exception.DRException;
import net.sf.jasperreports.engine.JRDataSource;
public class DynamicReportsTest {
public DynamicReportsTest() {
build();
}
public void build() {
JasperReportBuilder report = DynamicReports.report();
report.setPageFormat(PageType.LETTER);
// report.setPageFormat(PageType.A4, PageOrientation.PORTRAIT);
//Create the Currency Object
CurrencyType currencyType = new CurrencyType();
//Set the currency type
TextColumnBuilder<BigDecimal> priceColumn = Columns.column("Price", "price", currencyType);
TextColumnBuilder<Integer> quantityOrderedColumn = Columns.column("Quantity Ordered", "quantityOrdered", DataTypes.integerType());
TextColumnBuilder<BigDecimal> totalPay = priceColumn.multiply(quantityOrderedColumn)
//Set the currency pattern
.setTitle("Amount Paid")
.setPattern(currencyType.getPattern());
// .setPattern("$#,###,###.00");
report.columns(Columns.columnRowNumberColumn("Item"),
Columns.column("Name", "name", DataTypes.stringType()),
priceColumn,
quantityOrderedColumn,
totalPay
);
StyleBuilder bold = Styles.style().bold();
StyleBuilder centeredBold = Styles.style(bold)
.setHorizontalAlignment(HorizontalAlignment.CENTER);
StyleBuilder columnStyle = Styles.style(centeredBold)
.setBackgroundColor(Color.LIGHT_GRAY)
.setBorder(Styles.pen1Point());
report.setColumnTitleStyle(columnStyle);
report.setColumnStyle(Styles.style().setHorizontalAlignment(HorizontalAlignment.CENTER));
report.highlightDetailEvenRows();
report.title(Components.text("Test Report")
.setStyle(centeredBold));
report.title(Components.text("Detailed Report")
.setStyle(centeredBold));
report.pageFooter(Components.horizontalFlowList().add(Components.text("Page "))
.add(Components.pageNumber())
.setStyle(bold));
report.setDataSource(createDataSource());
try {
report.show();
// java.io.File file = new java.io.File("<your file path and name>.pdf");
java.io.File file = new java.io.File("<your file path and name>.txt");
// report.toPdf(new FileOutputStream(file));
report.toText(new FileOutputStream(file));
}catch(DRException e){
e.printStackTrace();
} catch (FileNotFoundException fe){
fe.printStackTrace();
}
}
private class CurrencyType extends BigDecimalType {
@Override
public String getPattern() {
if (usaMoney) {
return "$ #,###.00";
} else if (ukMoney){
return "£ #,###.00";
} else if (euroMoney) {
return "€ #,###.00";
}
return "¥ #,###.00";
}
}
private JRDataSource createDataSource() {
DRDataSource dataSource = new DRDataSource("name", "price", "quantityOrdered");
dataSource.add("Apple", new BigDecimal(1.29), 120);
dataSource.add("Apple", new BigDecimal(1.69), 150);
dataSource.add("Orange", new BigDecimal(0.99), 130);
dataSource.add("Orange", new BigDecimal(0.96), 100);
dataSource.add("Mange", new BigDecimal(2.99), 300);
return dataSource;
}
public static void main(String[] args){
new DynamicReportsTest();
}
}
------------------------------------------------------------------------------------------------------------
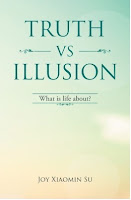
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
import java.awt.Color;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.math.BigDecimal;
import java.text.SimpleDateFormat;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import net.sf.dynamicreports.report.builder.DynamicReports;
import net.sf.dynamicreports.report.builder.column.Columns;
import net.sf.dynamicreports.report.builder.column.TextColumnBuilder;
import net.sf.dynamicreports.report.builder.component.Components;
import net.sf.dynamicreports.report.builder.datatype.BigDecimalType;
import net.sf.dynamicreports.report.builder.datatype.DataTypes;
import net.sf.dynamicreports.report.builder.style.StyleBuilder;
import net.sf.dynamicreports.report.builder.style.Styles;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
import net.sf.dynamicreports.report.constant.PageType;
import net.sf.dynamicreports.report.datasource.DRDataSource;
import net.sf.dynamicreports.report.exception.DRException;
import net.sf.jasperreports.engine.JRDataSource;
public class DynamicReportsTest {
public DynamicReportsTest() {
build();
}
public void build() {
JasperReportBuilder report = DynamicReports.report();
report.setPageFormat(PageType.LETTER);
// report.setPageFormat(PageType.A4, PageOrientation.PORTRAIT);
//Create the Currency Object
CurrencyType currencyType = new CurrencyType();
//Set the currency type
TextColumnBuilder<BigDecimal> priceColumn = Columns.column("Price", "price", currencyType);
TextColumnBuilder<Integer> quantityOrderedColumn = Columns.column("Quantity Ordered", "quantityOrdered", DataTypes.integerType());
TextColumnBuilder<BigDecimal> totalPay = priceColumn.multiply(quantityOrderedColumn)
//Set the currency pattern
.setTitle("Amount Paid")
.setPattern(currencyType.getPattern());
// .setPattern("$#,###,###.00");
report.columns(Columns.columnRowNumberColumn("Item"),
Columns.column("Name", "name", DataTypes.stringType()),
priceColumn,
quantityOrderedColumn,
totalPay
);
StyleBuilder bold = Styles.style().bold();
StyleBuilder centeredBold = Styles.style(bold)
.setHorizontalAlignment(HorizontalAlignment.CENTER);
StyleBuilder columnStyle = Styles.style(centeredBold)
.setBackgroundColor(Color.LIGHT_GRAY)
.setBorder(Styles.pen1Point());
report.setColumnTitleStyle(columnStyle);
report.setColumnStyle(Styles.style().setHorizontalAlignment(HorizontalAlignment.CENTER));
report.highlightDetailEvenRows();
report.title(Components.text("Test Report")
.setStyle(centeredBold));
report.title(Components.text("Detailed Report")
.setStyle(centeredBold));
report.pageFooter(Components.horizontalFlowList().add(Components.text("Page "))
.add(Components.pageNumber())
.setStyle(bold));
report.setDataSource(createDataSource());
try {
report.show();
// java.io.File file = new java.io.File("<your file path and name>.pdf");
java.io.File file = new java.io.File("<your file path and name>.txt");
// report.toPdf(new FileOutputStream(file));
report.toText(new FileOutputStream(file));
}catch(DRException e){
e.printStackTrace();
} catch (FileNotFoundException fe){
fe.printStackTrace();
}
}
private class CurrencyType extends BigDecimalType {
@Override
public String getPattern() {
if (usaMoney) {
return "$ #,###.00";
} else if (ukMoney){
return "£ #,###.00";
} else if (euroMoney) {
return "€ #,###.00";
}
return "¥ #,###.00";
}
}
private JRDataSource createDataSource() {
DRDataSource dataSource = new DRDataSource("name", "price", "quantityOrdered");
dataSource.add("Apple", new BigDecimal(1.29), 120);
dataSource.add("Apple", new BigDecimal(1.69), 150);
dataSource.add("Orange", new BigDecimal(0.99), 130);
dataSource.add("Orange", new BigDecimal(0.96), 100);
dataSource.add("Mange", new BigDecimal(2.99), 300);
return dataSource;
}
public static void main(String[] args){
new DynamicReportsTest();
}
}
------------------------------------------------------------------------------------------------------------
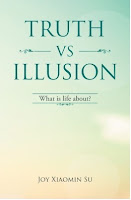
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Hi mam, I have doubt regarding Converting String To BigDecimal Value as Currency Type
ReplyDeleteHow will i do that
Example :
1 step :- Converting Double value to String type By using value formatter for when value became 0 that time i has to display Empty so i used value formatter
private static class ValueFormatter extends AbstractValueFormatter {
private static final long serialVersionUID = 1L;
@Override
public String format(BigDecimal value, ReportParameters reportParameters) {
if(value.compareTo(BigDecimal.ZERO==0){
return "";
}
return value+""; }
}
Above code will return as String value
2 step :-
Again That String value i has to convert as Currency Type how to do that...
private class CurrencyType extends BigDecimalType {
private static final long serialVersionUID = 1L;
@Override
public String getPattern() {
return "$ #,###.00";
}
}
If you use the example code in this post above, you do not have to convert anything particularly. All you need to do is to create a CurrencyType class that extends the BigDecimalType class and set the data type of the currency column to the instance of this CurrencyType class. That is all you need to do, no conversion is required.
ReplyDelete