To set focus to a field in a java swing user interface, you can use either the requestFocusInWindow method of java.awt.Component or the java.awt.FocusTraversalPolicy and the setFocusTraversalPolicy method of java.awt.Component.
The requestFocusInWindow method works only after the layout of the component that is going to gain focus in the window/frame is complete, which means it works only after the pack method is called. This can be achieved by either putting the requestFocusInWindow method after the pack method or using the invokeLater method of SwingUtilities to call the requestFocusInWindow method.
Following is a sample code.
import java.awt.GridLayout;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class FocusTest extends JFrame {
public FocusTest() {
getContentPane().setLayout(new GridLayout(2,2));
JLabel label1 = new JLabel("Input 1: ");
JLabel label2 = new JLabel("Input 2: ");
JTextField f1 = new JTextField(15);
JTextField f2 = new JTextField(20);
add(label1);
add(f1);
add(label2);
add(f2);
//the following will not work
//f2.requestFocusInWindow();
//the following will work
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
f2.requestFocusInWindow();
}
});
pack();
//the following will work
//f2.requestFocusInWindow();
setVisible(true);
}
public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new FocusTest();
}
}
);
}
}
> Next
--------------------------------------------------------------------------------------------------------
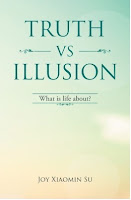
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
The requestFocusInWindow method works only after the layout of the component that is going to gain focus in the window/frame is complete, which means it works only after the pack method is called. This can be achieved by either putting the requestFocusInWindow method after the pack method or using the invokeLater method of SwingUtilities to call the requestFocusInWindow method.
Following is a sample code.
import java.awt.GridLayout;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class FocusTest extends JFrame {
public FocusTest() {
getContentPane().setLayout(new GridLayout(2,2));
JLabel label1 = new JLabel("Input 1: ");
JLabel label2 = new JLabel("Input 2: ");
JTextField f1 = new JTextField(15);
JTextField f2 = new JTextField(20);
add(label1);
add(f1);
add(label2);
add(f2);
//the following will not work
//f2.requestFocusInWindow();
//the following will work
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
f2.requestFocusInWindow();
}
});
pack();
//the following will work
//f2.requestFocusInWindow();
setVisible(true);
}
public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new FocusTest();
}
}
);
}
}
> Next
Reference:
1. Set focus to a field in window/frame (2): FocusTraversalPolicy--------------------------------------------------------------------------------------------------------
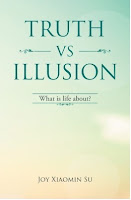
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment