To execute certain code after all the other code in the same method/constructor has been executed, you need to create a new thread and make the new thread to wait util the parent thread finishes execution.
Following is an example.
import java.awt.Component;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.FocusAdapter;
import java.awt.event.FocusEvent;
import java.util.ArrayList;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class FocusTest extends JFrame {
JTextField f1 = null;
JButton b1 = null;
public FocusTest() {
getContentPane().setLayout(new GridLayout(2,2));
JLabel label1 = new JLabel("Input 1: ");
f1 = new JTextField(15);
b1 = new JButton("button 1");
add(label1);
add(f1);
add(b1);
ArrayList<Component> tp = new ArrayList<Component>();
tp.add(f1);
tp.add(b1);
//the following code will not work
//because the layout of button 1 is not realized
//b1.requestFocusInWindow();
//request focus on button 1
//after all the other code in the constructor is executed.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
b1.requestFocusInWindow();
}
});
b1.addActionListener(new ButtonListener());
f1.addFocusListener(new F1FocusListener());
b1.setEnabled(false);
pack();
setVisible(true);
}
private class F1FocusListener extends FocusAdapter {
public void focusGained(FocusEvent e){
if (e.getSource().equals(f1)){
//because button 1 is disabled
//the following code will do nothing
//b1.doClick();
//will programmatically click button 1
//after all the other code in the method is executed.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
b1.doClick();
}
});
b1.setEnabled(true);
}
}
}
private class ButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e){
if (e.getSource() == b1){
f1.setText("Excellent job!!!!");
}
}
}
public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new FocusTest();
}
}
);
}
}
-------------------------------------------------------------------------------------------------------------
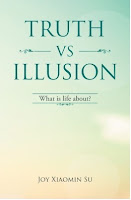
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Following is an example.
import java.awt.Component;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.FocusAdapter;
import java.awt.event.FocusEvent;
import java.util.ArrayList;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class FocusTest extends JFrame {
JTextField f1 = null;
JButton b1 = null;
public FocusTest() {
getContentPane().setLayout(new GridLayout(2,2));
JLabel label1 = new JLabel("Input 1: ");
f1 = new JTextField(15);
b1 = new JButton("button 1");
add(label1);
add(f1);
add(b1);
ArrayList<Component> tp = new ArrayList<Component>();
tp.add(f1);
tp.add(b1);
//the following code will not work
//because the layout of button 1 is not realized
//b1.requestFocusInWindow();
//request focus on button 1
//after all the other code in the constructor is executed.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
b1.requestFocusInWindow();
}
});
b1.addActionListener(new ButtonListener());
f1.addFocusListener(new F1FocusListener());
b1.setEnabled(false);
pack();
setVisible(true);
}
private class F1FocusListener extends FocusAdapter {
public void focusGained(FocusEvent e){
if (e.getSource().equals(f1)){
//because button 1 is disabled
//the following code will do nothing
//b1.doClick();
//will programmatically click button 1
//after all the other code in the method is executed.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
b1.doClick();
}
});
b1.setEnabled(true);
}
}
}
private class ButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e){
if (e.getSource() == b1){
f1.setText("Excellent job!!!!");
}
}
}
public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new FocusTest();
}
}
);
}
}
-------------------------------------------------------------------------------------------------------------
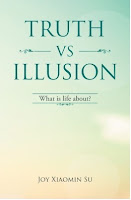
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment