The FocusTraversalPolicy controls the sequence of fields gaining focus when you tapping through the fields and also the field that has the focus when the window/frame is first constructed/activated/visible.
Following is a sample code.
import java.awt.Component;
import java.awt.Container;
import java.awt.FocusTraversalPolicy;
import java.awt.GridLayout;
import java.awt.Window;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class FocusTest extends JFrame {
JTextField f1 = null;
JTextField f2 = null;
JButton b1 = null;
JButton b2 = null;
public FocusTest() {
getContentPane().setLayout(new GridLayout(3,2));
JLabel label1 = new JLabel("Input 1: ");
JLabel label2 = new JLabel("Input 2: ");
f1 = new JTextField(15);
f2 = new JTextField(20);
b1 = new JButton("button 1");
b2 = new JButton("button 2");
add(label1);
add(f1);
add(label2);
add(f2);
add(b1);
add(b2);
b1.addActionListener(new ButtonListener());
b2.addActionListener(new ButtonListener());
ArrayList<Component> tp = new ArrayList<Component>();
tp.add(f1);
tp.add(f2);
tp.add(b1);
tp.add(b2);
setFocusTraversalPolicyProvider(true);
setFocusTraversalPolicy(new MyTraversalPolicy(tp));
pack();
setVisible(true);
}
private class MyTraversalPolicy extends FocusTraversalPolicy {
ArrayList<Component> components = null;
public MyTraversalPolicy(ArrayList<Component> list){
this.components = list;
}
public Component getComponentAfter(Container focusCycleRoot, Component focused){
int idx = components.indexOf(focused);
if (idx >= components.size()-1){
idx = -1;
}
return components.get(idx+1);
}
public Component getComponentBefore(Container c, Component focused){
int idx = components.indexOf(focused);
if (idx <= 0){
idx = components.size();
}
return components.get(idx-1);
}
public Component getDefaultComponent(Container c){
return f2;
}
public Component getFirstComponent(Container c){
return components.get(0);
}
//Set the field of focus
//when the window is first activated/contructed/visible
public Component getInitialComponent(Window w){
return f2;
}
public Component getLastComponent(Container c){
return components.get(components.size()-1);
}
}
private class ButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e){
if (e.getSource() == b1){
f1.setText("button 1 is pressed");
f2.setText("");
} else if (e.getSource() == b2){
f2.setText("button 2 is pressed");
f1.setText("");
}
}
}
public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new FocusTest();
}
}
);
}
}
Previous <
--------------------------------------------------------------------------------------------------------------
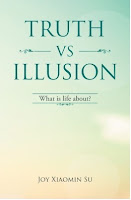
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Following is a sample code.
import java.awt.Component;
import java.awt.Container;
import java.awt.FocusTraversalPolicy;
import java.awt.GridLayout;
import java.awt.Window;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class FocusTest extends JFrame {
JTextField f1 = null;
JTextField f2 = null;
JButton b1 = null;
JButton b2 = null;
public FocusTest() {
getContentPane().setLayout(new GridLayout(3,2));
JLabel label1 = new JLabel("Input 1: ");
JLabel label2 = new JLabel("Input 2: ");
f1 = new JTextField(15);
f2 = new JTextField(20);
b1 = new JButton("button 1");
b2 = new JButton("button 2");
add(label1);
add(f1);
add(label2);
add(f2);
add(b1);
add(b2);
b1.addActionListener(new ButtonListener());
b2.addActionListener(new ButtonListener());
ArrayList<Component> tp = new ArrayList<Component>();
tp.add(f1);
tp.add(f2);
tp.add(b1);
tp.add(b2);
setFocusTraversalPolicyProvider(true);
setFocusTraversalPolicy(new MyTraversalPolicy(tp));
pack();
setVisible(true);
}
private class MyTraversalPolicy extends FocusTraversalPolicy {
ArrayList<Component> components = null;
public MyTraversalPolicy(ArrayList<Component> list){
this.components = list;
}
public Component getComponentAfter(Container focusCycleRoot, Component focused){
int idx = components.indexOf(focused);
if (idx >= components.size()-1){
idx = -1;
}
return components.get(idx+1);
}
public Component getComponentBefore(Container c, Component focused){
int idx = components.indexOf(focused);
if (idx <= 0){
idx = components.size();
}
return components.get(idx-1);
}
public Component getDefaultComponent(Container c){
return f2;
}
public Component getFirstComponent(Container c){
return components.get(0);
}
//Set the field of focus
//when the window is first activated/contructed/visible
public Component getInitialComponent(Window w){
return f2;
}
public Component getLastComponent(Container c){
return components.get(components.size()-1);
}
}
private class ButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e){
if (e.getSource() == b1){
f1.setText("button 1 is pressed");
f2.setText("");
} else if (e.getSource() == b2){
f2.setText("button 2 is pressed");
f1.setText("");
}
}
}
public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new FocusTest();
}
}
);
}
}
Previous <
Reference:
1. Set focus to a field in java swing when a window/JFrame is first activated/constructed (1): requestFocusInWindow--------------------------------------------------------------------------------------------------------------
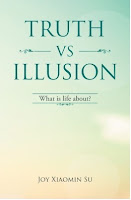
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment