If you don't have the javax.mail package in your JDK, you can download it from its official website
Following is an example of sending a simple text email to recipients
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class SimpleEmailDemo {
//an example of username: "john.smith@gmail.com"
private final String username = "<username>@<mail host>";
private final String password = "<your password>";
private Properties properties = null;
private Session session = null;
public SimpleEmailDemo() {
properties = new Properties();
//the smtp host at gmail is "smtp.gmail.com"
properties.put("mail.smtp.host", "<the smtp host>");
//the smtp port at gmail is "587"
properties.put("mail.smtp.port", "<port>");
properties.put("mail.smtp.auth", "true");
properties.put("mail.smtp.starttls.enable", "true");
session = Session.getInstance(properties,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
}
public void sendEmail(String recipients, String subject, String content, String messageType)
throws MessagingException {
MimeMessage message = new MimeMessage(session);
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(recipients));
// message.setRecipients(Message.RecipientType.BCC, InternetAddress.parse(recipients));
// message.setRecipients(Message.RecipientType.CC, InternetAddress.parse(recipients));
message.setSubject(subject);
if (messageType.equalsIgnoreCase("text/html")) {
message.setContent(content, messageType);
} else {
message.setText(content);
}
Transport.send(message);
}
public static void main(String[] args) {
String recipients = "justLucky@gmail.com, justLucky@yahoo.com";
String subject = "Testing";
String content = "I am happy that you are reading this";
String htmlContent = "<html><h1>I am happy that you are reading this</h1></html>";
SimpleEmailDemo mailSender = new SimpleEmailDemo();
try {
mailSender.sendEmail(recipients, subject, content, null);
mailSender.sendEmail(recipients, subject, htmlContent, "text/html");
} catch (MessagingException e) {
System.out.println(e.getMessage());
}
}
}
> Next
2. Java - Sending Email
3. JavaMail API – Sending Email Via Gmail SMTP Example
-------------------------------------------------------------------------------------------------------------------
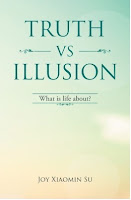
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Following is an example of sending a simple text email to recipients
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class SimpleEmailDemo {
//an example of username: "john.smith@gmail.com"
private final String username = "<username>@<mail host>";
private final String password = "<your password>";
private Properties properties = null;
private Session session = null;
public SimpleEmailDemo() {
properties = new Properties();
//the smtp host at gmail is "smtp.gmail.com"
properties.put("mail.smtp.host", "<the smtp host>");
//the smtp port at gmail is "587"
properties.put("mail.smtp.port", "<port>");
properties.put("mail.smtp.auth", "true");
properties.put("mail.smtp.starttls.enable", "true");
session = Session.getInstance(properties,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
}
public void sendEmail(String recipients, String subject, String content, String messageType)
throws MessagingException {
MimeMessage message = new MimeMessage(session);
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(recipients));
// message.setRecipients(Message.RecipientType.BCC, InternetAddress.parse(recipients));
// message.setRecipients(Message.RecipientType.CC, InternetAddress.parse(recipients));
message.setSubject(subject);
if (messageType.equalsIgnoreCase("text/html")) {
message.setContent(content, messageType);
} else {
message.setText(content);
}
Transport.send(message);
}
public static void main(String[] args) {
String recipients = "justLucky@gmail.com, justLucky@yahoo.com";
String subject = "Testing";
String content = "I am happy that you are reading this";
String htmlContent = "<html><h1>I am happy that you are reading this</h1></html>";
SimpleEmailDemo mailSender = new SimpleEmailDemo();
try {
mailSender.sendEmail(recipients, subject, content, null);
mailSender.sendEmail(recipients, subject, htmlContent, "text/html");
} catch (MessagingException e) {
System.out.println(e.getMessage());
}
}
}
> Next
References:
1. Email in Java - the JavaMail API (2): Multipart and attachment2. Java - Sending Email
3. JavaMail API – Sending Email Via Gmail SMTP Example
-------------------------------------------------------------------------------------------------------------------
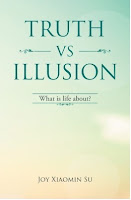
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment