To construct a complex email body, the javax.mail.Multipart and the javax.mail.internet.MimeBodyPart are used to set the content of the message. Each part can be set to independent style and content. The first part (part 0) is the main body of the email, all the other parts are attachments to the email.
Following is a sample code of constructing a complex email.
import java.util.Properties;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Multipart;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
public class JavaMailDemo {
//an example of username: "john.smith@gmail.com"
private final String username = "<username>@<email host>";
private final String password = "<your password>";
private Properties properties = null;
private Session session = null;
public JavaMailDemo() {
properties = new Properties();
//the smtp host at gmail is "smtp.gmail.com"
properties.put("mail.smtp.host", "<the smtp email host>");
//the smtp port at gmail is "587"
properties.put("mail.smtp.port", "<port>");
properties.put("mail.smtp.auth", "true");
properties.put("mail.smtp.starttls.enable", "true");
session = Session.getInstance(properties,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
}
public void sendEmail(String recipients, String subject, String content,
String messageType, String filePath)
throws MessagingException {
MimeMessage message = new MimeMessage(session);
Multipart theMail = new MimeMultipart();
//Add the text content
MimeBodyPart textContent = new MimeBodyPart();
textContent.setContent(content, "text/plain");
theMail.addBodyPart(textContent, 0);
//Add the html content
MimeBodyPart htmlContent = new MimeBodyPart();
htmlContent.setContent("<html><h1>"+content+"</h1></html>", "text/html");
theMail.addBodyPart(htmlContent, 1);
//Add the attachment
FileDataSource theFile = new FileDataSource(filePath);
MimeBodyPart attachment = new MimeBodyPart();
attachment.setDataHandler(new DataHandler(theFile));
attachment.setFileName(filePath);
theMail.addBodyPart(attachment);
message.setContent(theMail);
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(recipients));
// message.setRecipients(Message.RecipientType.BCC, InternetAddress.parse(recipients));
// message.setRecipients(Message.RecipientType.CC, InternetAddress.parse(recipients));
message.setSubject(subject);
Transport.send(message);
}
public static void main(String[] args) {
String recipients = "sarah.campbell@gmail.com, sarah.campbell@yahoo.com";
String subject = "Testing again";
String content = "I am happy that you are reading this";
JavaMailDemo mailSender = new JavaMailDemo();
try {
mailSender.sendEmail(recipients, subject, content, "text/html",
"<path>/<file name>");
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
Previous <
2. Internet media type
---------------------------------------------------------------------------------------------------------------------
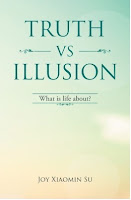
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Following is a sample code of constructing a complex email.
import java.util.Properties;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Multipart;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
public class JavaMailDemo {
//an example of username: "john.smith@gmail.com"
private final String username = "<username>@<email host>";
private final String password = "<your password>";
private Properties properties = null;
private Session session = null;
public JavaMailDemo() {
properties = new Properties();
//the smtp host at gmail is "smtp.gmail.com"
properties.put("mail.smtp.host", "<the smtp email host>");
//the smtp port at gmail is "587"
properties.put("mail.smtp.port", "<port>");
properties.put("mail.smtp.auth", "true");
properties.put("mail.smtp.starttls.enable", "true");
session = Session.getInstance(properties,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
}
public void sendEmail(String recipients, String subject, String content,
String messageType, String filePath)
throws MessagingException {
MimeMessage message = new MimeMessage(session);
Multipart theMail = new MimeMultipart();
//Add the text content
MimeBodyPart textContent = new MimeBodyPart();
textContent.setContent(content, "text/plain");
theMail.addBodyPart(textContent, 0);
//Add the html content
MimeBodyPart htmlContent = new MimeBodyPart();
htmlContent.setContent("<html><h1>"+content+"</h1></html>", "text/html");
theMail.addBodyPart(htmlContent, 1);
//Add the attachment
FileDataSource theFile = new FileDataSource(filePath);
MimeBodyPart attachment = new MimeBodyPart();
attachment.setDataHandler(new DataHandler(theFile));
attachment.setFileName(filePath);
theMail.addBodyPart(attachment);
message.setContent(theMail);
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(recipients));
// message.setRecipients(Message.RecipientType.BCC, InternetAddress.parse(recipients));
// message.setRecipients(Message.RecipientType.CC, InternetAddress.parse(recipients));
message.setSubject(subject);
Transport.send(message);
}
public static void main(String[] args) {
String recipients = "sarah.campbell@gmail.com, sarah.campbell@yahoo.com";
String subject = "Testing again";
String content = "I am happy that you are reading this";
JavaMailDemo mailSender = new JavaMailDemo();
try {
mailSender.sendEmail(recipients, subject, content, "text/html",
"<path>/<file name>");
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
Previous <
References:
1.Email in Java - the JavaMail API (1): A simple text or html email2. Internet media type
---------------------------------------------------------------------------------------------------------------------
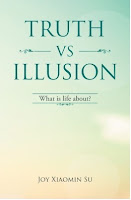
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment