The OutOfMemoryError is thrown when there is not enough memory available for the Java Virtual Machine to allocate an Object. Several situations can cause it to happen such as infinite loop in your program, the size of the heap space is set too small, or the program is too big to run on your machine.
public class MemoryTest {
public static void main(String[] args) {
int j = 0;
for (int i=0; i<10; j++) {
System.out.println("Finished item: "+j);
j++;
}
}
}
The typo "j++" in the for loop will let the program to run continuously until it runs out of the memory. Replacing the j++ with i++ fixes the problem.
A. Run program from command line
To run your MemoryTest program from command line, yo can type
java -Xmx1024M MemoryTest
or
java -Xms512M -Xmx1G MemoryTest
Many of the IDEs for java development have places for setting these configurations.
B. NetBeans
a). Click "Run" in the top menu, choose "Set Project Configuration", then choose "Customize". Or right click a project, choose "Set Configuration", then choose "Customize".
b). In the pop up window, select "Run" in the left Categories, then set your memory size in the "VM Options" on the right side of the window.
C. Eclipse
a). Click "Run" on the menu, choose "Run Configurations"
b). In the pop up window, choose the application from Java Application on the left side of the window.
c). On the right side of the window, click on the Arguments tab, and enter the -Xms<size> -Xmx<size> under the VM arguments.
If you are a NetBeans IDE user, you may use the IDE's profiler to find the code that has memory leak.
----------------------------------------------------------------------------------------------------------
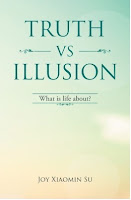
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
1. Code has infinite loop.
The following code will throw the OutOfMemoryErrorpublic class MemoryTest {
public static void main(String[] args) {
int j = 0;
for (int i=0; i<10; j++) {
System.out.println("Finished item: "+j);
j++;
}
}
}
The typo "j++" in the for loop will let the program to run continuously until it runs out of the memory. Replacing the j++ with i++ fixes the problem.
2. Heap space is too small for running a program
This can be fixed by allocating more memory for the heap space through changing the Java Virtual Machine arguments: -Xms<size> (the initial memory size) and -Xmx<size> (the maximum memory size).A. Run program from command line
To run your MemoryTest program from command line, yo can type
java -Xmx1024M MemoryTest
or
java -Xms512M -Xmx1G MemoryTest
Many of the IDEs for java development have places for setting these configurations.
B. NetBeans
a). Click "Run" in the top menu, choose "Set Project Configuration", then choose "Customize". Or right click a project, choose "Set Configuration", then choose "Customize".
b). In the pop up window, select "Run" in the left Categories, then set your memory size in the "VM Options" on the right side of the window.
C. Eclipse
a). Click "Run" on the menu, choose "Run Configurations"
b). In the pop up window, choose the application from Java Application on the left side of the window.
c). On the right side of the window, click on the Arguments tab, and enter the -Xms<size> -Xmx<size> under the VM arguments.
3. Memory leaks
Exam your code carefully to ensure that all references to objects are set to null as soon as the objects are not needed any more. For more information on memory leaks, you can read this article from Oracle and the article from IBM. There are also many memory leak detection software available online.If you are a NetBeans IDE user, you may use the IDE's profiler to find the code that has memory leak.
4. The program is too big to run on the machine
You need to upgrade your computer to add more memory to it.----------------------------------------------------------------------------------------------------------
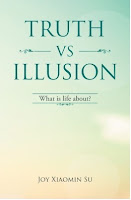
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment