TCP: Transmission Control Protocol, is a connection-based protocol which guarantees that data reaches its destination successfully and in the same order the data was sent.
UDP: User Datagram Protocol, sends packets of data, datagrams, from one application to another, without guarantee that the data will arrive their destination, and the datagrams may reach their destination in any order. UDP is not connection-based.
Java classes which use TCP or UDP to communicate over network are located in the java.net package. Classes such as URL, URLConnection, Socket, and ServerSocket use the TCP; and classes such as DatagramPacket, DatagramSocket, and MulticastSocket use the UDP.
import java.io.*;
public class TCPWorker {
public static void main(String[] args) throws Exception {
//Read the www.google.com web site line by line
URL gUrl = new URL("http://www.google.com/");
BufferedReader in = new BufferedReader(
new InputStreamReader(gUrl.openStream()));
//Or use URLConnection to read from the URL
// URLConnection guCon = gUrl.openConnection();
// BufferedReader in = new BufferedReader (
// new InputStreamReader(
// guCon.getInputStream()));
String inputLine;
while ((inputLine = in.readLine()) != null)
System.out.println(inputLine);
in.close();
}
//Save the content of www.google.com web site to a file
//URL gUrl = new URL("http://www.google.com/");
InputStream is = gUrl.openStream();
Files.copy(is, FileSystems.getDefault().getPath("<dirctory>", "<file name>"), StandardCopyOption.REPLACE_EXISTING);
}
import java.net.*;
import java.io.*;
public class TCPServer {
public static void main(String[] args) {
int connectionCount = 0;
try {
ServerSocket serverSocket = new ServerSocket(<port number>);
while (connectionCount < 20) {
Socket clientSocket = serverSocket.accept();
connectionCount++;
new Thread() {
public void run() {
BufferedReader reader = new BufferedReader(
new InputStreamReader(
clientSocket.getInputStream()));
PrintWriter writer = new PrintWriter(
clientSocket.getOutputStream());
String request = "";
while ((request = reader.readLine()) != null) {
writer.println("Keep searching, you will get the answer eventually!");
}
public void join() {
}.start();
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
//The client
import java.net.*;
import java.io.*;
public class TCPClient {
public static void main(String[] args) {
try {
Socket clientSocket = new Socket(<host name>, <port number>);
PrintWriter writer = new PrintWriter(
clientSocket.getOutputStream(), true);
BufferedReader reader = new BufferedReader(
new InputStreamReader(
clientSocket.getInputStream()));
writer.println("What is the status?");
String answer = "";
while ((answer = reader.readLine()) != null) {
if (answer.length() > 0) {
writer.println("Got it, thanks");
System.out.println("The answer is: "+answer);
}
writer.println("What is the status now?");
}
//Send the content of a file to the server
OutputStream os = clientSocket.getOutputStream();
Files.copy(FileSystems.getDefault().getPath("<dirctory>", "<file name>"), os);
}
}
}
import java.net.*;
import java.io.*;
public class UDPServer extends Thread {
private boolean keepRunning = true;
public void run() {
//create the socket
DatagramSocket serverSocket = new DatagramSocket(<port number>);
while (keepRunning) {
try {
//receive data
byte[] inData = new byte[serverSocket.getReceiveBufferSize()];
DatagramPacket packet = new DatagramPacket(inData, inData.length);
serverSocket.receive(packet);
byte[] dataReceived = packet.getData();
System.out.println("The request is: "+new String(dataReceived, 0, serverSocket.getLength()));
//send data
SocketAddress address = packet.getSocketAddress();
String answer = "Keep searching, you will get the answer eventually";
byte[] outData = answer.getBytes();
packet = new DatagramPacket(outData, outData.length, address);
serverSocket.send(packet);
} catch (Exception e) {
e.printStackTrace();
keepRunning = false;
}
serverSocket.close();
}
public static void main(String[] args) {
new UDPServer().start();
}
}
//The client
import java.net.*;
import java.io.*;
public class UDPClient {
public static void main(String[] args) throws Exception {
//create the socket
DatagramSocket clientSocket = new DatagramSocket();
//send request
String request = "What is the status?";
byte[] outData = request.getBytes();
SocketAddress serverAddress = new InetSocketAddress(<host name>, <port number>);
DatagramPacket packet = new DatagramPacket(outData, outData.length, serverAddress);
clientSocket.send(packet);
//receive response
byte[] inData = new byte[serverSocket.getReceiveBufferSize()];
packet = new DatagramPacket(inData, inData.length);
clientSocket.receive(packet);
byte[] dataReceived = packet.getData();
System.out.println("The response is: "+new String(dataReceived, 0, clientSocket.getLength()));
}
}
2. Writing a datagram client and server
-----------------------------------------------------------------------------------------------------------------
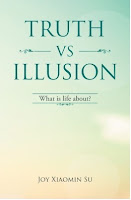
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
UDP: User Datagram Protocol, sends packets of data, datagrams, from one application to another, without guarantee that the data will arrive their destination, and the datagrams may reach their destination in any order. UDP is not connection-based.
Java classes which use TCP or UDP to communicate over network are located in the java.net package. Classes such as URL, URLConnection, Socket, and ServerSocket use the TCP; and classes such as DatagramPacket, DatagramSocket, and MulticastSocket use the UDP.
A. Sample code using TCP to communicate over the Internet
1. Through URL and URLConnection
import java.net.*;import java.io.*;
public class TCPWorker {
public static void main(String[] args) throws Exception {
//Read the www.google.com web site line by line
URL gUrl = new URL("http://www.google.com/");
BufferedReader in = new BufferedReader(
new InputStreamReader(gUrl.openStream()));
//Or use URLConnection to read from the URL
// URLConnection guCon = gUrl.openConnection();
// BufferedReader in = new BufferedReader (
// new InputStreamReader(
// guCon.getInputStream()));
String inputLine;
while ((inputLine = in.readLine()) != null)
System.out.println(inputLine);
in.close();
}
//Save the content of www.google.com web site to a file
//URL gUrl = new URL("http://www.google.com/");
InputStream is = gUrl.openStream();
Files.copy(is, FileSystems.getDefault().getPath("<dirctory>", "<file name>"), StandardCopyOption.REPLACE_EXISTING);
}
2. Through Socket
//The serverimport java.net.*;
import java.io.*;
public class TCPServer {
public static void main(String[] args) {
int connectionCount = 0;
try {
ServerSocket serverSocket = new ServerSocket(<port number>);
while (connectionCount < 20) {
Socket clientSocket = serverSocket.accept();
connectionCount++;
new Thread() {
public void run() {
BufferedReader reader = new BufferedReader(
new InputStreamReader(
clientSocket.getInputStream()));
PrintWriter writer = new PrintWriter(
clientSocket.getOutputStream());
String request = "";
while ((request = reader.readLine()) != null) {
writer.println("Keep searching, you will get the answer eventually!");
}
public void join() {
}.start();
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
//The client
import java.net.*;
import java.io.*;
public class TCPClient {
public static void main(String[] args) {
try {
Socket clientSocket = new Socket(<host name>, <port number>);
PrintWriter writer = new PrintWriter(
clientSocket.getOutputStream(), true);
BufferedReader reader = new BufferedReader(
new InputStreamReader(
clientSocket.getInputStream()));
writer.println("What is the status?");
String answer = "";
while ((answer = reader.readLine()) != null) {
if (answer.length() > 0) {
writer.println("Got it, thanks");
System.out.println("The answer is: "+answer);
}
writer.println("What is the status now?");
}
//Send the content of a file to the server
OutputStream os = clientSocket.getOutputStream();
Files.copy(FileSystems.getDefault().getPath("<dirctory>", "<file name>"), os);
}
}
}
B. Sample code using UDP to communicate over the network
//The serverimport java.net.*;
import java.io.*;
public class UDPServer extends Thread {
private boolean keepRunning = true;
public void run() {
//create the socket
DatagramSocket serverSocket = new DatagramSocket(<port number>);
while (keepRunning) {
try {
//receive data
byte[] inData = new byte[serverSocket.getReceiveBufferSize()];
DatagramPacket packet = new DatagramPacket(inData, inData.length);
serverSocket.receive(packet);
byte[] dataReceived = packet.getData();
System.out.println("The request is: "+new String(dataReceived, 0, serverSocket.getLength()));
//send data
SocketAddress address = packet.getSocketAddress();
String answer = "Keep searching, you will get the answer eventually";
byte[] outData = answer.getBytes();
packet = new DatagramPacket(outData, outData.length, address);
serverSocket.send(packet);
} catch (Exception e) {
e.printStackTrace();
keepRunning = false;
}
serverSocket.close();
}
public static void main(String[] args) {
new UDPServer().start();
}
}
//The client
import java.net.*;
import java.io.*;
public class UDPClient {
public static void main(String[] args) throws Exception {
//create the socket
DatagramSocket clientSocket = new DatagramSocket();
//send request
String request = "What is the status?";
byte[] outData = request.getBytes();
SocketAddress serverAddress = new InetSocketAddress(<host name>, <port number>);
DatagramPacket packet = new DatagramPacket(outData, outData.length, serverAddress);
clientSocket.send(packet);
//receive response
byte[] inData = new byte[serverSocket.getReceiveBufferSize()];
packet = new DatagramPacket(inData, inData.length);
clientSocket.receive(packet);
byte[] dataReceived = packet.getData();
System.out.println("The response is: "+new String(dataReceived, 0, clientSocket.getLength()));
}
}
References:
1. Reading from and writing to a URLConnection2. Writing a datagram client and server
-----------------------------------------------------------------------------------------------------------------
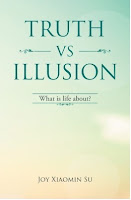
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment