Functional Interface is a new concept introduced in java 8. Functional interfaces are particularly used for Lambda Expressions. Following are the characters of function interfaces.
1. A functional interface can have only one abstract method except those methods declared in the Object class. For example the Runable interface has only one method run(), therefore it is a functional interface. Following is another example.
public interface Qualified {
public Object getTheObject();
public String toString();
public boolean equals(Object obj);
}
Since the toString and equals methods are methods declared in the Object class, this Qualified interface is also a functional interface.
2. A functional interface can have static and default methods.
public interface GetIt {
public Object getIt();
public static boolean checkIt(Object obj) {
if (obj instanceof String) return true;
return false;
}
public default Integer getSum(Integer one, Integer two) {
return one+two;
}
}
The GetIt interface has only one abstract method, getIt, so it is a functional interface.
3. The abstract method of a functional interface can be inherited from another interface or overrides a method in another interface.
//interface Face1 has two abstract methods
interface Face1 {
public boolean isTrue();
public void changeIt();
}
//interface Face2 has one abstract method, changeIt, inherited from face1
//therefore, interface Face2 is a functional interface
interface Face2 extends Face1 {
@Override
default boolean isTrue() {return true;}
}
//interface Face3 has one abstract method that overrides the method in its super interface
//therefore, interface Face3 is a functional interface
interface Face3 extends Face2 {
@Override
public void changeIt();
}
//Since Face2 and Face3 are functional interfaces, they can be implemented with Lambda Expression
public class TestDefautMethod {
public void testDefault(Face2 theFace2, Face3 theFace3){
theFace2.changeIt();
theFace3.changeIt();
}
public static void main(String[] args){
TestDefautMethod tester = new TestDefautMethod();
tester.testDefault(()->System.out.println("Changed it successfully in Face2!"),
()->System.out.println("Changed it successfully in Face3!"));
}
}
The output of executing TestDefautMethod is the following.
Changed it successfully in Face2!
Changed it successfully in Face3!
4. In java 8, a functional interface is normally marked with the annotation @FunctionalInterface. However, as long as an interface has only one abstract method, it is a qualified functional interface regardless whether it has the annotation or not.
However, if an interface has the @FunctionalInterface annotation and contains more than one abstract method, the compiler will identify it as an error.
@FunctionalInterface
interface Face4 extends Face3 {
public Integer sumIt(Integer one, Integer two);
}
Face4 has two abstract methods, changeIt inherited from super interface and sumIt declared in itself. Compiling this code generates the following error.
error: Unexpected @FunctionalInterface annotation
@FunctionalInterface
Face4 is not a functional interface
multiple non-overriding abstract methods found in interface Face4
-----------------------------------------------------------------------------------------------------------------
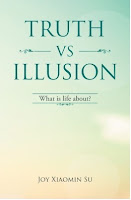
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
1. A functional interface can have only one abstract method except those methods declared in the Object class. For example the Runable interface has only one method run(), therefore it is a functional interface. Following is another example.
public interface Qualified {
public Object getTheObject();
public String toString();
public boolean equals(Object obj);
}
Since the toString and equals methods are methods declared in the Object class, this Qualified interface is also a functional interface.
2. A functional interface can have static and default methods.
public interface GetIt {
public Object getIt();
public static boolean checkIt(Object obj) {
if (obj instanceof String) return true;
return false;
}
public default Integer getSum(Integer one, Integer two) {
return one+two;
}
}
The GetIt interface has only one abstract method, getIt, so it is a functional interface.
3. The abstract method of a functional interface can be inherited from another interface or overrides a method in another interface.
//interface Face1 has two abstract methods
interface Face1 {
public boolean isTrue();
public void changeIt();
}
//interface Face2 has one abstract method, changeIt, inherited from face1
//therefore, interface Face2 is a functional interface
interface Face2 extends Face1 {
@Override
default boolean isTrue() {return true;}
}
//interface Face3 has one abstract method that overrides the method in its super interface
//therefore, interface Face3 is a functional interface
interface Face3 extends Face2 {
@Override
public void changeIt();
}
//Since Face2 and Face3 are functional interfaces, they can be implemented with Lambda Expression
public class TestDefautMethod {
public void testDefault(Face2 theFace2, Face3 theFace3){
theFace2.changeIt();
theFace3.changeIt();
}
public static void main(String[] args){
TestDefautMethod tester = new TestDefautMethod();
tester.testDefault(()->System.out.println("Changed it successfully in Face2!"),
()->System.out.println("Changed it successfully in Face3!"));
}
}
The output of executing TestDefautMethod is the following.
Changed it successfully in Face2!
Changed it successfully in Face3!
4. In java 8, a functional interface is normally marked with the annotation @FunctionalInterface. However, as long as an interface has only one abstract method, it is a qualified functional interface regardless whether it has the annotation or not.
However, if an interface has the @FunctionalInterface annotation and contains more than one abstract method, the compiler will identify it as an error.
@FunctionalInterface
interface Face4 extends Face3 {
public Integer sumIt(Integer one, Integer two);
}
Face4 has two abstract methods, changeIt inherited from super interface and sumIt declared in itself. Compiling this code generates the following error.
error: Unexpected @FunctionalInterface annotation
@FunctionalInterface
Face4 is not a functional interface
multiple non-overriding abstract methods found in interface Face4
References:
1. Default Methods-----------------------------------------------------------------------------------------------------------------
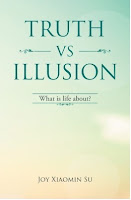
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment