To execute an external shell command in java, you can either call the exec method in java.lang.Runtime or create a ProcessBuilder and call its start method.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import static java.lang.Compiler.command;
public class RunShellCommand {
public static void main(String[] args) {
Process p;
try {
p = Runtime.getRuntime().exec("\"/Program Files/Internet Explorer/iexplore.exe\" http://www.google.com");
p.waitFor();
p.destroy();
} catch (Exception e) {
e.printStackTrace();
}
}
}
2. Get the hostname of your machine
import java.io.BufferedReader;
import java.io.InputStreamReader;
import static java.lang.Compiler.command;
public class RunShellCommand {
public static void main(String[] args) {
Process p;
try {
p = Runtime.getRuntime().exec("hostname");
p.waitFor();
BufferedReader reader = new BufferedReader(
new InputStreamReader(
p.getInputStream()));
StringBuilder result = new StringBuilder();
String line = "";
while ((line = reader.readLine()) != null) {
result.append(line + "\n");
}
System.out.println(result.toString());
p.destroy();
} catch (Exception e) {
e.printStackTrace();
}
}
}
import java.io.InputStreamReader;
public class RunShellCommand {
public static void main(String[] args) {
Process p;
try {
ProcessBuilder pb = new ProcessBuilder("\"/Program Files/Internet Explorer/iexplore.exe\"", "http://www.google.com/search?q=java");
p = pb.start();
p.waitFor();
BufferedReader reader = new BufferedReader(
new InputStreamReader(
p.getInputStream()));
StringBuilder result = new StringBuilder();
String line = "";
while ((line = reader.readLine()) != null) {
result.append(line + "\n");
}
System.out.println(result.toString());
p.destroy();
} catch (Exception e) {
e.printStackTrace();
}
}
}
--------------------------------------------------------------------------------------------------------------
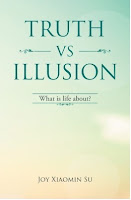
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Call the exec method in java.lang.Runtime
1. Launch the internet explorerimport java.io.BufferedReader;
import java.io.InputStreamReader;
import static java.lang.Compiler.command;
public class RunShellCommand {
public static void main(String[] args) {
Process p;
try {
p = Runtime.getRuntime().exec("\"/Program Files/Internet Explorer/iexplore.exe\" http://www.google.com");
p.waitFor();
p.destroy();
} catch (Exception e) {
e.printStackTrace();
}
}
}
2. Get the hostname of your machine
import java.io.BufferedReader;
import java.io.InputStreamReader;
import static java.lang.Compiler.command;
public class RunShellCommand {
public static void main(String[] args) {
Process p;
try {
p = Runtime.getRuntime().exec("hostname");
p.waitFor();
BufferedReader reader = new BufferedReader(
new InputStreamReader(
p.getInputStream()));
StringBuilder result = new StringBuilder();
String line = "";
while ((line = reader.readLine()) != null) {
result.append(line + "\n");
}
System.out.println(result.toString());
p.destroy();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Create a ProcessBuilder and call its start method
import java.io.BufferedReader;import java.io.InputStreamReader;
public class RunShellCommand {
public static void main(String[] args) {
Process p;
try {
ProcessBuilder pb = new ProcessBuilder("\"/Program Files/Internet Explorer/iexplore.exe\"", "http://www.google.com/search?q=java");
p = pb.start();
p.waitFor();
BufferedReader reader = new BufferedReader(
new InputStreamReader(
p.getInputStream()));
StringBuilder result = new StringBuilder();
String line = "";
while ((line = reader.readLine()) != null) {
result.append(line + "\n");
}
System.out.println(result.toString());
p.destroy();
} catch (Exception e) {
e.printStackTrace();
}
}
}
--------------------------------------------------------------------------------------------------------------
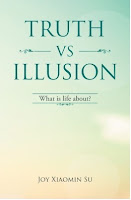
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment