The new Date and Time API in java 8 makes it much easier to create, manipulate, and convert Date related objects. Classes in this new addition such as LocalDate, LocalDateTime, and LocalDateTimeFormatter are very convenient to use.
LocalDate date = LocalDate.now();
b). Current date with hour, minute, and second
LocalDateTime dateTime = LocalDateTime.now();
c). A date with given year, month and dayOfMonth
int year = 2013;
int month = 11;
int dayOfMonth = 22;
LocalDate date2 = LocalDate.of (year, month, dayOfMonth);
d). A date with given year, month, dayOfMonth, hour, minute, second, and nanosecond
int year = 2013;
int month = 11;
int dayOfMonth = 22;
int hour = 21, minute = 15, second = 50;
LocalDateTime dateTime2 = LocalDateTime.of (year, month, dayOfMonth, hour, minute, second);
To find next Saturday,
LocalDate nextSaturday = date.with(TemporalAdjusters.next(DayOfWeek.SATURDAY));
DateTimeFormatter pattern = DateTimeFormatter.ofPattern("<pattern of your date string>"); //e.g "MM/dd/yyyy"
LocalDate date = LocalDate.parse(dateString, pattern);
b). String dateTimeString = "<your dateTime>"; //e.g "11/22/2013 21:15:50"
DateTimeFormatter pattern = DateTimeFormatter.ofPattern("<pattern of your date string>"); //e.g "MM/dd/yyyy HH:mm:ss"
LocalDateTime dateTime = LocalDateTime.parse(dateTimeString, pattern);
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("MM/dd/yyyy HH:mm:ss");
String formattedDate = formatter.format(dateTime2);
b). Converting to "MM/dd/yyyy hh:mm:ss aa" format, e.g "11/22/2013 09:15:50 PM"
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("MM/dd/yyyy hh:mm:ss aa");
String formattedDate = formatter.format(dateTime2);
References:
1. Convert Date format
2. Oracle: LocalDate
---------------------------------------------------------------------------------------------------------------
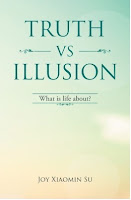
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
1. Creating a date
a). Current date without hour, minute, and secondLocalDate date = LocalDate.now();
b). Current date with hour, minute, and second
LocalDateTime dateTime = LocalDateTime.now();
c). A date with given year, month and dayOfMonth
int year = 2013;
int month = 11;
int dayOfMonth = 22;
LocalDate date2 = LocalDate.of (year, month, dayOfMonth);
d). A date with given year, month, dayOfMonth, hour, minute, second, and nanosecond
int year = 2013;
int month = 11;
int dayOfMonth = 22;
int hour = 21, minute = 15, second = 50;
LocalDateTime dateTime2 = LocalDateTime.of (year, month, dayOfMonth, hour, minute, second);
2. Finding a date
LocalDate date = <your date>;To find next Saturday,
LocalDate nextSaturday = date.with(TemporalAdjusters.next(DayOfWeek.SATURDAY));
3. Converting a String to a date
a). String dateString = "<your date>"; //e.g "11/22/2013"DateTimeFormatter pattern = DateTimeFormatter.ofPattern("<pattern of your date string>"); //e.g "MM/dd/yyyy"
LocalDate date = LocalDate.parse(dateString, pattern);
b). String dateTimeString = "<your dateTime>"; //e.g "11/22/2013 21:15:50"
DateTimeFormatter pattern = DateTimeFormatter.ofPattern("<pattern of your date string>"); //e.g "MM/dd/yyyy HH:mm:ss"
LocalDateTime dateTime = LocalDateTime.parse(dateTimeString, pattern);
4. Converting date Format
a). Converting to "MM/dd/yyyy HH:mm:ss" format, e.g "11/22/2013 21:15:50"DateTimeFormatter formatter = DateTimeFormatter.ofPattern("MM/dd/yyyy HH:mm:ss");
String formattedDate = formatter.format(dateTime2);
b). Converting to "MM/dd/yyyy hh:mm:ss aa" format, e.g "11/22/2013 09:15:50 PM"
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("MM/dd/yyyy hh:mm:ss aa");
String formattedDate = formatter.format(dateTime2);
References:
1. Convert Date format
2. Oracle: LocalDate
---------------------------------------------------------------------------------------------------------------
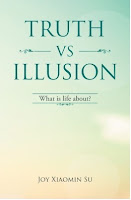
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment