Java DB is a full-featured, easy-to-use, open source database which is the Apache Derby open source database included in the java JDK starting version 5.
DBMS or Vendor: derby
Driver: org.apache.derby.jdbc.EmbeddedDriver
URL: when it is used before the database is created, the URL is jdbc:derby:<database name>;create=true, afterwards, the URL is simply jdbc:derby:<database name>
You need to include the jars in the <java home>\db\lib directory in your project classpath for using the database.
Following is a sample code of using the Java DB.
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class JavaDBTest {
private Connection conn = null;
private boolean databaseCreated = false;
private void createDatabase()
throws ClassNotFoundException, SQLException {
Class.forName("org.apache.derby.jdbc.EmbeddedDriver");
conn = DriverManager.getConnection(
"jdbc:derby:testDB;create=true");
databaseCreated = true;
}
public Connection getConnection()
throws ClassNotFoundException, SQLException{
if (conn != null) return conn;
if (databaseCreated) {
Class.forName("org.apache.derby.jdbc.EmbeddedDriver");
conn = DriverManager.getConnection(
"jdbc:derby:testDB");
} else {
createDatabase();
}
return conn;
}
public static void main(String[] args){
JavaDBTest test = new JavaDBTest();
try {
Connection connection = test.getConnection();
DatabaseMetaData dmd = connection.getMetaData();
System.out.println(dmd.getDriverName());
Statement statement = connection.createStatement();
statement.execute("create table fruit (name varchar(30), value varchar(20))");
statement.executeUpdate("insert into fruit values ('Apple', '100')");
ResultSet result = statement.executeQuery("select * from fruit");
while(result.next()){
System.out.println("name="+result.getString(1));
System.out.println("value="+result.getString(2));
}
result.close();
statement.close();
connection.close();
}catch(Exception e){
e.printStackTrace();
}
}
}
References:
1.Java DB
2. Working with Java DB in NetBeans
-----------------------------------------------------------------------------------------------------------------
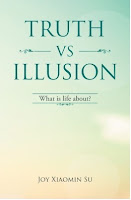
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
DBMS or Vendor: derby
Driver: org.apache.derby.jdbc.EmbeddedDriver
URL: when it is used before the database is created, the URL is jdbc:derby:<database name>;create=true, afterwards, the URL is simply jdbc:derby:<database name>
You need to include the jars in the <java home>\db\lib directory in your project classpath for using the database.
Following is a sample code of using the Java DB.
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class JavaDBTest {
private Connection conn = null;
private boolean databaseCreated = false;
private void createDatabase()
throws ClassNotFoundException, SQLException {
Class.forName("org.apache.derby.jdbc.EmbeddedDriver");
conn = DriverManager.getConnection(
"jdbc:derby:testDB;create=true");
databaseCreated = true;
}
public Connection getConnection()
throws ClassNotFoundException, SQLException{
if (conn != null) return conn;
if (databaseCreated) {
Class.forName("org.apache.derby.jdbc.EmbeddedDriver");
conn = DriverManager.getConnection(
"jdbc:derby:testDB");
} else {
createDatabase();
}
return conn;
}
public static void main(String[] args){
JavaDBTest test = new JavaDBTest();
try {
Connection connection = test.getConnection();
DatabaseMetaData dmd = connection.getMetaData();
System.out.println(dmd.getDriverName());
Statement statement = connection.createStatement();
statement.execute("create table fruit (name varchar(30), value varchar(20))");
statement.executeUpdate("insert into fruit values ('Apple', '100')");
ResultSet result = statement.executeQuery("select * from fruit");
while(result.next()){
System.out.println("name="+result.getString(1));
System.out.println("value="+result.getString(2));
}
result.close();
statement.close();
connection.close();
}catch(Exception e){
e.printStackTrace();
}
}
}
References:
1.Java DB
2. Working with Java DB in NetBeans
-----------------------------------------------------------------------------------------------------------------
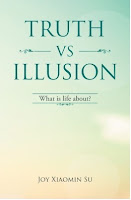
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment