If in your JTable, one column stores the value that is the result of certain calculation of the values in other columns, you can let the value of the column to be automatically updated when values in other columns are modified. This can be achieved by using the TableModelListener to monitor changes in the other columns. The following code is an example of how it is done.
import java.awt.Component;
import javax.swing.DefaultCellEditor;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.event.TableModelEvent;
import javax.swing.event.TableModelListener;
import javax.swing.table.DefaultTableCellRenderer;
import javax.swing.table.DefaultTableModel;
public class TableCalculationTest extends JFrame {
JTable table = null;
DefaultTableModel model = null;
TableModelListener listener = null;
public TableCalculationTest() {
table = new JTable();
model = new TableModel();
model.addRow(new Object[] {"Apple", new Float(3.99), new Float(80), new Float(2.00), new Float(100), new Float(519.20)});
model.addRow(new Object[] {"Apple", new Float(3.99), new Float(65.5), new Float(2.50), new Float(300), new Float(1011.35)});
model.addRow(new Object[] {"Avacado", new Float(4.99), new Float(15), new Float(3.50), new Float(200), new Float(774.85)});
table.setModel(model);
table.getTableHeader().setReorderingAllowed(false);
table.setDefaultRenderer(Float.class, new FloatRenderer());
table.setDefaultEditor(Float.class, new FloatCellEditor(new JTextField()));
listener = new TableChangeListener();
model.addTableModelListener(listener);
JScrollPane jsp = new JScrollPane(table);
getContentPane().add(jsp);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setVisible(true);
}
private class TableChangeListener implements TableModelListener {
@Override
public void tableChanged(TableModelEvent e){
int row = e.getFirstRow();
int col = e.getColumn();
//remove the TableModelListener to avoid infinite looping
model.removeTableModelListener(listener);
if (col != 0){
//do the calculation
Float rowTotal = (Float)model.getValueAt(row, 1) * (Float)model.getValueAt(row, 2) +
(Float)model.getValueAt(row, 3) * (Float)model.getValueAt(row, 4);
//set the corresponding field with the calculated value
model.setValueAt(rowTotal, row, 5);
}
//add back the TableModelListener
model.addTableModelListener(listener);
}
}
private class TableModel extends DefaultTableModel{
public TableModel() {
setColumnIdentifiers(new Object[] {"Name", "Detail Price", "Detail Order", "WholeSale Price", "Wholesale Order", "Total Charges"});
}
public Object getColumnCalss(int col){
Object type = Float.class;
if (col == 0){
type = String.class;
}
return type;
}
@Override
public void setValueAt(Object v, int row, int col) {
if (col != 0) {
if (v instanceof String) {
super.setValueAt(Float.parseFloat((String) v), row, col);
} else {
super.setValueAt((Float) v, row, col);
}
}
}
}
private class FloatRenderer extends DefaultTableCellRenderer {
JTextField field = new JTextField();
@Override
public Component getTableCellRendererComponent(JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column){
if (value instanceof Float){
field.setText(String.valueOf((Float)value));
}
return field;
}
}
private class FloatCellEditor extends DefaultCellEditor{
private JTextField field = new JTextField();
public FloatCellEditor(JTextField f) {
super(f);
field = f;
}
@Override
public Component getTableCellEditorComponent(JTable table, Object value, boolean isSelected, int row, int column){
if (value instanceof Float){
JTextField field = new JTextField();
field.setText(String.valueOf((Float)value));
}
return field;
}
}
public static void main(String[] args){
new TableCalculationTest();
}
}
-------------------------------------------------------------------------------------------------------------------
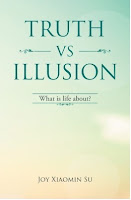
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
import java.awt.Component;
import javax.swing.DefaultCellEditor;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.event.TableModelEvent;
import javax.swing.event.TableModelListener;
import javax.swing.table.DefaultTableCellRenderer;
import javax.swing.table.DefaultTableModel;
public class TableCalculationTest extends JFrame {
JTable table = null;
DefaultTableModel model = null;
TableModelListener listener = null;
public TableCalculationTest() {
table = new JTable();
model = new TableModel();
model.addRow(new Object[] {"Apple", new Float(3.99), new Float(80), new Float(2.00), new Float(100), new Float(519.20)});
model.addRow(new Object[] {"Apple", new Float(3.99), new Float(65.5), new Float(2.50), new Float(300), new Float(1011.35)});
model.addRow(new Object[] {"Avacado", new Float(4.99), new Float(15), new Float(3.50), new Float(200), new Float(774.85)});
table.setModel(model);
table.getTableHeader().setReorderingAllowed(false);
table.setDefaultRenderer(Float.class, new FloatRenderer());
table.setDefaultEditor(Float.class, new FloatCellEditor(new JTextField()));
listener = new TableChangeListener();
model.addTableModelListener(listener);
JScrollPane jsp = new JScrollPane(table);
getContentPane().add(jsp);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setVisible(true);
}
private class TableChangeListener implements TableModelListener {
@Override
public void tableChanged(TableModelEvent e){
int row = e.getFirstRow();
int col = e.getColumn();
//remove the TableModelListener to avoid infinite looping
model.removeTableModelListener(listener);
if (col != 0){
//do the calculation
Float rowTotal = (Float)model.getValueAt(row, 1) * (Float)model.getValueAt(row, 2) +
(Float)model.getValueAt(row, 3) * (Float)model.getValueAt(row, 4);
//set the corresponding field with the calculated value
model.setValueAt(rowTotal, row, 5);
}
//add back the TableModelListener
model.addTableModelListener(listener);
}
}
private class TableModel extends DefaultTableModel{
public TableModel() {
setColumnIdentifiers(new Object[] {"Name", "Detail Price", "Detail Order", "WholeSale Price", "Wholesale Order", "Total Charges"});
}
public Object getColumnCalss(int col){
Object type = Float.class;
if (col == 0){
type = String.class;
}
return type;
}
@Override
public void setValueAt(Object v, int row, int col) {
if (col != 0) {
if (v instanceof String) {
super.setValueAt(Float.parseFloat((String) v), row, col);
} else {
super.setValueAt((Float) v, row, col);
}
}
}
}
private class FloatRenderer extends DefaultTableCellRenderer {
JTextField field = new JTextField();
@Override
public Component getTableCellRendererComponent(JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column){
if (value instanceof Float){
field.setText(String.valueOf((Float)value));
}
return field;
}
}
private class FloatCellEditor extends DefaultCellEditor{
private JTextField field = new JTextField();
public FloatCellEditor(JTextField f) {
super(f);
field = f;
}
@Override
public Component getTableCellEditorComponent(JTable table, Object value, boolean isSelected, int row, int column){
if (value instanceof Float){
JTextField field = new JTextField();
field.setText(String.valueOf((Float)value));
}
return field;
}
}
public static void main(String[] args){
new TableCalculationTest();
}
}
-------------------------------------------------------------------------------------------------------------------
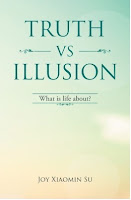
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.