Lets say that you have a FRUIT table containing columns: FRUIT_ID, NAME, PRICE, and POPULARITY. The FRUIT_ID is the primary key
And you have another ORDER table having these columns: ORDER_ID, FRUIT_ID, AMOUNT, and TOTAL_PRICE, The ORDER_ID is the primary key. The FRUIT_ID is the foreign key referencing the FRUIT table.
Now, for some reason, you want the ORDER table to have a FRUIT_NAME column. You can use the following SQL to copy the NAME from the FRUIT table to the ORDER table after you add the FRUIT_NAME column to the ORDER table.
UPDATE ORDER ord
SET FRUIT_NAME = (SELECT frt.NAME
FROM FRUIT frt
WHERE frt.FRUIT_ID = ord.FRUIT_ID)
WHERE FRUIT_NAME is null;
To add the FRUIT_ID column to the ORDER table:
ALTER TABLE ORDER
ADD FRUIT_ID VARCHAR2(9);
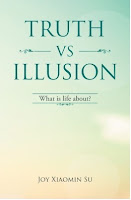
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
And you have another ORDER table having these columns: ORDER_ID, FRUIT_ID, AMOUNT, and TOTAL_PRICE, The ORDER_ID is the primary key. The FRUIT_ID is the foreign key referencing the FRUIT table.
Now, for some reason, you want the ORDER table to have a FRUIT_NAME column. You can use the following SQL to copy the NAME from the FRUIT table to the ORDER table after you add the FRUIT_NAME column to the ORDER table.
UPDATE ORDER ord
SET FRUIT_NAME = (SELECT frt.NAME
FROM FRUIT frt
WHERE frt.FRUIT_ID = ord.FRUIT_ID)
WHERE FRUIT_NAME is null;
To add the FRUIT_ID column to the ORDER table:
ALTER TABLE ORDER
ADD FRUIT_ID VARCHAR2(9);
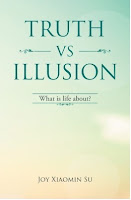
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.