A. Launch the default web browser
1. Launch in Windowsimport java.awt.Desktop;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
public class LaunchBrowserTest {
public static void main(String args[]) {
String url = "http://www.google.com";
//method 1 of launching web browser in Windows
if (Desktop.isDesktopSupported()) {
Desktop desktop = Desktop.getDesktop();
try {
desktop.browse(new URI(url));
} catch (IOException | URISyntaxException e) {
e.printStackTrace();
}
}
//method 2 of launching web browser in Windows
String os = System.getProperty("os.name").toLowerCase();
Runtime rt = Runtime.getRuntime();
if (os.indexOf("win") >= 0) {
try {
rt.exec("rundll32 url.dll,FileProtocolHandler " + url);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
2. Launch in MAC
public class LaunchBrowserTest {
public static void main(String args[]) {
String url = "http://www.google.com";
String os = System.getProperty("os.name").toLowerCase();
Runtime rt = Runtime.getRuntime();
if (os.indexOf("mac") >= 0) {
try {
rt.exec("open " + url);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
3. Launch in other systems
public class LaunchBrowserTest {
public static void main(String args[]) {
String url = "http://www.google.com";
Runtime rt = Runtime.getRuntime();
try {
rt.exec("xdg-open " + url);
} catch (IOException e) {
e.printStackTrace();
}
}
}
B. Launch a particular web browser
1. Launch in Windowspublic class LaunchBrowserTest {
public static void main(String args[]) {
String url = "http://www.google.com";
String os = System.getProperty("os.name").toLowerCase();
Runtime rt = Runtime.getRuntime();
try {
//launch the google chrome
String[] cmds = new String[] {"C:/Program Files (x86)/Google/Chrome/Application/chrome.exe", url};
//launch the internet explorer
// String[] cmds = new String[] {"C:/Program Files (x86)/Internet Explorer/iexplore.exe", url}; //launch the firefox
//String[] cmds = new String[] {"<your path>/firefox.exe", url};
rt.exec(cmds);
} catch (IOException e) {
e.printStackTrace();
}
}
}
2. Launch in Unix/Linux
public class LaunchBrowserTest {
public static void main(String args[]) {
String url = "http://www.google.com";
String os = System.getProperty("os.name").toLowerCase();
Runtime rt = Runtime.getRuntime();
if (os.indexOf("nix") >= 0 || os.indexOf("nux") >= 0) {
try {
//launch the google chrome
rt.exec(new String[] {"chrome", url});
//or
// rt.exec(new String[]{"sh", "-c", "chrome\"+url+"\"});
//launch the firefox
rt.exec(new String[] {"firefox", url});
//or
// rt.exec(new String[]{"sh", "-c", "firefox\"+url+"\"});
} catch (IOException e) {
e.printStackTrace();
}
}
}
------------------------------------------------------------------------------------------------------------------
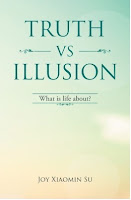
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.