After you upgrade your Oracle database to 12c, you suddenly see this error when you try to connect remotely to your database through JDBC.
java.sql.SQLException: ORA-28040: No matching authentication protocol
at oracle.jdbc.driver.DatabaseError.throwSqlException(DatabaseError.java:112)
at oracle.jdbc.driver.T4CTTIoer.processError(T4CTTIoer.java:331)
at oracle.jdbc.driver.T4CTTIoer.processError(T4CTTIoer.java:283)
at oracle.jdbc.driver.T4CTTIoer.processError(T4CTTIoer.java:278)
at oracle.jdbc.driver.T4CTTIoauthenticate.receiveOsesskey(T4CTTIoauthenticate.java:294)
at oracle.jdbc.driver.T4CConnection.logon(T4CConnection.java:357)
at oracle.jdbc.driver.PhysicalConnection.<init>(PhysicalConnection.java:441)
at oracle.jdbc.driver.T4CConnection.<init>(T4CConnection.java:165)
at oracle.jdbc.driver.T4CDriverExtension.getConnection(T4CDriverExtension.java:35)
at oracle.jdbc.driver.OracleDriver.connect(OracleDriver.java:801)
at java.sql.DriverManager.getConnection(Unknown Source)
at java.sql.DriverManager.getConnection(Unknown Source)
To fix this problem:
Make sure the JDBC driver used by the client is ojdbc7.jar and all other drivers such as ojdbc14.jar are removed.
If the above does not fix this problem, on your Oracle server, open the file $ORACLE_HOME/network/admin/sqlnet.ora and add the line below to it.
SQLNET.ALLOWED_LOGON_VERSION=8
This SQLNET.ALLOWED_LOGON_VERSION parameter specifies the minimum authentication protocol allowed when connecting to Oracle Database instances. The value 8 permits most password versions, and allows any combination of the
-----------------------------------------------------------------------------------------------------------------
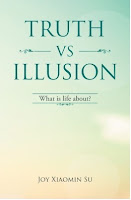
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
java.sql.SQLException: ORA-28040: No matching authentication protocol
at oracle.jdbc.driver.DatabaseError.throwSqlException(DatabaseError.java:112)
at oracle.jdbc.driver.T4CTTIoer.processError(T4CTTIoer.java:331)
at oracle.jdbc.driver.T4CTTIoer.processError(T4CTTIoer.java:283)
at oracle.jdbc.driver.T4CTTIoer.processError(T4CTTIoer.java:278)
at oracle.jdbc.driver.T4CTTIoauthenticate.receiveOsesskey(T4CTTIoauthenticate.java:294)
at oracle.jdbc.driver.T4CConnection.logon(T4CConnection.java:357)
at oracle.jdbc.driver.PhysicalConnection.<init>(PhysicalConnection.java:441)
at oracle.jdbc.driver.T4CConnection.<init>(T4CConnection.java:165)
at oracle.jdbc.driver.T4CDriverExtension.getConnection(T4CDriverExtension.java:35)
at oracle.jdbc.driver.OracleDriver.connect(OracleDriver.java:801)
at java.sql.DriverManager.getConnection(Unknown Source)
at java.sql.DriverManager.getConnection(Unknown Source)
To fix this problem:
Make sure the JDBC driver used by the client is ojdbc7.jar and all other drivers such as ojdbc14.jar are removed.
If the above does not fix this problem, on your Oracle server, open the file $ORACLE_HOME/network/admin/sqlnet.ora and add the line below to it.
SQLNET.ALLOWED_LOGON_VERSION=8
This SQLNET.ALLOWED_LOGON_VERSION parameter specifies the minimum authentication protocol allowed when connecting to Oracle Database instances. The value 8 permits most password versions, and allows any combination of the
DBA_USERS.PASSWORD_VERSIONS
values 10G
, and 11G
.-----------------------------------------------------------------------------------------------------------------
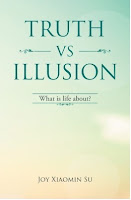
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.