java.net.BindException: Address already in use
at java.net.PlainSocketImpl.socketBind(Native Method)
at java.net.AbstractPlainSocketImpl.bind(AbstractPlainSocketImpl.java:376)
at java.net.ServerSocket.bind(ServerSocket.java:376)
When you disconnect a socket connection and immediately use the same IP address and port number for a new socket, this exception may throw. This is because when a TCP connection is closed, the connection may remain in a timeout state for a period of time after the connection is closed, also known as the TIME_WAIT state or 2MSL wait state. It may not be possible to bind a socket to the requred socket address if there is a connection in the timeout state involving the socket address or port. However, setting the reuse address of the socket to true before binding allows the socket to be bound even though a previous connection is in a timeout state.
For example, the following code throws the exception.
ServerSocket serverSocket = new ServerSocket(<port>);
The following code will not throw the exception.
ServerSocket serverSocket = new ServerSocket();
serverSocket.setReuseAddress(true);
serverSocket.bind(new InetSocketAddress(<port>));
------------------------------------------------------------------------------------------------------------------------
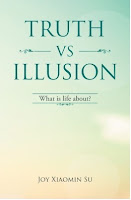
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
at java.net.PlainSocketImpl.socketBind(Native Method)
at java.net.AbstractPlainSocketImpl.bind(AbstractPlainSocketImpl.java:376)
at java.net.ServerSocket.bind(ServerSocket.java:376)
When you disconnect a socket connection and immediately use the same IP address and port number for a new socket, this exception may throw. This is because when a TCP connection is closed, the connection may remain in a timeout state for a period of time after the connection is closed, also known as the TIME_WAIT state or 2MSL wait state. It may not be possible to bind a socket to the requred socket address if there is a connection in the timeout state involving the socket address or port. However, setting the reuse address of the socket to true before binding allows the socket to be bound even though a previous connection is in a timeout state.
For example, the following code throws the exception.
ServerSocket serverSocket = new ServerSocket(<port>);
The following code will not throw the exception.
ServerSocket serverSocket = new ServerSocket();
serverSocket.setReuseAddress(true);
serverSocket.bind(new InetSocketAddress(<port>));
------------------------------------------------------------------------------------------------------------------------
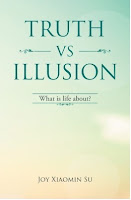
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.