Web browsers such as Google chrome and Microsoft internet explorer fail to go to any websites through https, and give an error page.
In Google chrome, the error page looks like the following
In Microsoft internet explorer, the error page looks like the following.
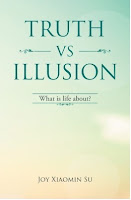
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
In Google chrome, the error page looks like the following
In Microsoft internet explorer, the error page looks like the following.
To fix this problem
- Adjust the date and time of your computer to the current date and time
- Close the web browsers and reopen them
- For Google chrome, click the three bar icon at the top-right corner, choose Settings. Click on the Show Advanced Settings at the bottom. Scroll down, under HTTPS/SSL, click the Manage Certificates button. use the arrows at the top-right corner of the pup-up window to show more tabs, select Trusted Publishers and remove all expired certificates. Then select the Untrusted Publishers tab and remove all the expired certificates.
- For Microsoft internet explorer, click on the setting icon at the top-right corner, choose Internet Options. Select the Content tab, click the Clear SSL State button to clear all the SSL state. Click the Certificates button, use the arrows at the top-right corner of the pup-up window to show more tabs, select Trusted Publishers and remove all expired certificates. Remove all the expired certificates from the Untrusted Publishers tab too.
----------------------------------------------------------------------------------------------------------------
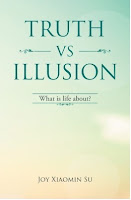
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.