After a new installation of java, occasionally it throws this error when running java.
Following is the procedure to fix it.
Run the command: $JAVA_HOME/bin/java
1. If it returns the usage instruction of java,
Usage: java [-options] class [args...]
(to execute a class)
or java [-options] -jar jarfile [args...]
(to execute a jar file)
........
you have installed java correctly.
If you have multiple versions of java installed on your machine, run command:
$JAVA_HOME/bin/java -version
to check if the correct version is executed. If not, correct your PATH to ensure the correct version of java is used.
2. If executing the command "$JAVA_HOME/bin/java" gives the error:
Error occurred during initialization of VM
java/lang/NoClassDefFoundError: java/lang/Object
it means java is not properly installed. You can reinstall java or do the following.
Check if you can find any .pack files (e.g tools.pack and rt.pack) in the $JAVA_HOME/lib, $JAVA_HOME/jre/lib, and $JAVA_HOME/jre/ext directores. If such files exist, unpack all of them to .jar files with the following command.
$JAVA_HOME/bin/unpack200 -r -v -l "" <file>.pack <file>.jar
e.g. $JAVA_HOME/bin/unpack200 -r -v -l "" $JAVA_HOME/lib/tools.pack $JAVA_HOME/lib/tools.jar
---------------------------------------------------------------------------------------------------
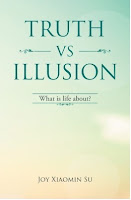
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Following is the procedure to fix it.
Run the command: $JAVA_HOME/bin/java
1. If it returns the usage instruction of java,
Usage: java [-options] class [args...]
(to execute a class)
or java [-options] -jar jarfile [args...]
(to execute a jar file)
........
you have installed java correctly.
If you have multiple versions of java installed on your machine, run command:
$JAVA_HOME/bin/java -version
to check if the correct version is executed. If not, correct your PATH to ensure the correct version of java is used.
2. If executing the command "$JAVA_HOME/bin/java" gives the error:
Error occurred during initialization of VM
java/lang/NoClassDefFoundError: java/lang/Object
it means java is not properly installed. You can reinstall java or do the following.
Check if you can find any .pack files (e.g tools.pack and rt.pack) in the $JAVA_HOME/lib, $JAVA_HOME/jre/lib, and $JAVA_HOME/jre/ext directores. If such files exist, unpack all of them to .jar files with the following command.
$JAVA_HOME/bin/unpack200 -r -v -l "" <file>.pack <file>.jar
e.g. $JAVA_HOME/bin/unpack200 -r -v -l "" $JAVA_HOME/lib/tools.pack $JAVA_HOME/lib/tools.jar
---------------------------------------------------------------------------------------------------
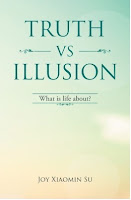
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.