This exception occurs when the SELECT clause contains aggregate functions such as MAX, MIN, SUM, AVG, and COUNT, and there has no GROUP BY clause or the GROUP BY clause does not include all the items in the SELECT clause except the aggregate functions.
For example, the following queries throw such an exception.
1. SELECT NAME, DESCRIPTION, MAX(PRICE) PRICE FROM FRUIT WHERE PRICE < 1.33;
In this case, the query has an aggregate function MAX(PRICE) in the SELECT clause but does not have a GROUP BY clause.
2. SELECT NAME, DESCRIPTION, MAX(PRICE) PRICE FROM FRUIT WHERE PRICE < 1.33 GROUP BY NAME;
In this case, though the GROUP BY clause is there but it dose not contain all the non-aggregate items. The DESCRIPTION is missing from the GROUP BY clause.
To Fix this problem, you may add all the items in the SELECT clause to the GROUP BY clause . However, the GROUP BY clause must have all the non-aggregate items (both NAME and DESCRIPTION in the above queries).
------------------------------------------------------------------------------------------------------------------
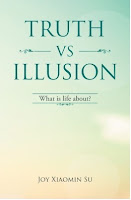
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
For example, the following queries throw such an exception.
1. SELECT NAME, DESCRIPTION, MAX(PRICE) PRICE FROM FRUIT WHERE PRICE < 1.33;
In this case, the query has an aggregate function MAX(PRICE) in the SELECT clause but does not have a GROUP BY clause.
2. SELECT NAME, DESCRIPTION, MAX(PRICE) PRICE FROM FRUIT WHERE PRICE < 1.33 GROUP BY NAME;
In this case, though the GROUP BY clause is there but it dose not contain all the non-aggregate items. The DESCRIPTION is missing from the GROUP BY clause.
To Fix this problem, you may add all the items in the SELECT clause to the GROUP BY clause . However, the GROUP BY clause must have all the non-aggregate items (both NAME and DESCRIPTION in the above queries).
------------------------------------------------------------------------------------------------------------------
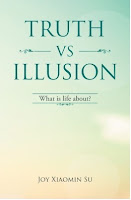
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.