In the case of using JCheckBox in JTable, it is nice to just put the Boolean value in the table model and let the DefaultTableCellRenderer and the DefaultCellEditor to automatically do the job for you. And you don't have to do anything more than just putting the Boolean values in the table model, modifying the getColumnClass method to return Boolean.class for the column, and modifying the isCellEditable method to return true for this column in your table model.
However, when it comes to the case of using JRadioButtons in JTable, the story changes due to the need of exclusive selection. The DefaultTableCellRenderer and the DefaultCellEditor use the same JRadioButton to render and edit all the cells. You have to loop through the table to manually set the Boolean value to false each time a new selection is made. To avoid this, the JRadioButtons can be directly put into the table model, and make the cell renderer and editor to use this same JRadioButton to render and edit the cell. So, these radiobuttons can be added to one or more ButtonGroups for exclusive selection. The trick here is that you need a MouseListener added to your JTable to set selection for the ButtonGroup.
Following is the sample code.
import java.awt.Color;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.ButtonGroup;
import javax.swing.DefaultCellEditor;
import javax.swing.JCheckBox;
import javax.swing.JRadioButton;
import javax.swing.JTable;
import javax.swing.SwingConstants;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableCellEditor;
import javax.swing.table.TableCellRenderer;
public class NewJFrame extends javax.swing.JFrame {
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTable jTable1;
private ButtonGroup radioGroup = new ButtonGroup();
public NewJFrame() {
jScrollPane1 = new javax.swing.JScrollPane();
jTable1 = new javax.swing.JTable();
jScrollPane1.setViewportView(jTable1);
getContentPane().add(jScrollPane1);
setPreferredSize(new Dimension(500,300));
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
pack();
JRadioButton bt1 = new JRadioButton();
JRadioButton bt2 = new JRadioButton();
JRadioButton bt3 = new JRadioButton();
radioGroup.add(bt1);
radioGroup.add(bt2);
radioGroup.add(bt3);
Object[][] data = {{"Item1", bt1}, {"Item2", bt2}, {"Item3", bt3}};
Object[] headers = {"Name", "Select"};
MyTableModel tableModel = new MyTableModel();
tableModel.setDataVector(data, headers);
jTable1.setModel(tableModel);
RadioButtonRendererEditor re = new RadioButtonRendererEditor();
jTable1.getColumn("Select").setCellRenderer(re);
jTable1.getColumn("Select").setCellEditor(re);
jTable1.addMouseListener(new TableListener());
tableModel.fireTableDataChanged();
}
public class MyTableModel extends DefaultTableModel {
@Override
public Class getColumnClass(int colIndex) {
Class type = Object.class;
if (colIndex == 1) {
type = JRadioButton.class;
}
return type;
}
@Override
public boolean isCellEditable(int rowIndex, int columnIndex){
if (columnIndex == 1){
return true;
} else {
return false;
}
}
}
public class TableListener extends MouseAdapter {
@Override
public void mousePressed(MouseEvent e){
int row = jTable1.rowAtPoint(e.getPoint());
int col = jTable1.columnAtPoint(e.getPoint());
if (col == 1){// the radiobutton column
JRadioButton bt = (JRadioButton)jTable1.getValueAt(row, 1);
// radioGroup.setSelected(radioGroup.getSelection(), false);
ButtonModel bm = radioGroup.getSelection();
ButtonModel btM = bt.getModel();
if (bm != null && !bm.equals(btM)){
bm.setSelected(false);
bm.setArmed(false);
bm.setPressed(false);
}
radioGroup.setSelected(btM, true);
((MyTableModel)jTable1.getModel()).fireTableDataChanged();
}
}
}
public class RadioButtonRendererEditor extends DefaultCellEditor
implements TableCellRenderer, TableCellEditor, ItemListener {
private JRadioButton field;
public RadioButtonRendererEditor() {
super(new JCheckBox());
}
public RadioButtonRendererEditor(JCheckBox checkBox) {
super(checkBox);
}
@Override
public Component getTableCellRendererComponent(
JTable table, Object value,
boolean isSelected,
boolean hasFocus,
int row, int col) {
if (value instanceof JRadioButton) {
field = (JRadioButton) value;
field.setHorizontalAlignment(SwingConstants.CENTER);
field.setBackground(Color.WHITE);
}
return field;
}
@Override
public Component getTableCellEditorComponent(
JTable table, Object value, boolean isSelected, int row, int column) {
field = (JRadioButton) value;
field.addItemListener(this);
return field;
}
@Override
public void itemStateChanged(ItemEvent e) {
super.fireEditingStopped();
}
@Override
public Object getCellEditorValue() {
field.removeItemListener(this);
return field;
}
public JRadioButton getValue() {
return field;
}
public void setValue(JRadioButton panel) {
field = panel;
}
}
}
---------------------------------------------------------------------------------------------------------------
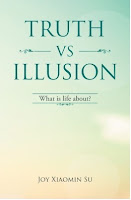
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
However, when it comes to the case of using JRadioButtons in JTable, the story changes due to the need of exclusive selection. The DefaultTableCellRenderer and the DefaultCellEditor use the same JRadioButton to render and edit all the cells. You have to loop through the table to manually set the Boolean value to false each time a new selection is made. To avoid this, the JRadioButtons can be directly put into the table model, and make the cell renderer and editor to use this same JRadioButton to render and edit the cell. So, these radiobuttons can be added to one or more ButtonGroups for exclusive selection. The trick here is that you need a MouseListener added to your JTable to set selection for the ButtonGroup.
Following is the sample code.
import java.awt.Color;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.ButtonGroup;
import javax.swing.DefaultCellEditor;
import javax.swing.JCheckBox;
import javax.swing.JRadioButton;
import javax.swing.JTable;
import javax.swing.SwingConstants;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableCellEditor;
import javax.swing.table.TableCellRenderer;
public class NewJFrame extends javax.swing.JFrame {
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTable jTable1;
private ButtonGroup radioGroup = new ButtonGroup();
public NewJFrame() {
jScrollPane1 = new javax.swing.JScrollPane();
jTable1 = new javax.swing.JTable();
jScrollPane1.setViewportView(jTable1);
getContentPane().add(jScrollPane1);
setPreferredSize(new Dimension(500,300));
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
pack();
JRadioButton bt1 = new JRadioButton();
JRadioButton bt2 = new JRadioButton();
JRadioButton bt3 = new JRadioButton();
radioGroup.add(bt1);
radioGroup.add(bt2);
radioGroup.add(bt3);
Object[][] data = {{"Item1", bt1}, {"Item2", bt2}, {"Item3", bt3}};
Object[] headers = {"Name", "Select"};
MyTableModel tableModel = new MyTableModel();
tableModel.setDataVector(data, headers);
jTable1.setModel(tableModel);
RadioButtonRendererEditor re = new RadioButtonRendererEditor();
jTable1.getColumn("Select").setCellRenderer(re);
jTable1.getColumn("Select").setCellEditor(re);
jTable1.addMouseListener(new TableListener());
tableModel.fireTableDataChanged();
}
public class MyTableModel extends DefaultTableModel {
@Override
public Class getColumnClass(int colIndex) {
Class type = Object.class;
if (colIndex == 1) {
type = JRadioButton.class;
}
return type;
}
@Override
public boolean isCellEditable(int rowIndex, int columnIndex){
if (columnIndex == 1){
return true;
} else {
return false;
}
}
}
public class TableListener extends MouseAdapter {
@Override
public void mousePressed(MouseEvent e){
int row = jTable1.rowAtPoint(e.getPoint());
int col = jTable1.columnAtPoint(e.getPoint());
if (col == 1){// the radiobutton column
JRadioButton bt = (JRadioButton)jTable1.getValueAt(row, 1);
// radioGroup.setSelected(radioGroup.getSelection(), false);
ButtonModel bm = radioGroup.getSelection();
ButtonModel btM = bt.getModel();
if (bm != null && !bm.equals(btM)){
bm.setSelected(false);
bm.setArmed(false);
bm.setPressed(false);
}
radioGroup.setSelected(btM, true);
((MyTableModel)jTable1.getModel()).fireTableDataChanged();
}
}
}
public class RadioButtonRendererEditor extends DefaultCellEditor
implements TableCellRenderer, TableCellEditor, ItemListener {
private JRadioButton field;
public RadioButtonRendererEditor() {
super(new JCheckBox());
}
public RadioButtonRendererEditor(JCheckBox checkBox) {
super(checkBox);
}
@Override
public Component getTableCellRendererComponent(
JTable table, Object value,
boolean isSelected,
boolean hasFocus,
int row, int col) {
if (value instanceof JRadioButton) {
field = (JRadioButton) value;
field.setHorizontalAlignment(SwingConstants.CENTER);
field.setBackground(Color.WHITE);
}
return field;
}
@Override
public Component getTableCellEditorComponent(
JTable table, Object value, boolean isSelected, int row, int column) {
field = (JRadioButton) value;
field.addItemListener(this);
return field;
}
@Override
public void itemStateChanged(ItemEvent e) {
super.fireEditingStopped();
}
@Override
public Object getCellEditorValue() {
field.removeItemListener(this);
return field;
}
public JRadioButton getValue() {
return field;
}
public void setValue(JRadioButton panel) {
field = panel;
}
}
}
---------------------------------------------------------------------------------------------------------------
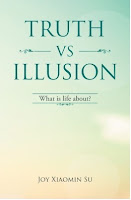
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment