Comparator is a third party class which compares two objects through its compare (T object1, T object2) method. Different implementations of the Comparator interface can compare the same two objects using different fields and result in different outcomes.
Comparable, on the other hand, is a super type of the objects being compared. The class, the instances of which the objects being compared are, implements the Comparable interface and overrides the compareTo (T object) method. There is only one outcome when two objects are compared in this way.
1. Example code of Comparable
Lets say you have an Employee class and you want to order them by their performances.
public class Employee implements Comparable {
private String name;
private int performance;
private int yearsWorked;
private int salary;
public Employee (String nm, int per, int yw, int sa) {
name = nm;
performance = per;
yearsWorked = yw;
salaery = sa;
}
public String getName() { return name; }
public int getPerformance() { return performance; }
public int getYearsWorked() { return yearsWorked; }
public int getSalary() { return salary; }
@Override //Overrides the method in the Comparable interface
public int compareTo (Employee emp) {
return this.getPerformance() - emp.getPerformance();
}
public static void main (String[] args) throws SQLException {
List<Employee> employees = new List<>();
try {
Connection dbConn = <.......>;
PreparedStatement ps = dbConn.prepareStatement(
"select name, performance, yearsWorked, salary from employee");
ResultSet rs = ps.executeQuery()) {
while(rs.next()) {
employees.add(new Employee(rs.getString("name"),
rs.getInt("performance"),
rs.getInt("yearsWorked").
rs.getInt("salary"));
}
}
//Sort employees by performance and print them on screen
//Collections.sort(employees);
employees.stream()
.sorted()
.map(Employee::getName)
.forEach(System.out::println);
}
}
2. Example code of Comparator
Now, you also want to order the employees by years worked or by salary. The code shows how to compare by years worked. It is similar to compare by salary.
public class CompareByYearsWorked<Employee> implements Comparator<Employee> {
public int compare (Employee e1, Employee e2) {
return e1.getYearsWorked() - e2.getYearsWorked();
}
public boolean equals(Object obj) {
return false;
}
}
Add the segment of code to the end of the main method after you printed the employees in order of their performances.
//employees.sort(new CompareByYearsWorked());
employees.stream()
.sorted (new CompareByYearsWorked())
.map(Employee::getName)
.forEach(System.out::println);
You can always use Comparator to compare two object instances of a class. However, the code of using Comparable is more simple and does not require you to pass a parameter of the Comparator when being used. But you can only use Comparable to compare one thing of the objects.
-------------------------------------------------------------------------------------------------------------
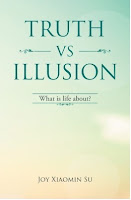
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Comparable, on the other hand, is a super type of the objects being compared. The class, the instances of which the objects being compared are, implements the Comparable interface and overrides the compareTo (T object) method. There is only one outcome when two objects are compared in this way.
1. Example code of Comparable
Lets say you have an Employee class and you want to order them by their performances.
public class Employee implements Comparable {
private String name;
private int performance;
private int yearsWorked;
private int salary;
public Employee (String nm, int per, int yw, int sa) {
name = nm;
performance = per;
yearsWorked = yw;
salaery = sa;
}
public String getName() { return name; }
public int getPerformance() { return performance; }
public int getYearsWorked() { return yearsWorked; }
public int getSalary() { return salary; }
@Override //Overrides the method in the Comparable interface
public int compareTo (Employee emp) {
return this.getPerformance() - emp.getPerformance();
}
public static void main (String[] args) throws SQLException {
List<Employee> employees = new List<>();
try {
Connection dbConn = <.......>;
PreparedStatement ps = dbConn.prepareStatement(
"select name, performance, yearsWorked, salary from employee");
ResultSet rs = ps.executeQuery()) {
while(rs.next()) {
employees.add(new Employee(rs.getString("name"),
rs.getInt("performance"),
rs.getInt("yearsWorked").
rs.getInt("salary"));
}
}
//Sort employees by performance and print them on screen
//Collections.sort(employees);
employees.stream()
.sorted()
.map(Employee::getName)
.forEach(System.out::println);
}
}
2. Example code of Comparator
Now, you also want to order the employees by years worked or by salary. The code shows how to compare by years worked. It is similar to compare by salary.
public class CompareByYearsWorked<Employee> implements Comparator<Employee> {
public int compare (Employee e1, Employee e2) {
return e1.getYearsWorked() - e2.getYearsWorked();
}
public boolean equals(Object obj) {
return false;
}
}
Add the segment of code to the end of the main method after you printed the employees in order of their performances.
//employees.sort(new CompareByYearsWorked());
employees.stream()
.sorted (new CompareByYearsWorked())
.map(Employee::getName)
.forEach(System.out::println);
You can always use Comparator to compare two object instances of a class. However, the code of using Comparable is more simple and does not require you to pass a parameter of the Comparator when being used. But you can only use Comparable to compare one thing of the objects.
-------------------------------------------------------------------------------------------------------------
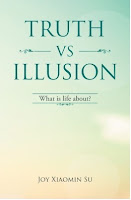
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment