To print a document using a printer, you must have one or more printers connected to your computer. The javax.print packages have all the java classes for doing this job. Following is an sample code to print a text document using a printer.
public class PrintTest {
public static void main(String[] args) {
byte[] bytes = null;
try {
//read the file to be printed into a byte array
Path path = FileSystems.getDefault().getPath("<file directory>","<file name>");
bytes = Files.readAllBytes(path);
} catch (IOException ffne) {//FileNotFoundException ffne) {
ffne.printStackTrace();
}
//create the Doc object using the byte array
DocFlavor docFlavor = DocFlavor.BYTE_ARRAY.AUTOSENSE;
// DocAttributeSet daset = new HashDocAttributeSet();
// daset.add(OrientationRequested.LANDSCAPE);
// daset.add(Sides.ONE_SIDED);
Doc myDoc = new SimpleDoc(bytes, docFlavor, daset);
//create the PrintRequestAttributeSet
PrintRequestAttributeSet aset = new HashPrintRequestAttributeSet();
// aset.add(new Copies(5));
// aset.add(Sides.ONE_SIDED);
// aset.add(Sides.TWO_SIDED_LONG_EDGE);
// aset.add(MediaName.NA_LETTER_WHITE);
// aset.add(MediaSizeName.NA_LETTER);
// aset.add(MediaTray.TOP);
// aset.add(OrientationRequested.LANDSCAPE);
//Find the printer that maches the name that you would like to use
PrintService[] services = PrintServiceLookup.lookupPrintServices(docFlavor, null);
for (PrintService service : services) {
if (service.getName().equalsIgnoreCase("<your printer name>")) {
//Manually confirm the printer or select another printer
// service = ServiceUI.printDialog(null, 250, 250, services, service,
// docFlavor, aset);
//create the DocPrintJob
DocPrintJob job = service.createPrintJob();
try {
//print the document
job.print(myDoc, aset);
} catch (PrintException pe) {
pe.printStackTrace();
}
}
}
}
}
---------------------------------------------------------------------------------------------------------------
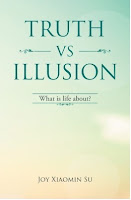
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
public class PrintTest {
public static void main(String[] args) {
byte[] bytes = null;
try {
//read the file to be printed into a byte array
Path path = FileSystems.getDefault().getPath("<file directory>","<file name>");
bytes = Files.readAllBytes(path);
} catch (IOException ffne) {//FileNotFoundException ffne) {
ffne.printStackTrace();
}
//create the Doc object using the byte array
DocFlavor docFlavor = DocFlavor.BYTE_ARRAY.AUTOSENSE;
// DocAttributeSet daset = new HashDocAttributeSet();
// daset.add(OrientationRequested.LANDSCAPE);
// daset.add(Sides.ONE_SIDED);
Doc myDoc = new SimpleDoc(bytes, docFlavor, daset);
//create the PrintRequestAttributeSet
PrintRequestAttributeSet aset = new HashPrintRequestAttributeSet();
// aset.add(new Copies(5));
// aset.add(Sides.ONE_SIDED);
// aset.add(Sides.TWO_SIDED_LONG_EDGE);
// aset.add(MediaName.NA_LETTER_WHITE);
// aset.add(MediaSizeName.NA_LETTER);
// aset.add(MediaTray.TOP);
// aset.add(OrientationRequested.LANDSCAPE);
//Find the printer that maches the name that you would like to use
PrintService[] services = PrintServiceLookup.lookupPrintServices(docFlavor, null);
for (PrintService service : services) {
if (service.getName().equalsIgnoreCase("<your printer name>")) {
//Manually confirm the printer or select another printer
// service = ServiceUI.printDialog(null, 250, 250, services, service,
// docFlavor, aset);
//create the DocPrintJob
DocPrintJob job = service.createPrintJob();
try {
//print the document
job.print(myDoc, aset);
} catch (PrintException pe) {
pe.printStackTrace();
}
}
}
}
}
---------------------------------------------------------------------------------------------------------------
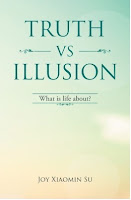
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Thank you for code,But I can not print a txt file with it:(
ReplyDelete