The Files class was introduced in Java 7. It contains only static methods that are convenient to use for operations on a file.
1. the readAllLines method
List<String> allLines = Files.readAllLines(theFile);
2. the newBufferedReader
BufferedReader reader = Files.newBufferedReader(theFile);
String line = "";
for ((line = reader.readLine()) != null) {
System.out.println(line);
}
3. the lines method
Stream<String> lineStream = Files.lines(theFile);
List<String> lineList = lineStream.collect(Collectors.toList());
4. the readAllBytes method
byte[] allByes = Files.readAllBytes(theFile);
5. the newInputStream method
InputStream is = Files.newInputStream(theFile);
byte[] isBytes = new byte[Files.size(theFile)];
is.read(isBytes); //read the file to the byte array
6. the newByteChannel method
//The advantage of using SeekableByteChannel is that it allows you
//to set an arbitrary position to start reading and writing
SeekableByteChannel sbc = Files.newByteChannel(theFile);
String encode = System.getProperty("file.encoding");
ByteBuffer theBuffer = ByteBuffer.allocate(theFile.toFile().length());
sbc.read(theBuffer);
theBuffer.reWind();
String theString = CharSet.forName(encode).decode(theBuffer).toString();
1. the newBufferedWriter method
BufferedWriter writer = Files.newBufferedWriter(theFile);
PrintWriter pw = new PrintWriter(writer);
2. the newOutputStream method
OutputStream os = Files.newOutputStream(theFile);
3. the write method
byte[] theBytes = (new String("The words to write")).getBytes();
Files.write(theFile, theBytes);
4. the newByteChannel method
SeekableByteChannel sbc = Files.newByteChannel(theFile);
byte[] theBytes = (new String("The words to write")).getBytes();
ByteBuffer theBuffer = ByteBuffer.wrap(theBytes);
sbc.write(theBuffer);
> Next
2. Read from and write to the command line in Java
3. Java communications over network
4. Class Files
---------------------------------------------------------------------------------------------------------------
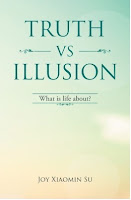
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
A. Read from a file
Path theFile = FileSystems.getDefault().getPath("<dirctory>", "<file name>");1. the readAllLines method
List<String> allLines = Files.readAllLines(theFile);
2. the newBufferedReader
BufferedReader reader = Files.newBufferedReader(theFile);
String line = "";
for ((line = reader.readLine()) != null) {
System.out.println(line);
}
3. the lines method
Stream<String> lineStream = Files.lines(theFile);
List<String> lineList = lineStream.collect(Collectors.toList());
4. the readAllBytes method
byte[] allByes = Files.readAllBytes(theFile);
5. the newInputStream method
InputStream is = Files.newInputStream(theFile);
byte[] isBytes = new byte[Files.size(theFile)];
is.read(isBytes); //read the file to the byte array
6. the newByteChannel method
//The advantage of using SeekableByteChannel is that it allows you
//to set an arbitrary position to start reading and writing
SeekableByteChannel sbc = Files.newByteChannel(theFile);
String encode = System.getProperty("file.encoding");
ByteBuffer theBuffer = ByteBuffer.allocate(theFile.toFile().length());
sbc.read(theBuffer);
theBuffer.reWind();
String theString = CharSet.forName(encode).decode(theBuffer).toString();
B. Write to a file
Path theFile = FileSystems.getDefault().getPath("<dirctory>", "<file name>");1. the newBufferedWriter method
BufferedWriter writer = Files.newBufferedWriter(theFile);
PrintWriter pw = new PrintWriter(writer);
2. the newOutputStream method
OutputStream os = Files.newOutputStream(theFile);
3. the write method
byte[] theBytes = (new String("The words to write")).getBytes();
Files.write(theFile, theBytes);
4. the newByteChannel method
SeekableByteChannel sbc = Files.newByteChannel(theFile);
byte[] theBytes = (new String("The words to write")).getBytes();
ByteBuffer theBuffer = ByteBuffer.wrap(theBytes);
sbc.write(theBuffer);
> Next
References:
1. Read from and write to a file in Java (2): Other commonly used methods2. Read from and write to the command line in Java
3. Java communications over network
4. Class Files
---------------------------------------------------------------------------------------------------------------
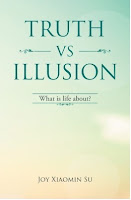
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment