The following code throws such an exception. The line of code that causes the exception is marked in yellow. The reason that this exception occurs is that the program uses a list of beans instead an array of beans to hold the data of the subreport. Therefore, to fix this problem, you just need to change the "exp.subDatasourceBeanArray" to "exp.subDatasourceBeanCollection" in the marked code below.
import java.util.ArrayList;
import java.util.List;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import static net.sf.dynamicreports.report.builder.DynamicReports.cmp;
import static net.sf.dynamicreports.report.builder.DynamicReports.col;
import static net.sf.dynamicreports.report.builder.DynamicReports.exp;
import static net.sf.dynamicreports.report.builder.DynamicReports.report;
import static net.sf.dynamicreports.report.builder.DynamicReports.stl;
import net.sf.dynamicreports.report.builder.component.SubreportBuilder;
import net.sf.dynamicreports.report.builder.datatype.DataTypes;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
import net.sf.dynamicreports.report.constant.StretchType;
import net.sf.dynamicreports.report.exception.DRException;
import net.sf.jasperreports.engine.data.JRBeanCollectionDataSource;
public class PageSubreportTest {
public static void main(String[] args){
new PageSubreportTest();
}
public PageSubreportTest() {
JasperReportBuilder report = build();
try {
report.show();
}catch (DRException e){
e.printStackTrace();
}
}
private JasperReportBuilder build() {
JasperReportBuilder report = report();
report.setDataSource(createDataSource());
SubreportBuilder storeSubreport = cmp.subreport(buildStoreSubReport())
.setDataSource(exp.subDatasourceBeanCollection("storeData"));
SubreportBuilder fruitSubreport = cmp.subreport(buildFruitSubReport())
.setDataSource(exp.subDatasourceBeanArray("fruitData"));
report.detail(cmp.horizontalList(cmp.horizontalGap(50),storeSubreport, cmp.horizontalGap(50)),
cmp.verticalGap(15),
//To set the border for the fruitSubreport
//put it in a horizontallist and then set the border
cmp.horizontalList(fruitSubreport)
.setStretchType(StretchType.RELATIVE_TO_TALLEST_OBJECT)
.setStyle(stl.style().setBorder(stl.pen1Point())));
//For page subreport, set the page break at the end.
// cmp.pageBreak());
report.title(cmp.text("Fuit Stores Report")
.setStyle(stl.style().bold()
.setHorizontalAlignment(HorizontalAlignment.CENTER)));
report.pageFooter(cmp.pageXofY());
return report;
}
private JasperReportBuilder buildStoreSubReport(){
JasperReportBuilder storeReport = report();
storeReport.columns(col.column("", "storeInfo", DataTypes.stringType()));
return storeReport;
}
private JasperReportBuilder buildFruitSubReport(){
JasperReportBuilder fruitReport = report();
fruitReport.columns(col.column("Name", "fruitName", DataTypes.stringType()),
col.column("Price", "fruitPrice", DataTypes.doubleType())
.setPattern("$#,###,##0.00")
.setHorizontalAlignment(HorizontalAlignment.LEFT),
col.column("Description", "des", DataTypes.stringType()));
fruitReport.setColumnTitleStyle(stl.style().bold());
return fruitReport;
}
private JRBeanCollectionDataSource createDataSource() {
List<ReportData> dataSource = new ArrayList<ReportData>();
ReportData data = new ReportData();
List<StoreData> storeList = new ArrayList<StoreData>();
List<FruitData> fruitList = new ArrayList<FruitData>();
//first set of data
StoreData storeData = new StoreData();
storeData.setStoreInfo("Delicious Fruit");
storeList.add(storeData);
storeData = new StoreData();
storeData.setStoreInfo("554 Main Street, Burlington, MN 33596");
storeList.add(storeData);
data.setStoreData(storeList);
FruitData fruitData = new FruitData();
fruitData.setFruitName("Apple");
fruitData.setFruitPrice(1.98d);
fruitData.setDes("Medium juicy");
fruitList.add(fruitData);
fruitData = new FruitData();
fruitData.setFruitName("Orange");
fruitData.setFruitPrice(0.98d);
fruitData.setDes("Very juicy");
fruitList.add(fruitData);
data.setFruitData(fruitList);
dataSource.add(data);
//second set of data
data = new ReportData();
storeList = new ArrayList<StoreData>();
fruitList = new ArrayList<FruitData>();
storeData = new StoreData();
storeData.setStoreInfo("Fruit Outlet");
storeList.add(storeData);
storeData = new StoreData();
storeData.setStoreInfo("99 Uphill Ave., Rochester, MN 33596");
storeList.add(storeData);
data.setStoreData(storeList);
fruitData = new FruitData();
fruitData.setFruitName("Papaya");
fruitData.setFruitPrice(2.98d);
fruitData.setDes("Medium juicy");
fruitList.add(fruitData);
fruitData = new FruitData();
fruitData.setFruitName("Mango");
fruitData.setFruitPrice(3.98d);
fruitData.setDes("Medium juicy");
fruitList.add(fruitData);
data.setFruitData(fruitList);
dataSource.add(data);
return new JRBeanCollectionDataSource(dataSource);
}
public class ReportData {
private List<StoreData> storeData;
private List<FruitData> fruitData;
public List<StoreData> getStoreData() { return storeData; }
public void setStoreData(List<StoreData> s) { storeData = s; }
public List<FruitData> getFruitData() { return fruitData; }
public void setFruitData (List<FruitData> s) { fruitData = s; }
}
public class StoreData {
private String storeInfo;
public String getStoreInfo() { return storeInfo; }
public void setStoreInfo(String s) { storeInfo = s; }
}
public class FruitData {
private String fruitName;
private Double fruitPrice;
private String des;
public String getFruitName() { return fruitName; }
public void setFruitName(String s) { fruitName = s; }
public Double getFruitPrice() { return fruitPrice; }
public void setFruitPrice(Double s) { fruitPrice = s; }
public String getDes() { return des; }
public void setDes(String s) { des = s; }
}
}
------------------------------------------------------------------------------------------------------------
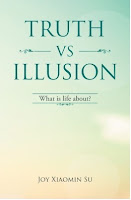
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
import java.util.ArrayList;
import java.util.List;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import static net.sf.dynamicreports.report.builder.DynamicReports.cmp;
import static net.sf.dynamicreports.report.builder.DynamicReports.col;
import static net.sf.dynamicreports.report.builder.DynamicReports.exp;
import static net.sf.dynamicreports.report.builder.DynamicReports.report;
import static net.sf.dynamicreports.report.builder.DynamicReports.stl;
import net.sf.dynamicreports.report.builder.component.SubreportBuilder;
import net.sf.dynamicreports.report.builder.datatype.DataTypes;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
import net.sf.dynamicreports.report.constant.StretchType;
import net.sf.dynamicreports.report.exception.DRException;
import net.sf.jasperreports.engine.data.JRBeanCollectionDataSource;
public class PageSubreportTest {
public static void main(String[] args){
new PageSubreportTest();
}
public PageSubreportTest() {
JasperReportBuilder report = build();
try {
report.show();
}catch (DRException e){
e.printStackTrace();
}
}
private JasperReportBuilder build() {
JasperReportBuilder report = report();
report.setDataSource(createDataSource());
SubreportBuilder storeSubreport = cmp.subreport(buildStoreSubReport())
.setDataSource(exp.subDatasourceBeanCollection("storeData"));
SubreportBuilder fruitSubreport = cmp.subreport(buildFruitSubReport())
.setDataSource(exp.subDatasourceBeanArray("fruitData"));
report.detail(cmp.horizontalList(cmp.horizontalGap(50),storeSubreport, cmp.horizontalGap(50)),
cmp.verticalGap(15),
//To set the border for the fruitSubreport
//put it in a horizontallist and then set the border
cmp.horizontalList(fruitSubreport)
.setStretchType(StretchType.RELATIVE_TO_TALLEST_OBJECT)
.setStyle(stl.style().setBorder(stl.pen1Point())));
//For page subreport, set the page break at the end.
// cmp.pageBreak());
report.title(cmp.text("Fuit Stores Report")
.setStyle(stl.style().bold()
.setHorizontalAlignment(HorizontalAlignment.CENTER)));
report.pageFooter(cmp.pageXofY());
return report;
}
private JasperReportBuilder buildStoreSubReport(){
JasperReportBuilder storeReport = report();
storeReport.columns(col.column("", "storeInfo", DataTypes.stringType()));
return storeReport;
}
private JasperReportBuilder buildFruitSubReport(){
JasperReportBuilder fruitReport = report();
fruitReport.columns(col.column("Name", "fruitName", DataTypes.stringType()),
col.column("Price", "fruitPrice", DataTypes.doubleType())
.setPattern("$#,###,##0.00")
.setHorizontalAlignment(HorizontalAlignment.LEFT),
col.column("Description", "des", DataTypes.stringType()));
fruitReport.setColumnTitleStyle(stl.style().bold());
return fruitReport;
}
private JRBeanCollectionDataSource createDataSource() {
List<ReportData> dataSource = new ArrayList<ReportData>();
ReportData data = new ReportData();
List<StoreData> storeList = new ArrayList<StoreData>();
List<FruitData> fruitList = new ArrayList<FruitData>();
//first set of data
StoreData storeData = new StoreData();
storeData.setStoreInfo("Delicious Fruit");
storeList.add(storeData);
storeData = new StoreData();
storeData.setStoreInfo("554 Main Street, Burlington, MN 33596");
storeList.add(storeData);
data.setStoreData(storeList);
FruitData fruitData = new FruitData();
fruitData.setFruitName("Apple");
fruitData.setFruitPrice(1.98d);
fruitData.setDes("Medium juicy");
fruitList.add(fruitData);
fruitData = new FruitData();
fruitData.setFruitName("Orange");
fruitData.setFruitPrice(0.98d);
fruitData.setDes("Very juicy");
fruitList.add(fruitData);
data.setFruitData(fruitList);
dataSource.add(data);
//second set of data
data = new ReportData();
storeList = new ArrayList<StoreData>();
fruitList = new ArrayList<FruitData>();
storeData = new StoreData();
storeData.setStoreInfo("Fruit Outlet");
storeList.add(storeData);
storeData = new StoreData();
storeData.setStoreInfo("99 Uphill Ave., Rochester, MN 33596");
storeList.add(storeData);
data.setStoreData(storeList);
fruitData = new FruitData();
fruitData.setFruitName("Papaya");
fruitData.setFruitPrice(2.98d);
fruitData.setDes("Medium juicy");
fruitList.add(fruitData);
fruitData = new FruitData();
fruitData.setFruitName("Mango");
fruitData.setFruitPrice(3.98d);
fruitData.setDes("Medium juicy");
fruitList.add(fruitData);
data.setFruitData(fruitList);
dataSource.add(data);
return new JRBeanCollectionDataSource(dataSource);
}
public class ReportData {
private List<StoreData> storeData;
private List<FruitData> fruitData;
public List<StoreData> getStoreData() { return storeData; }
public void setStoreData(List<StoreData> s) { storeData = s; }
public List<FruitData> getFruitData() { return fruitData; }
public void setFruitData (List<FruitData> s) { fruitData = s; }
}
public class StoreData {
private String storeInfo;
public String getStoreInfo() { return storeInfo; }
public void setStoreInfo(String s) { storeInfo = s; }
}
public class FruitData {
private String fruitName;
private Double fruitPrice;
private String des;
public String getFruitName() { return fruitName; }
public void setFruitName(String s) { fruitName = s; }
public Double getFruitPrice() { return fruitPrice; }
public void setFruitPrice(Double s) { fruitPrice = s; }
public String getDes() { return des; }
public void setDes(String s) { des = s; }
}
}
------------------------------------------------------------------------------------------------------------
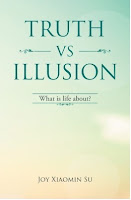
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment