There are two ways to achieve this result. One way is to use the setRows and setColumns method to set the size of the JTextArea. The other way is making your text area the subclass of the JTextArea and override the paint() method to get the width and height of the font used. Then, set the size of your text area.
Since for many types of font, the characters do not always have the same widths, some characters are wider than the others, it is better that you use the monospaced font for achieving a constant result.
Following is an example.
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import javax.swing.BorderFactory;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextArea;
public class CharInLine {
private int charWidth = 0;
private int charHeight = 0;
private int charPerLine = 30;
public CharInLine() {
MyTextArea textArea = new MyTextArea();
textArea.setFont(new Font("monospaced", Font.PLAIN, 12));
//Method 1
//setting the rows and columns is important for an
//empty JTextArea to show its size.
textArea.setRows(5);
textArea.setColumns(30);
textArea.setBorder(BorderFactory.createTitledBorder("Text Area"));
//Method 2
//designate 30 characters per line and 5 lines in the text area
textArea.setLineWrap(true);
textArea.setSize(new Dimension(charWidth*charPerLine, charHeight*5));
//textArea.setPreferredSize(new Dimension(charWidth*charPerLine, charHeight*5));
JPanel panel = new JPanel();
panel.add(textArea);
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(panel,BorderLayout.CENTER);
frame.setSize(500, 300);
frame.setVisible(true);
}
private class MyTextArea extends JTextArea {
@Override
public void paint(Graphics g){
super.paint(g);
FontMetrics fm = g.getFontMetrics();
charWidth = fm.charWidth('M');
charHeight = fm.getHeight();
}
}
public int getCharWidth() {
return charWidth;
}
public static void main(String[] args){
new CharInLine();
}
}
-----------------------------------------------------------------------------------------------------------------
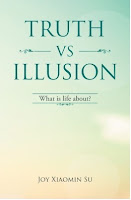
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Since for many types of font, the characters do not always have the same widths, some characters are wider than the others, it is better that you use the monospaced font for achieving a constant result.
Following is an example.
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import javax.swing.BorderFactory;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextArea;
public class CharInLine {
private int charWidth = 0;
private int charHeight = 0;
private int charPerLine = 30;
public CharInLine() {
MyTextArea textArea = new MyTextArea();
textArea.setFont(new Font("monospaced", Font.PLAIN, 12));
//Method 1
//setting the rows and columns is important for an
//empty JTextArea to show its size.
textArea.setRows(5);
textArea.setColumns(30);
textArea.setBorder(BorderFactory.createTitledBorder("Text Area"));
//Method 2
//designate 30 characters per line and 5 lines in the text area
textArea.setLineWrap(true);
textArea.setSize(new Dimension(charWidth*charPerLine, charHeight*5));
//textArea.setPreferredSize(new Dimension(charWidth*charPerLine, charHeight*5));
JPanel panel = new JPanel();
panel.add(textArea);
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(panel,BorderLayout.CENTER);
frame.setSize(500, 300);
frame.setVisible(true);
}
private class MyTextArea extends JTextArea {
@Override
public void paint(Graphics g){
super.paint(g);
FontMetrics fm = g.getFontMetrics();
charWidth = fm.charWidth('M');
charHeight = fm.getHeight();
}
}
public int getCharWidth() {
return charWidth;
}
public static void main(String[] args){
new CharInLine();
}
}
Reference:
1. setSize vs setPreferredSize in JTextArea, JTextField, JButton, JLabel, JPanel and JFrame-----------------------------------------------------------------------------------------------------------------
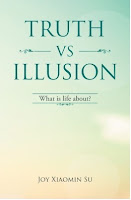
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment