Following are the steps of setting the maximum numbers of characters that a JTextArea can take.
- Create a Document class that extends the PlainDocument class.
- In the class create a maxSize field.
- Override the insertString method so that only the maxSize or less number of characters can be inserted into the document.
- Create a setMaxSize method for setting the value of the maxSize.
- Create a Text Area class that extends the JTextArea, which uses the Document created in step 1 as its default document. Add a setMaxSize method to the class that calls the Document's setMaxSize mthod to set the max size for the Document.
Here is the sample code.
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextArea;
import javax.swing.text.AttributeSet;
import javax.swing.text.BadLocationException;
import javax.swing.text.PlainDocument;
public class TextAreaSize {
private class MyDocument extends PlainDocument {
private int maxSize = 1000000000;
@Override
public void insertString(int offset, String s, AttributeSet attributeSet)
throws BadLocationException {
int length = s.length();
if (length == 0) {
return;
}
if (offset + length > maxSize) {
s = s.substring(0, maxSize - offset);
}
super.insertString(offset, s, attributeSet);
}
public void setMaxSize(int size) {
maxSize = size;
}
}
private class MyTextArea extends JTextArea {
private MyDocument document;
public MyTextArea (MyDocument d){
super(d);
document = d;
}
public void setMaxSize(int s){
document.setMaxSize(s);
}
}
public TextAreaSize() {
MyTextArea textArea = new MyTextArea(new MyDocument());
textArea.setMaxSize(30);
textArea.setRows(2);
textArea.setColumns(20);
JPanel panel = new JPanel();
panel.add(textArea);
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(panel);
frame.setSize(500, 300);
frame.setVisible(true);
}
public static void main(String[] args){
new TextAreaSize();
}
}
----------------------------------------------------------------------------------------------------------------
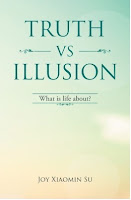
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
----------------------------------------------------------------------------------------------------------------
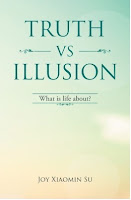
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment