The ConditionalStyleBuilder can be used to set the style of a column conditionally. Following sample code shows how to set the background color to Orange and font to bold when the Price value is more than 1.
import java.awt.Color;
import java.math.BigDecimal;
import java.text.SimpleDateFormat;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import net.sf.dynamicreports.report.base.expression.AbstractSimpleExpression;
import net.sf.dynamicreports.report.builder.DynamicReports;
import net.sf.dynamicreports.report.builder.column.Columns;
import net.sf.dynamicreports.report.builder.column.TextColumnBuilder;
import net.sf.dynamicreports.report.builder.component.Components;
import net.sf.dynamicreports.report.builder.datatype.BigDecimalType;
import net.sf.dynamicreports.report.builder.datatype.DataTypes;
import net.sf.dynamicreports.report.builder.style.ConditionalStyleBuilder;
import net.sf.dynamicreports.report.builder.style.StyleBuilder;
import net.sf.dynamicreports.report.builder.style.Styles;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
import net.sf.dynamicreports.report.constant.PageType;
import net.sf.dynamicreports.report.datasource.DRDataSource;
import net.sf.dynamicreports.report.definition.ReportParameters;
import net.sf.dynamicreports.report.exception.DRException;
import net.sf.jasperreports.engine.JRDataSource;
public class DynamicReportsTest {
public DynamicReportsTest() {
build();
}
public void build() {
JasperReportBuilder report = DynamicReports.report();
report.setPageFormat(PageType.LETTER);
// report.setPageFormat(PageType.A4, PageOrientation.PORTRAIT);
CurrencyType ct = new CurrencyType();
TextColumnBuilder<BigDecimal> priceColumn = Columns.column("Price", "price", ct);
TextColumnBuilder<Integer> quantityOrderedColumn = Columns.column("Quantity Ordered", "quantityOrdered", DataTypes.integerType());
TextColumnBuilder<BigDecimal> totalPay = priceColumn.multiply(quantityOrderedColumn)
.setTitle("Amount Paid")
.setPattern(ct.getPattern());
report.columns(Columns.columnRowNumberColumn("Item"),
Columns.column("Name", "name", DataTypes.stringType()),
priceColumn,
quantityOrderedColumn,
totalPay
);
StyleBuilder bold = Styles.style().bold();
StyleBuilder centeredBold = Styles.style(bold)
.setHorizontalAlignment(HorizontalAlignment.CENTER);
StyleBuilder columnStyle = Styles.style(centeredBold);
//Create the ConditionalStyleBuilder
ConditionalStyleBuilder condColumnStyle = Styles.conditionalStyle(new CCExpression())
.bold()
.setHorizontalAlignment(HorizontalAlignment.CENTER)//.style(centeredBold)
.setBackgroundColor(Color.ORANGE)
.setBorder(Styles.pen1Point());
//Create a StyleBuilder using the ConditionalStyleBuilder
StyleBuilder priceStyle = Styles.style().conditionalStyles(condColumnStyle);
report.setColumnTitleStyle(columnStyle);
report.setColumnStyle(Styles.style().setHorizontalAlignment(HorizontalAlignment.CENTER));
//Apply the StyleBuilder to a report column
priceColumn.setStyle(priceStyle);
report.highlightDetailEvenRows();
report.title(Components.text("Test Report")
.setStyle(centeredBold));
report.title(Components.text("Detailed Report")
.setStyle(centeredBold));
SimpleDateFormat format = new SimpleDateFormat("MM/dd/YY");
report.pageFooter(Components.horizontalFlowList().add(Components.text("Page "))
.add(Components.pageNumber())
.setStyle(bold));
report.setDataSource(createDataSource());
try {
report.show();
}catch(DRException e){
e.printStackTrace();
}
}
private class CCExpression extends AbstractSimpleExpression<Boolean> {
public Boolean evaluate (ReportParameters param){
BigDecimal price = param.getFieldValue("price");
if (price.doubleValue() > 1){
return true;
} else {
return false;
}
}
}
private class CurrencyType extends BigDecimalType {
@Override
public String getPattern() {
return "$ #,###.##";
}
}
private JRDataSource createDataSource() {
DRDataSource dataSource = new DRDataSource("name", "price", "quantityOrdered");
dataSource.add("Apple", new BigDecimal(1.29), 120);
dataSource.add("Apple", new BigDecimal(1.69), 150);
dataSource.add("Orange", new BigDecimal(0.99), 130);
dataSource.add("Orange", new BigDecimal(0.96), 100);
dataSource.add("Mange", new BigDecimal(0), 300);
return dataSource;
}
public static void main(String[] args){
new DynamicReportsTest();
}
}
---------------------------------------------------------------------------------------------------------------------
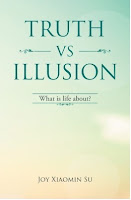
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
import java.awt.Color;
import java.math.BigDecimal;
import java.text.SimpleDateFormat;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import net.sf.dynamicreports.report.base.expression.AbstractSimpleExpression;
import net.sf.dynamicreports.report.builder.DynamicReports;
import net.sf.dynamicreports.report.builder.column.Columns;
import net.sf.dynamicreports.report.builder.column.TextColumnBuilder;
import net.sf.dynamicreports.report.builder.component.Components;
import net.sf.dynamicreports.report.builder.datatype.BigDecimalType;
import net.sf.dynamicreports.report.builder.datatype.DataTypes;
import net.sf.dynamicreports.report.builder.style.ConditionalStyleBuilder;
import net.sf.dynamicreports.report.builder.style.StyleBuilder;
import net.sf.dynamicreports.report.builder.style.Styles;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
import net.sf.dynamicreports.report.constant.PageType;
import net.sf.dynamicreports.report.datasource.DRDataSource;
import net.sf.dynamicreports.report.definition.ReportParameters;
import net.sf.dynamicreports.report.exception.DRException;
import net.sf.jasperreports.engine.JRDataSource;
public class DynamicReportsTest {
public DynamicReportsTest() {
build();
}
public void build() {
JasperReportBuilder report = DynamicReports.report();
report.setPageFormat(PageType.LETTER);
// report.setPageFormat(PageType.A4, PageOrientation.PORTRAIT);
CurrencyType ct = new CurrencyType();
TextColumnBuilder<BigDecimal> priceColumn = Columns.column("Price", "price", ct);
TextColumnBuilder<Integer> quantityOrderedColumn = Columns.column("Quantity Ordered", "quantityOrdered", DataTypes.integerType());
TextColumnBuilder<BigDecimal> totalPay = priceColumn.multiply(quantityOrderedColumn)
.setTitle("Amount Paid")
.setPattern(ct.getPattern());
report.columns(Columns.columnRowNumberColumn("Item"),
Columns.column("Name", "name", DataTypes.stringType()),
priceColumn,
quantityOrderedColumn,
totalPay
);
StyleBuilder bold = Styles.style().bold();
StyleBuilder centeredBold = Styles.style(bold)
.setHorizontalAlignment(HorizontalAlignment.CENTER);
StyleBuilder columnStyle = Styles.style(centeredBold);
//Create the ConditionalStyleBuilder
ConditionalStyleBuilder condColumnStyle = Styles.conditionalStyle(new CCExpression())
.bold()
.setHorizontalAlignment(HorizontalAlignment.CENTER)//.style(centeredBold)
.setBackgroundColor(Color.ORANGE)
.setBorder(Styles.pen1Point());
//Create a StyleBuilder using the ConditionalStyleBuilder
StyleBuilder priceStyle = Styles.style().conditionalStyles(condColumnStyle);
report.setColumnTitleStyle(columnStyle);
report.setColumnStyle(Styles.style().setHorizontalAlignment(HorizontalAlignment.CENTER));
//Apply the StyleBuilder to a report column
priceColumn.setStyle(priceStyle);
report.highlightDetailEvenRows();
report.title(Components.text("Test Report")
.setStyle(centeredBold));
report.title(Components.text("Detailed Report")
.setStyle(centeredBold));
SimpleDateFormat format = new SimpleDateFormat("MM/dd/YY");
report.pageFooter(Components.horizontalFlowList().add(Components.text("Page "))
.add(Components.pageNumber())
.setStyle(bold));
report.setDataSource(createDataSource());
try {
report.show();
}catch(DRException e){
e.printStackTrace();
}
}
private class CCExpression extends AbstractSimpleExpression<Boolean> {
public Boolean evaluate (ReportParameters param){
BigDecimal price = param.getFieldValue("price");
if (price.doubleValue() > 1){
return true;
} else {
return false;
}
}
}
private class CurrencyType extends BigDecimalType {
@Override
public String getPattern() {
return "$ #,###.##";
}
}
private JRDataSource createDataSource() {
DRDataSource dataSource = new DRDataSource("name", "price", "quantityOrdered");
dataSource.add("Apple", new BigDecimal(1.29), 120);
dataSource.add("Apple", new BigDecimal(1.69), 150);
dataSource.add("Orange", new BigDecimal(0.99), 130);
dataSource.add("Orange", new BigDecimal(0.96), 100);
dataSource.add("Mange", new BigDecimal(0), 300);
return dataSource;
}
public static void main(String[] args){
new DynamicReportsTest();
}
}
---------------------------------------------------------------------------------------------------------------------
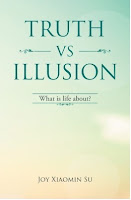
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment