A. Sort by clicking at column header
1. Set all column headers clickable for sortingJTable table = new JTable();
table.setModel(new MyTableModel());
//This will allow sort by clicking column header
table.setAutoCreateRowSorter(true);
//To programmatically reverse the order of sorting of a column
table.getRowSorter().toggleSortOrder(<columnIndex>);
2. Set sorting by a particular column in the beggining
TableRowSorter<MyTableModel> sorter = new TableRowSorter<>(table.getModel());
table.setRowSorter(sorter);
//or
//table.setAutoCreateRowSorter(true);
//DefaultRowSorter sorter = (DefaultRowSorter)table.getRowSorter();
List<RowSorter.SortKey> sortKeys = new ArrayList<>();
sortKeys.add(new RowSorter.SortKey(<columnIndex>, SortOrder.ASCENDING));
sorter.setSortKeys(sortKeys);
public class SortableVector<E> extends Vector<E> implements Comparable<E> {
public SortableVector(List values) {
super(vaules);
}
public int compareTo(Object obj) {
SortableVector<E> r2 = (SortableVector<E>) obj;
Object v1 = get(<columnIndex>);
Object v2 = r2.get(<columnIndex>);
if (v1 <= v2) {
return -1;
}
return 1;
}
}
public class MyTableModel extends DefaultTableModel{
public void sort() {
Vector<SortableVector<Object>> data = getDataVector();
Collections.sort(data);
}
}
MyTableModel model = new MyTableModel();
model.addRow(new SortableVector<>(Arrays.asList(new String[] {"Apple", "East Street"})));
mode.addRow(new SortableVector<>(Arrays.asList(new String[] {"Orange", "Main Ave"})));
mode.addRow(new SortableVector<>(Arrays.asList(new String[] {"Mangle", "5th Street"})));
JTable table = new JTable();
table.setModel(model);
//When data is changed, call the sort() method.
if (table.getCellEditor() != null) {
table.getCellEditor().stopCellEditing();
}
model.sort();
model.fireTableDataChanged();
---------------------------------------------------------------------------------------------------------------
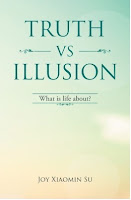
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
sortKeys.add(new RowSorter.SortKey(<columnIndex>, SortOrder.ASCENDING));
sorter.setSortKeys(sortKeys);
B. Sort programmatically on values in one column
public class SortableVector<E> extends Vector<E> implements Comparable<E> {
public SortableVector(List values) {
super(vaules);
}
public int compareTo(Object obj) {
SortableVector<E> r2 = (SortableVector<E>) obj;
Object v1 = get(<columnIndex>);
Object v2 = r2.get(<columnIndex>);
if (v1 <= v2) {
return -1;
}
return 1;
}
}
public class MyTableModel extends DefaultTableModel{
public void sort() {
Vector<SortableVector<Object>> data = getDataVector();
Collections.sort(data);
}
}
MyTableModel model = new MyTableModel();
model.addRow(new SortableVector<>(Arrays.asList(new String[] {"Apple", "East Street"})));
mode.addRow(new SortableVector<>(Arrays.asList(new String[] {"Orange", "Main Ave"})));
mode.addRow(new SortableVector<>(Arrays.asList(new String[] {"Mangle", "5th Street"})));
JTable table = new JTable();
table.setModel(model);
//When data is changed, call the sort() method.
if (table.getCellEditor() != null) {
table.getCellEditor().stopCellEditing();
}
model.sort();
model.fireTableDataChanged();
---------------------------------------------------------------------------------------------------------------
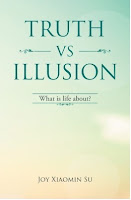
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment