After a container is maximized or stretched to a very large size, the initial font may be too small to look nice in the big container. One way to resize the font according to the size of the container is through a ComponentListener. Below is a sample code.
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.plaf.FontUIResource;
public class PanelFontResize extends JFrame {
private JPanel thePanel;
private JLabel theLabel;
private JTextField theText;
private JTable theTable;
public PanelFontResize() {
theLabel = new JLabel("The Topic: ");
theText = new JTextField("Does the Sun rise the same time each day?");
JPanel northPane = new JPanel(new GridBagLayout());
northPane.add(theLabel, new GridBagConstraints(0,0,1,1,1.0,1.0,GridBagConstraints.EAST, GridBagConstraints.NONE, new Insets(0,0,0,5),0,0));
northPane.add(theText, new GridBagConstraints(1,0,2,1,2.0,1.0,GridBagConstraints.WEST, GridBagConstraints.NONE, new Insets(0,0,0,0),0,0));
String[] colnames = {"Season", "Sun Rise"};
String[][] data = {{"Spring", "6:00"},
{"Summer", "5:30"},
{"Autum", "6:30"},
{"Winter", "7:00"}};
theTable = new JTable(data, colnames);
JScrollPane sp = new JScrollPane(theTable);
thePanel = new JPanel(new BorderLayout());
thePanel.add(northPane, BorderLayout.NORTH);
thePanel.add(sp, BorderLayout.CENTER);
thePanel.addComponentListener(new PanelListener());
setContentPane(thePanel);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 200);
validate();
setVisible(true);
}
private class PanelListener extends ComponentAdapter {
@Override
public void componentResized(ComponentEvent e) {
Dimension psize = thePanel.getSize();
if (psize.width <= 761 && psize.height <= 551) {
setFonts(11);
} else if (psize.width <= 984 && psize.height <= 617
&& psize.width > 761 && psize.height > 551) {
setFonts(13);
} else if (psize.width <= 1046 && psize.height <= 762
&& psize.width > 984 && psize.height > 617) {
setFonts(15);
} else if (psize.width <= 1245 && psize.height <= 867
&& psize.width > 1046 && psize.height > 762) {
setFonts(18);
} else if (psize.width > 1245 && psize.height > 867){
setFonts(20);
}
}
}
public void setFonts(int fontSize) {
Font plainFont = new Font("Dialog", Font.PLAIN, fontSize);
Font boldFont = new Font("Dialog", Font.BOLD, fontSize + 2);
UIManager.put("Text.font", new FontUIResource(plainFont));
UIManager.put("TextField.font", new FontUIResource(plainFont));
UIManager.put("Table.font", new FontUIResource(plainFont));
UIManager.put("Label.font", new FontUIResource(boldFont));
UIManager.put("TableHeader.font", new FontUIResource(boldFont));
UIManager.put("Panel.font", new FontUIResource(plainFont));
UIManager.put("ScrollPane.font", new FontUIResource(plainFont));
setTableSize(fontSize);
SwingUtilities.updateComponentTreeUI(thePanel);
}
private void setTableSize(int fontSize){
Font f = getFont().deriveFont((float)fontSize);
theTable.setRowHeight(new Float(f.getSize2D() * 1.5).intValue());
}
public static void main(String[] args){
new PanelFontResize();
}
}
---------------------------------------------------------------------------------------------------------------
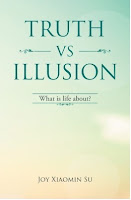
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.plaf.FontUIResource;
public class PanelFontResize extends JFrame {
private JPanel thePanel;
private JLabel theLabel;
private JTextField theText;
private JTable theTable;
public PanelFontResize() {
theLabel = new JLabel("The Topic: ");
theText = new JTextField("Does the Sun rise the same time each day?");
JPanel northPane = new JPanel(new GridBagLayout());
northPane.add(theLabel, new GridBagConstraints(0,0,1,1,1.0,1.0,GridBagConstraints.EAST, GridBagConstraints.NONE, new Insets(0,0,0,5),0,0));
northPane.add(theText, new GridBagConstraints(1,0,2,1,2.0,1.0,GridBagConstraints.WEST, GridBagConstraints.NONE, new Insets(0,0,0,0),0,0));
String[] colnames = {"Season", "Sun Rise"};
String[][] data = {{"Spring", "6:00"},
{"Summer", "5:30"},
{"Autum", "6:30"},
{"Winter", "7:00"}};
theTable = new JTable(data, colnames);
JScrollPane sp = new JScrollPane(theTable);
thePanel = new JPanel(new BorderLayout());
thePanel.add(northPane, BorderLayout.NORTH);
thePanel.add(sp, BorderLayout.CENTER);
thePanel.addComponentListener(new PanelListener());
setContentPane(thePanel);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 200);
validate();
setVisible(true);
}
private class PanelListener extends ComponentAdapter {
@Override
public void componentResized(ComponentEvent e) {
Dimension psize = thePanel.getSize();
if (psize.width <= 761 && psize.height <= 551) {
setFonts(11);
} else if (psize.width <= 984 && psize.height <= 617
&& psize.width > 761 && psize.height > 551) {
setFonts(13);
} else if (psize.width <= 1046 && psize.height <= 762
&& psize.width > 984 && psize.height > 617) {
setFonts(15);
} else if (psize.width <= 1245 && psize.height <= 867
&& psize.width > 1046 && psize.height > 762) {
setFonts(18);
} else if (psize.width > 1245 && psize.height > 867){
setFonts(20);
}
}
}
public void setFonts(int fontSize) {
Font plainFont = new Font("Dialog", Font.PLAIN, fontSize);
Font boldFont = new Font("Dialog", Font.BOLD, fontSize + 2);
UIManager.put("Text.font", new FontUIResource(plainFont));
UIManager.put("TextField.font", new FontUIResource(plainFont));
UIManager.put("Table.font", new FontUIResource(plainFont));
UIManager.put("Label.font", new FontUIResource(boldFont));
UIManager.put("TableHeader.font", new FontUIResource(boldFont));
UIManager.put("Panel.font", new FontUIResource(plainFont));
UIManager.put("ScrollPane.font", new FontUIResource(plainFont));
setTableSize(fontSize);
SwingUtilities.updateComponentTreeUI(thePanel);
}
private void setTableSize(int fontSize){
Font f = getFont().deriveFont((float)fontSize);
theTable.setRowHeight(new Float(f.getSize2D() * 1.5).intValue());
}
public static void main(String[] args){
new PanelFontResize();
}
}
---------------------------------------------------------------------------------------------------------------
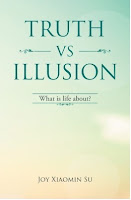
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment