There are several ways to calculate the days between two dates.
public DateTest {
//METHOD 1
private int getDaysBetweetDates(Calendar day1, Calendar day2) {
int days;
Calendar dayOne = (Calendar) day1.clone(),
dayTwo = (Calendar) day2.clone();
if (dayOne.get(Calendar.YEAR) == dayTwo.get(Calendar.YEAR)) {
days = (dayTwo.get(Calendar.DAY_OF_YEAR) - dayOne.get(Calendar.DAY_OF_YEAR));
} else if (dayTwo.get(Calendar.YEAR) > dayOne.get(Calendar.YEAR)) {
int extraDays = 0;
int dayTwoOriginalYearDays = dayTwo.get(Calendar.DAY_OF_YEAR);
while (dayTwo.get(Calendar.YEAR) > dayOne.get(Calendar.YEAR)) {
dayTwo.add(Calendar.YEAR, -1);
// getActualMaximum() important for leap years
extraDays += dayTwo.getActualMaximum(Calendar.DAY_OF_YEAR);
}
days = extraDays + dayTwoOriginalYearDays - dayOne.get(Calendar.DAY_OF_YEAR);
} else {
days = 0;
}
return days;
}
//METHOD 2
private int getDaysBetweenDates2(Calendar day1, Calendar day2){
Calendar dayOne = (Calendar) day1.clone(),
dayTwo = (Calendar) day2.clone();
long diff = dayTwo.getTimeInMillis() - dayOne.getTimeInMillis();
return (diff /(24*60*60*1000));
}
//METHOD 3
private float getDaysBetweenDates3(Calendar day1, Calendar day2){
Calendar dayOne = (Calendar) day1.clone(),
dayTwo = (Calendar) day2.clone();
long diff = dayTwo.getTimeInMillis() - dayOne.getTimeInMillis();
return TimeUnit.DAYS.convert(diff, TimeUnit.MILLISECONDS);
}
public static void main(String[] args){
DateTest test = new DateTest();
long t1 = 1371773105000L;
long t2 = 1372203030000L;
Calendar d1 = Calendar.getInstance();
d1.setTimeInMillis(t1);
System.out.println("Date1: " + d1.get(Calendar.YEAR) + "/" + (d1.get(Calendar.MONTH)+1) + "/" + d1.get(Calendar.DAY_OF_MONTH));
Calendar d2 = Calendar.getInstance();
d2.setTimeInMillis(t2);
System.out.println("Date2: " + d2.get(Calendar.YEAR) + "/" + (d2.get(Calendar.MONTH)+1) + "/" + d2.get(Calendar.DAY_OF_MONTH));
System.out.println("Method1: "+ test.getDaysBetweenDates1(d1,d2));
System.out.println("Method2: "+ test.getDaysBetweenDates2(d1, d2));
System.out.println("Method3: "+ test.getDaysBetweenDates3(d1, d2));
}
The output:
Date1: 2013/6/20
Date2: 2013/6/25
Method1: 5
Method2: 4
Method3: 4
However, if you add the following lines to the beginning of Method 2 and Method 3. For example, the Method 2 will look like this.
private int getDaysBetweenDates2(Calendar day1, Calendar day2){
day1.set(Calendar.HOUR_OF_DAY, 1);
day2.set(Calendar.HOUR_OF_DAY, 1);
day1.set(Calendar.MINUTE, 1);
day2.set(Calendar.MINUTE, 1);
Calendar dayOne = (Calendar) day1.clone(),
dayTwo = (Calendar) day2.clone();
long diff = dayTwo.getTimeInMillis() - dayOne.getTimeInMillis();
return (diff /(24*60*60*1000));
}
The output will become:
Date1: 2013/6/20
Date2: 2013/6/25
Method1: 5
Method2: 5
Method3: 5
---------------------------------------------------------------------------------------------------------------
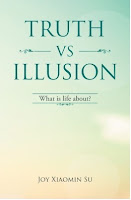
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
public DateTest {
//METHOD 1
private int getDaysBetweetDates(Calendar day1, Calendar day2) {
int days;
Calendar dayOne = (Calendar) day1.clone(),
dayTwo = (Calendar) day2.clone();
if (dayOne.get(Calendar.YEAR) == dayTwo.get(Calendar.YEAR)) {
days = (dayTwo.get(Calendar.DAY_OF_YEAR) - dayOne.get(Calendar.DAY_OF_YEAR));
} else if (dayTwo.get(Calendar.YEAR) > dayOne.get(Calendar.YEAR)) {
int extraDays = 0;
int dayTwoOriginalYearDays = dayTwo.get(Calendar.DAY_OF_YEAR);
while (dayTwo.get(Calendar.YEAR) > dayOne.get(Calendar.YEAR)) {
dayTwo.add(Calendar.YEAR, -1);
// getActualMaximum() important for leap years
extraDays += dayTwo.getActualMaximum(Calendar.DAY_OF_YEAR);
}
days = extraDays + dayTwoOriginalYearDays - dayOne.get(Calendar.DAY_OF_YEAR);
} else {
days = 0;
}
return days;
}
//METHOD 2
private int getDaysBetweenDates2(Calendar day1, Calendar day2){
Calendar dayOne = (Calendar) day1.clone(),
dayTwo = (Calendar) day2.clone();
long diff = dayTwo.getTimeInMillis() - dayOne.getTimeInMillis();
return (diff /(24*60*60*1000));
}
//METHOD 3
private float getDaysBetweenDates3(Calendar day1, Calendar day2){
Calendar dayOne = (Calendar) day1.clone(),
dayTwo = (Calendar) day2.clone();
long diff = dayTwo.getTimeInMillis() - dayOne.getTimeInMillis();
return TimeUnit.DAYS.convert(diff, TimeUnit.MILLISECONDS);
}
public static void main(String[] args){
DateTest test = new DateTest();
long t1 = 1371773105000L;
long t2 = 1372203030000L;
Calendar d1 = Calendar.getInstance();
d1.setTimeInMillis(t1);
System.out.println("Date1: " + d1.get(Calendar.YEAR) + "/" + (d1.get(Calendar.MONTH)+1) + "/" + d1.get(Calendar.DAY_OF_MONTH));
Calendar d2 = Calendar.getInstance();
d2.setTimeInMillis(t2);
System.out.println("Date2: " + d2.get(Calendar.YEAR) + "/" + (d2.get(Calendar.MONTH)+1) + "/" + d2.get(Calendar.DAY_OF_MONTH));
System.out.println("Method1: "+ test.getDaysBetweenDates1(d1,d2));
System.out.println("Method2: "+ test.getDaysBetweenDates2(d1, d2));
System.out.println("Method3: "+ test.getDaysBetweenDates3(d1, d2));
}
The output:
Date1: 2013/6/20
Date2: 2013/6/25
Method1: 5
Method2: 4
Method3: 4
However, if you add the following lines to the beginning of Method 2 and Method 3. For example, the Method 2 will look like this.
private int getDaysBetweenDates2(Calendar day1, Calendar day2){
day1.set(Calendar.HOUR_OF_DAY, 1);
day2.set(Calendar.HOUR_OF_DAY, 1);
day1.set(Calendar.MINUTE, 1);
day2.set(Calendar.MINUTE, 1);
Calendar dayOne = (Calendar) day1.clone(),
dayTwo = (Calendar) day2.clone();
long diff = dayTwo.getTimeInMillis() - dayOne.getTimeInMillis();
return (diff /(24*60*60*1000));
}
The output will become:
Date1: 2013/6/20
Date2: 2013/6/25
Method1: 5
Method2: 5
Method3: 5
---------------------------------------------------------------------------------------------------------------
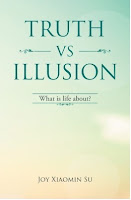
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment