The builder pattern is used to construct a complex object by making its components one by one.
For example if you would like to build a car, you need to build the frame, the engine, the wheels, the doors, etc.
String name;
float price;
}
public class Frame implements Part {
public String getName() {
return "Frame";
}
public float getPrice() {
return 2000;
}
}
public class Engine implements Part {
public String getName() {
return "Engine";
}
public float getPrice() {
return 6000;
}
}
private float cost = 0;
public void addPart(Part part) {
cost += part.getPrice();
System.out.println(part.getName() + " built on the car");
}
public void getCost() {
return (50000 + cost);
}
}
private Car theCar;
public Car buildCar() {
theCar = new Car();
theCar.addPart(new Frame());
theCar.addPart(new Engine());
System.out.println("The cost for building this car is " + theCar.getCost());
}
}
public static void main(String[] args) {
CarBuilder builder = new CarBuilder();
builder.buildCar();
}
}
---------------------------------------------------------------------------------------------------------------
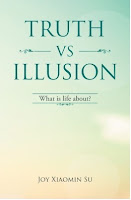
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
For example if you would like to build a car, you need to build the frame, the engine, the wheels, the doors, etc.
//The components or parts of a car
public interface Part {String name;
float price;
}
public class Frame implements Part {
public String getName() {
return "Frame";
}
public float getPrice() {
return 2000;
}
}
public class Engine implements Part {
public String getName() {
return "Engine";
}
public float getPrice() {
return 6000;
}
}
//The car
public class Car {private float cost = 0;
public void addPart(Part part) {
cost += part.getPrice();
System.out.println(part.getName() + " built on the car");
}
public void getCost() {
return (50000 + cost);
}
}
//The car builder
public class CarBuilder {private Car theCar;
public Car buildCar() {
theCar = new Car();
theCar.addPart(new Frame());
theCar.addPart(new Engine());
System.out.println("The cost for building this car is " + theCar.getCost());
}
}
//The Runner
public class CarBuilderDemo {public static void main(String[] args) {
CarBuilder builder = new CarBuilder();
builder.buildCar();
}
}
---------------------------------------------------------------------------------------------------------------
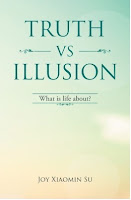
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment