JTable by default uses a JTextField to render and editor a String field. In JTable, use the setColumns method in the JTextField does not do the job to restrict the length of the field. One way to restrict the size of the field and characters/strings the field can take is to override the insertString method in the Document that is used as the model of the JTextField. Another way is to check the field when the field is stopped editing or a Submit button is clicked.
import java.util.Arrays;
import javax.swing.DefaultCellEditor;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.table.DefaultTableModel;
import javax.swing.text.AttributeSet;
import javax.swing.text.BadLocationException;
import javax.swing.text.PlainDocument;
public class TableTest extends JFrame{
private JTable table;
private DefaultTableModel model;
private JTextField charField;
private ArrayList<String> notAllowedStrings;
public TableTest() {
model = new DefaultTableModel(new String[]{"Name", "Char"}, 2);
table = new JTable();
table.setModel(model);
notAllowedStrings = new ArrayList<String>(Arrays.asList(new String[]{"A", "B", "C", "D"}));
charField = new JTextField();
MyDocument document = new MyDocument();
document.setMaxSize(1);
charField.setDocument(document);
table.getColumn("Char").setCellEditor(new DefaultCellEditor(charField));
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setSize(300, 200);
setVisible(true);
}
class MyDocument extends PlainDocument{
private int maxSize = 10;
public void insertString(int offs, String str, AttributeSet a)
throws BadLocationException {
if (str == null) return;
int length = str.length();
if (length <= 0) return;
if ((offs + length) > maxSize ){
str = "";
} else if (notAllowedStrings.contains(str.toUpperCase())){
str = "";
}
super.insertString(offs, str.toUpperCase(), a);
}
public void setMaxSize(int s){
maxSize = s;
}
}
public static void main(String[] args){
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TableTest();
}
});
}
}
import java.util.ArrayList;
import java.util.Arrays;
import javax.swing.DefaultCellEditor;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.event.CellEditorListener;
import javax.swing.event.ChangeEvent;
import javax.swing.table.DefaultTableModel;
public class TableTest extends JFrame{
private JTable table;
private JButton submitButton;
private DefaultTableModel model;
private JTextField charField;
private ArrayList<String> notAllowedStrings;
private int maxSize = 1;
public TableTest() {
model = new DefaultTableModel(new String[]{"Name", "Char"}, 2);
table = new JTable();//{
table.setModel(model);
table.getColumnModel().getColumn(1).setCellEditor(new MyCellEditor(new JTextField()));
notAllowedStrings = new ArrayList<String>(Arrays.asList(new String[]{"A", "B", "C", "D"}));
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setSize(300, 200);
setVisible(true);
}
class MyCellEditor extends DefaultCellEditor implements CellEditorListener{
Object value;
int row;
int col;
public MyCellEditor(JTextField t){
super(t);
this.setClickCountToStart(1);
addCellEditorListener(this);
}
public Component getTableCellEditorComponent(JTable t, Object v, boolean seleted, int r, int c){
row = r;
col = c;
Component com = super.getTableCellEditorComponent(t, v, seleted, r, c);
return com;
}
public void editingStopped(ChangeEvent e) {
System.out.println(row+"; "+col+"; "+value+";"+table.getValueAt(row, col)+";");
if (col == 1) {
value = table.getValueAt(row, col);
if (value != null && !value.toString().isEmpty()) {
if (notAllowedStrings.contains(value.toString().toUpperCase())
|| value.toString().length() > maxSize) {
JOptionPane.showMessageDialog(TableTest.this,
"The Char value in row " + row + " is not valid",
"Error",
JOptionPane.ERROR_MESSAGE);
table.setValueAt("", row, col);
return;
}
}
}
}
public void editingCanceled(ChangeEvent e){
System.out.println("Editing canceled");
}
}
public static void main(String[] args){
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TableTest();
}
});
}
}
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Arrays;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.table.DefaultTableModel;
public class TableTest extends JFrame{
private JTable table;
private JButton submitButton;
private DefaultTableModel model;
private JTextField charField;
private ArrayList<String> notAllowedStrings;
private int maxSize = 1;
public TableTest() {
model = new DefaultTableModel(new String[]{"Name", "Char"}, 2);
table = new JTable();
table.setModel(model);
notAllowedStrings = new ArrayList<String>(Arrays.asList(new String[]{"A", "B", "C", "D"}));
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane);
submitButton = new JButton("Submit");
add(submitButton, BorderLayout.SOUTH);
submitButton.addActionListener(new MyActionListener());
setDefaultCloseOperation(EXIT_ON_CLOSE);
setSize(300, 200);
setVisible(true);
}
class MyActionListener implements ActionListener {
public void actionPerformed(ActionEvent e){
table.getCellEditor().stopCellEditing();
for (int i=0; i<table.getRowCount(); i++){
Object v = table.getValueAt(i, 1);
if (v == null) continue;
if (notAllowedStrings.contains(v.toString().toUpperCase()) ||
v.toString().length()> maxSize){
JOptionPane.showMessageDialog(TableTest.this,
"The Char value in row "+i+" is not valid",
"Error",
JOptionPane.ERROR_MESSAGE);
return;
}
}
}
}
public static void main(String[] args){
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TableTest();
}
});
}
}
----------------------------------------------------------------------------------------------------------------
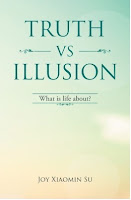
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
1. Override the insertString method in Document. The following sample code shows how to restrict the "Char" column of the table to size 1 and exclude the field from taking any of these characters in either upper or lower case: 'A', 'B', 'C', and 'D'.
import java.util.ArrayList;import java.util.Arrays;
import javax.swing.DefaultCellEditor;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.table.DefaultTableModel;
import javax.swing.text.AttributeSet;
import javax.swing.text.BadLocationException;
import javax.swing.text.PlainDocument;
public class TableTest extends JFrame{
private JTable table;
private DefaultTableModel model;
private JTextField charField;
private ArrayList<String> notAllowedStrings;
public TableTest() {
model = new DefaultTableModel(new String[]{"Name", "Char"}, 2);
table = new JTable();
table.setModel(model);
notAllowedStrings = new ArrayList<String>(Arrays.asList(new String[]{"A", "B", "C", "D"}));
charField = new JTextField();
MyDocument document = new MyDocument();
document.setMaxSize(1);
charField.setDocument(document);
table.getColumn("Char").setCellEditor(new DefaultCellEditor(charField));
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setSize(300, 200);
setVisible(true);
}
class MyDocument extends PlainDocument{
private int maxSize = 10;
public void insertString(int offs, String str, AttributeSet a)
throws BadLocationException {
if (str == null) return;
int length = str.length();
if (length <= 0) return;
if ((offs + length) > maxSize ){
str = "";
} else if (notAllowedStrings.contains(str.toUpperCase())){
str = "";
}
super.insertString(offs, str.toUpperCase(), a);
}
public void setMaxSize(int s){
maxSize = s;
}
}
public static void main(String[] args){
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TableTest();
}
});
}
}
2. Add a CellEditorListener to the cell editor
import java.awt.Component;import java.util.ArrayList;
import java.util.Arrays;
import javax.swing.DefaultCellEditor;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.event.CellEditorListener;
import javax.swing.event.ChangeEvent;
import javax.swing.table.DefaultTableModel;
public class TableTest extends JFrame{
private JTable table;
private JButton submitButton;
private DefaultTableModel model;
private JTextField charField;
private ArrayList<String> notAllowedStrings;
private int maxSize = 1;
public TableTest() {
model = new DefaultTableModel(new String[]{"Name", "Char"}, 2);
table = new JTable();//{
table.setModel(model);
table.getColumnModel().getColumn(1).setCellEditor(new MyCellEditor(new JTextField()));
notAllowedStrings = new ArrayList<String>(Arrays.asList(new String[]{"A", "B", "C", "D"}));
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setSize(300, 200);
setVisible(true);
}
class MyCellEditor extends DefaultCellEditor implements CellEditorListener{
Object value;
int row;
int col;
public MyCellEditor(JTextField t){
super(t);
this.setClickCountToStart(1);
addCellEditorListener(this);
}
public Component getTableCellEditorComponent(JTable t, Object v, boolean seleted, int r, int c){
row = r;
col = c;
Component com = super.getTableCellEditorComponent(t, v, seleted, r, c);
return com;
}
public void editingStopped(ChangeEvent e) {
System.out.println(row+"; "+col+"; "+value+";"+table.getValueAt(row, col)+";");
if (col == 1) {
value = table.getValueAt(row, col);
if (value != null && !value.toString().isEmpty()) {
if (notAllowedStrings.contains(value.toString().toUpperCase())
|| value.toString().length() > maxSize) {
JOptionPane.showMessageDialog(TableTest.this,
"The Char value in row " + row + " is not valid",
"Error",
JOptionPane.ERROR_MESSAGE);
table.setValueAt("", row, col);
return;
}
}
}
}
public void editingCanceled(ChangeEvent e){
System.out.println("Editing canceled");
}
}
public static void main(String[] args){
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TableTest();
}
});
}
}
3. Check field when Submit button is pressed
import java.awt.BorderLayout;import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Arrays;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.table.DefaultTableModel;
public class TableTest extends JFrame{
private JTable table;
private JButton submitButton;
private DefaultTableModel model;
private JTextField charField;
private ArrayList<String> notAllowedStrings;
private int maxSize = 1;
public TableTest() {
model = new DefaultTableModel(new String[]{"Name", "Char"}, 2);
table = new JTable();
table.setModel(model);
notAllowedStrings = new ArrayList<String>(Arrays.asList(new String[]{"A", "B", "C", "D"}));
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane);
submitButton = new JButton("Submit");
add(submitButton, BorderLayout.SOUTH);
submitButton.addActionListener(new MyActionListener());
setDefaultCloseOperation(EXIT_ON_CLOSE);
setSize(300, 200);
setVisible(true);
}
class MyActionListener implements ActionListener {
public void actionPerformed(ActionEvent e){
table.getCellEditor().stopCellEditing();
for (int i=0; i<table.getRowCount(); i++){
Object v = table.getValueAt(i, 1);
if (v == null) continue;
if (notAllowedStrings.contains(v.toString().toUpperCase()) ||
v.toString().length()> maxSize){
JOptionPane.showMessageDialog(TableTest.this,
"The Char value in row "+i+" is not valid",
"Error",
JOptionPane.ERROR_MESSAGE);
return;
}
}
}
}
public static void main(String[] args){
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TableTest();
}
});
}
}
----------------------------------------------------------------------------------------------------------------
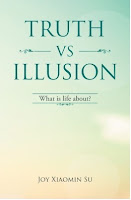
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment