It can be frustrating that some times the TableModelListener is fired and other times it is not when you click some where outside the currently editing cell. After you just entered a new value into a table cell, you immediately click the Save button, and surprisingly the new value you just entered was not saved, and you wonder why. The table model takes the modified value from the JTable only at the moment the cell stopped editing, and that is when the tableChanged method in the TableModelListener is called. Therefore, the code needs to specifically call the stopCellEditing method when you are performing other actions instead of selecting another cell in the JTable. In the above example, after you typed a string in one of the table cells and then click the Save button, you need to deliberately call the stopCellEditing method for the TableModelListener to work.
In the following sample code, you can notice the difference. If you comment out the line table.getCellEditor().stopCellEditing(); The pop-up window in the TableModelListener will not show.
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.event.TableModelEvent;
import javax.swing.event.TableModelListener;
import javax.swing.table.DefaultTableModel;
public class TestCode extends JFrame {
DefaultTableModel model = new DefaultTableModel();
JTable table = new JTable();
public TestCode() {
model.setColumnIdentifiers(new String[] {"Name", "Value"});
model.addRow(new String[] {"Rose", ""});
model.addRow(new String[] {"Peony", ""});
model.addTableModelListener(new MyModelListener());
table.setModel(model);
JScrollPane jsp = new JScrollPane(table);
add(jsp);
JButton save = new JButton("Save");
save.addActionListener(new MyActionListener());
add(save, BorderLayout.SOUTH);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300,400);
setVisible(true);
}
private class MyModelListener implements TableModelListener {
public void tableChanged(TableModelEvent e){
JOptionPane.showMessageDialog(TestCode.this, "Table has been modified");
}
}
private class MyActionListener implements ActionListener {
public void actionPerformed(ActionEvent e){
table.getCellEditor().stopCellEditing();
System.out.println("Changed saved!!!!");
}
}
public static void main(String[] args){
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TestCode();
}
});
}
}
-------------------------------------------------------------------------------------------------------------
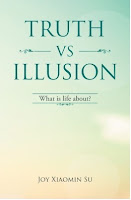
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
In the following sample code, you can notice the difference. If you comment out the line table.getCellEditor().stopCellEditing(); The pop-up window in the TableModelListener will not show.
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.event.TableModelEvent;
import javax.swing.event.TableModelListener;
import javax.swing.table.DefaultTableModel;
public class TestCode extends JFrame {
DefaultTableModel model = new DefaultTableModel();
JTable table = new JTable();
public TestCode() {
model.setColumnIdentifiers(new String[] {"Name", "Value"});
model.addRow(new String[] {"Rose", ""});
model.addRow(new String[] {"Peony", ""});
model.addTableModelListener(new MyModelListener());
table.setModel(model);
JScrollPane jsp = new JScrollPane(table);
add(jsp);
JButton save = new JButton("Save");
save.addActionListener(new MyActionListener());
add(save, BorderLayout.SOUTH);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300,400);
setVisible(true);
}
private class MyModelListener implements TableModelListener {
public void tableChanged(TableModelEvent e){
JOptionPane.showMessageDialog(TestCode.this, "Table has been modified");
}
}
private class MyActionListener implements ActionListener {
public void actionPerformed(ActionEvent e){
table.getCellEditor().stopCellEditing();
System.out.println("Changed saved!!!!");
}
}
public static void main(String[] args){
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TestCode();
}
});
}
}
-------------------------------------------------------------------------------------------------------------
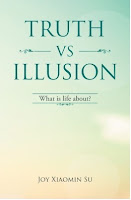
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
it works!
ReplyDeletethanks a lot