In java, a long value has 64 bits and it is a signed value ranging from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 inclusively. An int value has 32 bits and it is also a signed value ranging from -2,147,483,648 to 2,147,483,647 inclusively.
To convert a long value that is in the range of -2,147,483,648 to 2,147,483,647, you can directly cast it to int.
long a = 33444556L;
int b = (int)a;
However, if the long value is out of the -2,147,483,648 to 2,147,483,647 range, casting returns -1.
Here is a sample code for testing the conversion.
public class LongToIntegerTest {
public static void main(String[] args){
long x1 = 2147483647L;
int y1 = (int)x1;
Long x12 = new Long(x1);
int y12 = x12.intValue();
long x2 = 9223372036854775807L;
int y2 = (int)x2;
Long x22 = new Long(x2);
int y22 = x22.intValue();
System.out.println("y1 = "+y1);
System.out.println("y12 = "+y12);
System.out.println("y2 = "+y2);
System.out.println("y22 = "+y22);
}
}
Output:
y1 = 2147483647
y12 = 2147483647
y2 = -1
y22 = -1
-----------------------------------------------------------------------------------------------------------------------
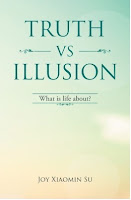
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
To convert a long value that is in the range of -2,147,483,648 to 2,147,483,647, you can directly cast it to int.
long a = 33444556L;
int b = (int)a;
However, if the long value is out of the -2,147,483,648 to 2,147,483,647 range, casting returns -1.
Here is a sample code for testing the conversion.
public class LongToIntegerTest {
public static void main(String[] args){
long x1 = 2147483647L;
int y1 = (int)x1;
Long x12 = new Long(x1);
int y12 = x12.intValue();
long x2 = 9223372036854775807L;
int y2 = (int)x2;
Long x22 = new Long(x2);
int y22 = x22.intValue();
System.out.println("y1 = "+y1);
System.out.println("y12 = "+y12);
System.out.println("y2 = "+y2);
System.out.println("y22 = "+y22);
}
}
Output:
y1 = 2147483647
y12 = 2147483647
y2 = -1
y22 = -1
-----------------------------------------------------------------------------------------------------------------------
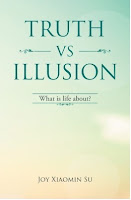
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment