Enum in Java is a special class type which serves as a data structure that holds a collection of objects having same structures. The data in an enum is static and final, they cannot be modified. Enum has a default constructor that takes no arguments.
For example:
public enum Month {
JANUARY, FEBRUARY, MARCH, APRIL, MAY, JUNE,
JULY, AUGUST, SEPTEMBER, OCTOBER, NOVEMBER, DECEMBER
}
is a simple enum defines the type of Month. Each element in the enum is called an enum constant. The enum can be used as shown below.
public class EnumTest {
public static void main(String[] args){
Month theMonth = Month.DECEMBER;
System.out.println(theMonth);
System.out.println(Month.valueOf("DECEMBER"));
//The ordinal method returns the position of an enum constant in the enum.
//The position of the first enum constant is zero.
System.out.println(Month.MARCH.ordinal());
Month[] months = Month.values();
for (Month month : months) {
System.out.println(month);
}
}
}
The output of the above code:
DECEMBER
DECEMBER
2
JANUARY
FEBRUARY
MARCH
APRIL
MAY
JUNE
JULY
AUGUST
SEPTEMBER
OCTOBER
NOVEMBER
DECEMBER
Each enum constant can have its own values. In the above Month enum, each month has the number of days and number of weekends in it. To have these values in the Month enum, you must create a constructor for the enum that matches the declaration of your enum constants.
public enum Month {
JANUARY(31,5),
FEBRUARY(28,4),
MARCH(31,5),
APRIL(30,4),
MAY(31,5),
. . . . . .
DECEMBER(31,4);
//The fields for the values
private final int days;
private final int weekends;
//Create a constructor that matches the type
Month(int days, int weekends) {
this.days = days;
this.weekends = weekends;
}
//Enums can also have methods
public int getDays() {return days;}
public int getWeekends() {return weekends;}
public int getWorkingDays() {
return days-weekends;
}
}
public class EnumTest {
public static void main(String[] args){
Month theMonth = Month.FEBRUARY;
System.out.println("Working days in February="+theMonth.getWorkingDays());
}
}
The output is:
Working days in February=24
-------------------------------------------------------------------------------------------------------------
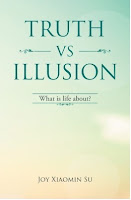
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
For example:
public enum Month {
JANUARY, FEBRUARY, MARCH, APRIL, MAY, JUNE,
JULY, AUGUST, SEPTEMBER, OCTOBER, NOVEMBER, DECEMBER
}
is a simple enum defines the type of Month. Each element in the enum is called an enum constant. The enum can be used as shown below.
public class EnumTest {
public static void main(String[] args){
Month theMonth = Month.DECEMBER;
System.out.println(theMonth);
System.out.println(Month.valueOf("DECEMBER"));
//The ordinal method returns the position of an enum constant in the enum.
//The position of the first enum constant is zero.
System.out.println(Month.MARCH.ordinal());
Month[] months = Month.values();
for (Month month : months) {
System.out.println(month);
}
}
}
The output of the above code:
DECEMBER
DECEMBER
2
JANUARY
FEBRUARY
MARCH
APRIL
MAY
JUNE
JULY
AUGUST
SEPTEMBER
OCTOBER
NOVEMBER
DECEMBER
Each enum constant can have its own values. In the above Month enum, each month has the number of days and number of weekends in it. To have these values in the Month enum, you must create a constructor for the enum that matches the declaration of your enum constants.
public enum Month {
JANUARY(31,5),
FEBRUARY(28,4),
MARCH(31,5),
APRIL(30,4),
MAY(31,5),
. . . . . .
DECEMBER(31,4);
//The fields for the values
private final int days;
private final int weekends;
//Create a constructor that matches the type
Month(int days, int weekends) {
this.days = days;
this.weekends = weekends;
}
//Enums can also have methods
public int getDays() {return days;}
public int getWeekends() {return weekends;}
public int getWorkingDays() {
return days-weekends;
}
}
public class EnumTest {
public static void main(String[] args){
Month theMonth = Month.FEBRUARY;
System.out.println("Working days in February="+theMonth.getWorkingDays());
}
}
The output is:
Working days in February=24
-------------------------------------------------------------------------------------------------------------
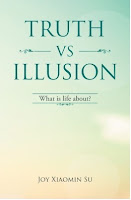
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment