DynamicReports, based on JasperReports, is a tool to view the data in your data source which can be your database connected through JDBC, dynamically generated data, or other source. The report can be viewed in JasperReports Viewer and be written to your hard drive as PDF, HTML, XML, DOCX and other types of files. The report can be formulated in the form of a table, one of the many styles of charts, or other styles. Following are the steps to generate a simple tabular report using a table in your database as the data source.
1. Download DynamicReports from its official website and add it to your classpath or project library. You need the jars in the dist and the lib directories.
2. Create and run the code. Following is a sample code.
import java.io.FileOutputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.Statement;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import net.sf.dynamicreports.report.builder.DynamicReports;
import net.sf.dynamicreports.report.builder.column.Columns;
import net.sf.dynamicreports.report.builder.component.Components;
import net.sf.dynamicreports.report.builder.datatype.DataTypes;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
public class Test {
String url = "<your JDBC url>";
String driver = "<your JDBC driver>";
String user = "<your username>";
String passwd = "<your password>";
public Test() {
build();
}
public void build() {
Connection conn = null;
try {
conn = getConnection();
//Creating the report
JasperReportBuilder report = DynamicReports.report();
report.setPageFormat(PageType.LETTER);
//Creating the columns
//Here "Price" is the column name on report
//"price" is the data source column name
TextColumnBuilder<Float> priceColumn = Columns.column("Price", "price", DataTypes.floatType());
TextColumnBuilder<Integer> quantityOrderedColumn = Columns.column("Quantity Ordered", "quantityOrdered", DataTypes.integerType());
report.columns(Columns.columnRowNumberColumn("Item"),
Columns.column("Name", "name", DataTypes.stringType()),
priceColumn,
quantityOrderedColumn
);
//Styles
StyleBuilder bold = Styles.style().bold();
StyleBuilder centeredBold = Styles.style(bold)
.setHorizontalAlignment(HorizontalAlignment.CENTER);
StyleBuilder columnStyle = Styles.style(centeredBold)
.setBackgroundColor(Color.LIGHT_GRAY)
.setBorder(Styles.pen1Point());
StyleBuilder titleStyle = Styles.style(centeredBold)
.setVerticalAlignment(VerticalAlignment.MIDDLE)
.setFontSize(15);
report.setColumnTitleStyle(columnStyle);
report.setColumnStyle(Styles.style().setHorizontalAlignment(HorizontalAlignment.CENTER));
report.highlightDetailEvenRows();
//Additional columns to hold values derived by caculation
TextColumnBuilder<BigDecimal> moneyPaidColumn = priceColumn.multiply(quantityOrderedColumn)
.setTitle("Amount Paid");
PercentageColumnBuilder percentPayment = Columns.percentageColumn("Payment %", moneyPaidColumn);
report.addColumn(moneyPaidColumn);
report.addColumn(percentPayment);
//Setting the title of the report
report.title(Components.text("Test Report")
.setStyle(titleStyle));
report.title(Components.currentDate()
.setHorizontalAlignment(HorizontalAlignment.LEFT));
//Printing the number of pages at the footer
report.pageFooter(Components.pageXofY()
.setStyle(centeredBold));
//Setting the data source
report.setDataSource(createDataSource());
//Or using data from database
//String sql = "select name, price, quantityOrdered from DIAMOND";
//report.setDataSource(sql, conn);
//Showing as a JasperReport
report.show();
//Writing to the hard drive as a pdf file
java.io.File file = new java.io.File("C:\\MyReport.pdf");
report.toPdf(new FileOutputStream(file));
//Or write as a html file
// java.io.File file = new java.io.File("C:\\MyReport.html");
// report.toHtml(new FileOutputStream(file));
conn.close();
}catch (Exception e){
e.printStackTrace();
}
}
public Connection getConnection() throws Exception {
Connection conn = null;
Class.forName(driver);
conn = DriverManager.getConnection(url, user, passwd);
return conn;
}
private JRDataSource createDataSource() {
DRDataSource dataSource = new DRDataSource("name", "price", "quantityOrdered");
dataSource.add("Apple", 1.29f, 120);
dataSource.add("Apple", 1.69f, 150);
dataSource.add("Orange", 0.99f, 130);
dataSource.add("Orange", 0.96f, 100);
dataSource.add("Mange", 2.99f, 300);
return dataSource;
}
public static void main(String[] args) {
new Test();
}
}
>Next
----------------------------------------------------------------------------------------------------------------
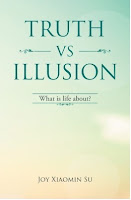
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
-------------------------------------------------------------------------------------------------------------------------
2. DynamicReports in java (3) - Concatenating reports
3. DynamicReports in java (4) - Setting/formatting the title
4. DynamicReports in java (5) - subreport and page break
5. Getting started
6. Reporting In Java Using DynamicReports And JasperReports
7. Report bands
1. Download DynamicReports from its official website and add it to your classpath or project library. You need the jars in the dist and the lib directories.
2. Create and run the code. Following is a sample code.
import java.io.FileOutputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.Statement;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import net.sf.dynamicreports.report.builder.DynamicReports;
import net.sf.dynamicreports.report.builder.column.Columns;
import net.sf.dynamicreports.report.builder.component.Components;
import net.sf.dynamicreports.report.builder.datatype.DataTypes;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
public class Test {
String url = "<your JDBC url>";
String driver = "<your JDBC driver>";
String user = "<your username>";
String passwd = "<your password>";
public Test() {
build();
}
public void build() {
Connection conn = null;
try {
conn = getConnection();
//Creating the report
JasperReportBuilder report = DynamicReports.report();
report.setPageFormat(PageType.LETTER);
//Creating the columns
//Here "Price" is the column name on report
//"price" is the data source column name
TextColumnBuilder<Float> priceColumn = Columns.column("Price", "price", DataTypes.floatType());
TextColumnBuilder<Integer> quantityOrderedColumn = Columns.column("Quantity Ordered", "quantityOrdered", DataTypes.integerType());
report.columns(Columns.columnRowNumberColumn("Item"),
Columns.column("Name", "name", DataTypes.stringType()),
priceColumn,
quantityOrderedColumn
);
//Styles
StyleBuilder bold = Styles.style().bold();
StyleBuilder centeredBold = Styles.style(bold)
.setHorizontalAlignment(HorizontalAlignment.CENTER);
StyleBuilder columnStyle = Styles.style(centeredBold)
.setBackgroundColor(Color.LIGHT_GRAY)
.setBorder(Styles.pen1Point());
StyleBuilder titleStyle = Styles.style(centeredBold)
.setVerticalAlignment(VerticalAlignment.MIDDLE)
.setFontSize(15);
report.setColumnTitleStyle(columnStyle);
report.setColumnStyle(Styles.style().setHorizontalAlignment(HorizontalAlignment.CENTER));
report.highlightDetailEvenRows();
//Additional columns to hold values derived by caculation
TextColumnBuilder<BigDecimal> moneyPaidColumn = priceColumn.multiply(quantityOrderedColumn)
.setTitle("Amount Paid");
PercentageColumnBuilder percentPayment = Columns.percentageColumn("Payment %", moneyPaidColumn);
report.addColumn(moneyPaidColumn);
report.addColumn(percentPayment);
//Setting the title of the report
report.title(Components.text("Test Report")
.setStyle(titleStyle));
report.title(Components.currentDate()
.setHorizontalAlignment(HorizontalAlignment.LEFT));
//Printing the number of pages at the footer
report.pageFooter(Components.pageXofY()
.setStyle(centeredBold));
//Setting the data source
report.setDataSource(createDataSource());
//Or using data from database
//String sql = "select name, price, quantityOrdered from DIAMOND";
//report.setDataSource(sql, conn);
//Showing as a JasperReport
report.show();
//Writing to the hard drive as a pdf file
java.io.File file = new java.io.File("C:\\MyReport.pdf");
report.toPdf(new FileOutputStream(file));
//Or write as a html file
// java.io.File file = new java.io.File("C:\\MyReport.html");
// report.toHtml(new FileOutputStream(file));
conn.close();
}catch (Exception e){
e.printStackTrace();
}
}
public Connection getConnection() throws Exception {
Connection conn = null;
Class.forName(driver);
conn = DriverManager.getConnection(url, user, passwd);
return conn;
}
private JRDataSource createDataSource() {
DRDataSource dataSource = new DRDataSource("name", "price", "quantityOrdered");
dataSource.add("Apple", 1.29f, 120);
dataSource.add("Apple", 1.69f, 150);
dataSource.add("Orange", 0.99f, 130);
dataSource.add("Orange", 0.96f, 100);
dataSource.add("Mange", 2.99f, 300);
return dataSource;
}
public static void main(String[] args) {
new Test();
}
}
>Next
----------------------------------------------------------------------------------------------------------------
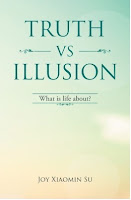
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
-------------------------------------------------------------------------------------------------------------------------
References:
1.DynamicReports in Java (2) - Using adhoc data types2. DynamicReports in java (3) - Concatenating reports
3. DynamicReports in java (4) - Setting/formatting the title
4. DynamicReports in java (5) - subreport and page break
5. Getting started
6. Reporting In Java Using DynamicReports And JasperReports
7. Report bands
how to find my JDBC url and driver????
ReplyDeleteThe best way is to ask your database administrator.
ReplyDeleteIf you use Oracle database, the following link maybe helpful.
http://razorsql.com/docs/help_oracle.html
If you use MySQL, please visit the following website.
http://razorsql.com/docs/help_sqlserver.html