To add a Video to your JavaFX screen, first create a Media using the video file, then create a MediaPlayer using the Media, and create a MeidaView using the created MediaPlayer or set the MediaPlayer of your MediaView to the created MediaPlayer. Finally add the MediaView to your scene.
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.Pane;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.media.MediaView;
import javafx.stage.Stage;
public class VedioTest extends Application {
@Override
public void start(Stage primaryStage) {
//Creating the Media
//Either use absolute path of the audio clip or it should be in the same place
//where the Class used to load it is located
//Here the Kalimba.mp3 should be in the same directory as the VedioTest
Media media = new Media(VedioTest.class.getResource("TestVideo.mp4").toString());
//Creating the MediaPlayer
final MediaPlayer mediaPlayer = new MediaPlayer(media);
// Make the video automatically play forever
// mediaPlayer.setAutoPlay(true);
// mediaPlayer.setCycleCount(MediaPlayer.INDEFINITE);
//Creating the MediaView
MediaView mediaView = new MediaView(mediaPlayer);
Button btn = new Button();
btn.setText("Play Video");
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
//Call one of the methods of MediaPlayer such as
//play(), pause(), and stop()
mediaPlayer.play();
}
});
//Add the MediaView to the scene
Pane root = new Pane();
btn.relocate(50, 200);
root.getChildren().addAll(mediaView, btn);
// root.getChildren().add(btn);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Video!");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
----------------------------------------------------------------------------------------------------------
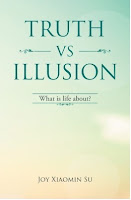
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.Pane;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.media.MediaView;
import javafx.stage.Stage;
public class VedioTest extends Application {
@Override
public void start(Stage primaryStage) {
//Creating the Media
//Either use absolute path of the audio clip or it should be in the same place
//where the Class used to load it is located
//Here the Kalimba.mp3 should be in the same directory as the VedioTest
Media media = new Media(VedioTest.class.getResource("TestVideo.mp4").toString());
//Creating the MediaPlayer
final MediaPlayer mediaPlayer = new MediaPlayer(media);
// Make the video automatically play forever
// mediaPlayer.setAutoPlay(true);
// mediaPlayer.setCycleCount(MediaPlayer.INDEFINITE);
//Creating the MediaView
MediaView mediaView = new MediaView(mediaPlayer);
Button btn = new Button();
btn.setText("Play Video");
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
//Call one of the methods of MediaPlayer such as
//play(), pause(), and stop()
mediaPlayer.play();
}
});
//Add the MediaView to the scene
Pane root = new Pane();
btn.relocate(50, 200);
root.getChildren().addAll(mediaView, btn);
// root.getChildren().add(btn);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Video!");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
----------------------------------------------------------------------------------------------------------
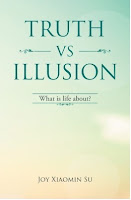
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment