In java 8, the List interface has a new method, stream(), which returns a Stream object. The Stream interface has a collection of methods, such as filter(), sorted(), map(), find(), sum(), reduce() and match(), that return the Stream itself after its operation. This makes it possible to call these methods one after another like they are a pipeline.
For example, to select all the women who are older than 18 from a collection of people:
List<Person> peopleList = <your collection of people>
List<Person> womenOlderThan18 = peopleList.stream()
.filter(p -> p.getGender() == female && p.getAge() > 18)
.sorted(Comparator.comparingInt(Person::getAge))
.collect(Collectors.toList());
The collect() function closes the pipeline and converts the Stream to a Collection. For example, the following code will return a Map that maps the department Id to a group of people.
Map<Integer, List<Person>> deptToPepleMap = peopleList.stream()
.collect(Collectors.groupingBy(Person::getDepartmentId));
The map() function of Stream tells the function that follows it what to operate on. For example, to get the sum of the ages of all the people in the collection.
int totalAge = peopleList.stream()
.map(Person::getAge)
.sum();
To get a collection of all the IDs.
List<Integer> ids = peopleList.stream()
.map<Person::getId)
.sorted()
.collect(Collectors.toList());
Many of the Stream operations perform the same functions as the corresponding aggregate functions in a SQL statement, they are therefore also referred to as aggregate operations.
-------------------------------------------------------------------------------------------------------------
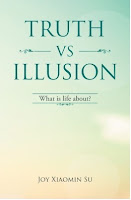
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
For example, to select all the women who are older than 18 from a collection of people:
List<Person> peopleList = <your collection of people>
List<Person> womenOlderThan18 = peopleList.stream()
.filter(p -> p.getGender() == female && p.getAge() > 18)
.sorted(Comparator.comparingInt(Person::getAge))
.collect(Collectors.toList());
The collect() function closes the pipeline and converts the Stream to a Collection. For example, the following code will return a Map that maps the department Id to a group of people.
Map<Integer, List<Person>> deptToPepleMap = peopleList.stream()
.collect(Collectors.groupingBy(Person::getDepartmentId));
The map() function of Stream tells the function that follows it what to operate on. For example, to get the sum of the ages of all the people in the collection.
int totalAge = peopleList.stream()
.map(Person::getAge)
.sum();
To get a collection of all the IDs.
List<Integer> ids = peopleList.stream()
.map<Person::getId)
.sorted()
.collect(Collectors.toList());
Many of the Stream operations perform the same functions as the corresponding aggregate functions in a SQL statement, they are therefore also referred to as aggregate operations.
-------------------------------------------------------------------------------------------------------------
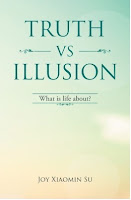
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment