The chain of responsibility pattern is used to pass a request through a chain of processors until it is resolved. It usually has a interface or abstract class that defines the common fields, request levels, and methods used by all the processors. Each implementing processor is specialized to process only requests below a certain level.
For example, a company is hiring new employees. The policy is that if the position is entry level, the team leader interviews the person and makes the decision; if it is middle level, the project leader does the interview and makes the decision; else if the position is senior level, the department chair does the interview and makes the decision.
//Create the HireProcessor common interface
public abstract class HireProcessor {
public static final int ENTRY = 1;
public static final int MIDDLE = 2;
public static final int SENIOR = 3;
protected int level;
protected HireProcessor nextProcessor
public void setNextProcessor(HireProcessor np) {
nextProcessor = np;
}
public void process(int lev) {
if (lev <= level) {
printDecision();
} else {
nextProcessor.process(lev);
}
}
protected abstract void printDecision();
}
//Create each processor
//Entry level processor
public class EntryHireProcessor extends HireProcessor {
public EntryHireProcessor(int level) {
this.level = level;
}
protected void printDecision() {
System.out.println("Hire as an entry level employee.");
}
}
//Middle level processor
public class MiddleHireProcessor extends HireProcessor {
public MiddleHireProcessor(int level) {
this.level = level;
}
protected void printDecision() {
System.out.println("Hire as a middle level employee.");
}
}
//Senior level processor
public class SeniorHireProcessor extends HireProcessor {
public SeniorHireProcessor(int level) {
this.level = level;
}
protected void printDecision() {
System.out.println("Hire as a senior level employee.");
}
}
//The Runner
public class ChainDemo {
public static void main(String[] args) {
//create the chain
HireProcessor ep = new EntryHireProcessor(HireProcessor.ENTRY);
HireProcessor mp = new MiddleHireProcessor(HireProcessor.MIDDLE);
HireProcessor sp = new SeniorHireProcessor(HireProcessor.SENIOR);
ep.setNextProcessor(mp);
mp.setNextProcessor(sp);
//call the first element in the chain to process a request
ep.process(HireProcessor.SENIOR);
ep.process(HireProcessor.MIDDLE);
ep.process(HireProcessor.ENTRY);
ep.process(HireProcessor.SENIOR);
}
}
-----------------------------------------------------------------------------------------------------------------
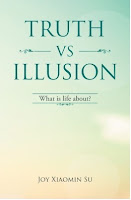
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
For example, a company is hiring new employees. The policy is that if the position is entry level, the team leader interviews the person and makes the decision; if it is middle level, the project leader does the interview and makes the decision; else if the position is senior level, the department chair does the interview and makes the decision.
//Create the HireProcessor common interface
public abstract class HireProcessor {
public static final int ENTRY = 1;
public static final int MIDDLE = 2;
public static final int SENIOR = 3;
protected int level;
protected HireProcessor nextProcessor
public void setNextProcessor(HireProcessor np) {
nextProcessor = np;
}
public void process(int lev) {
if (lev <= level) {
printDecision();
} else {
nextProcessor.process(lev);
}
}
protected abstract void printDecision();
}
//Create each processor
//Entry level processor
public class EntryHireProcessor extends HireProcessor {
public EntryHireProcessor(int level) {
this.level = level;
}
protected void printDecision() {
System.out.println("Hire as an entry level employee.");
}
}
//Middle level processor
public class MiddleHireProcessor extends HireProcessor {
public MiddleHireProcessor(int level) {
this.level = level;
}
protected void printDecision() {
System.out.println("Hire as a middle level employee.");
}
}
//Senior level processor
public class SeniorHireProcessor extends HireProcessor {
public SeniorHireProcessor(int level) {
this.level = level;
}
protected void printDecision() {
System.out.println("Hire as a senior level employee.");
}
}
//The Runner
public class ChainDemo {
public static void main(String[] args) {
//create the chain
HireProcessor ep = new EntryHireProcessor(HireProcessor.ENTRY);
HireProcessor mp = new MiddleHireProcessor(HireProcessor.MIDDLE);
HireProcessor sp = new SeniorHireProcessor(HireProcessor.SENIOR);
ep.setNextProcessor(mp);
mp.setNextProcessor(sp);
//call the first element in the chain to process a request
ep.process(HireProcessor.SENIOR);
ep.process(HireProcessor.MIDDLE);
ep.process(HireProcessor.ENTRY);
ep.process(HireProcessor.SENIOR);
}
}
-----------------------------------------------------------------------------------------------------------------
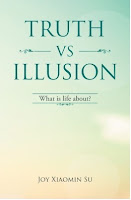
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment