It is a good manner to put common code used by sub classes in the super class to avoid repeating code, which makes it easier and less error prone for code modification. However, sometimes it requires to create new instances of the subclass in the super class. The following code shows how to achieve that.
//the super class
public abstract class AClass {
protected DoTheJob theDoer;
public AClass() {
}
protected abstract class DoTheJob{
protected abstract void dothejob() ;
}
public void performing() {
//do part 1 of performing
//now need a new instance of DoTheJob
//Method: using the factory design pattern
theDoer = createNewDoer();
theDoer.dothjob();
}
//The factory method to create a new instance of the current subclass of DoTheJob
protected abstract DoTheJob createNewDoer() ;
}
//subclass of AClass
public class BClass extends AClass{
public BClass() {
theDoer = new BDoTheJob();
}
protected DoTheJob createNewDoer() {
return new BDoTheJob();
}
protected class BDoTheJob extends DoTheJob{
protected void dothejob() {
System.out.println("do the job in BClass");
}
}
public static void main(String[] args) {
AClass theClass = new BClass();
theClass.performing();
}
}
//subclass of AClass
public class CClass extends AClass {
public CClass() {
theDoer = new CDoTheJob();
}
protected DoTheJob createNewDoer() {
return new CDoTheJob();
}
protected class CDoTheJob extends DoTheJob{
protected void dothejob() {
System.out.println("do the job in CClass");
}
}
}
//the runner
public class TheRunner {
public static void main(String[] args) {
AClass theClass1 = new BClass();
AClass theClass2 = new CClass();
theClass1.performing();
theClass2.performing();
}
}
---------------------------------------------------------------------------------------------------------------
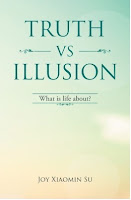
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
//the super class
public abstract class AClass {
protected DoTheJob theDoer;
public AClass() {
}
protected abstract class DoTheJob{
protected abstract void dothejob() ;
}
public void performing() {
//do part 1 of performing
//now need a new instance of DoTheJob
//Method: using the factory design pattern
theDoer = createNewDoer();
theDoer.dothjob();
}
//The factory method to create a new instance of the current subclass of DoTheJob
protected abstract DoTheJob createNewDoer() ;
}
//subclass of AClass
public class BClass extends AClass{
public BClass() {
theDoer = new BDoTheJob();
}
protected DoTheJob createNewDoer() {
return new BDoTheJob();
}
protected class BDoTheJob extends DoTheJob{
protected void dothejob() {
System.out.println("do the job in BClass");
}
}
public static void main(String[] args) {
AClass theClass = new BClass();
theClass.performing();
}
}
//subclass of AClass
public class CClass extends AClass {
public CClass() {
theDoer = new CDoTheJob();
}
protected DoTheJob createNewDoer() {
return new CDoTheJob();
}
protected class CDoTheJob extends DoTheJob{
protected void dothejob() {
System.out.println("do the job in CClass");
}
}
}
//the runner
public class TheRunner {
public static void main(String[] args) {
AClass theClass1 = new BClass();
AClass theClass2 = new CClass();
theClass1.performing();
theClass2.performing();
}
}
---------------------------------------------------------------------------------------------------------------
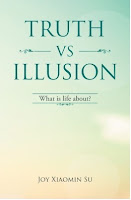
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment