The singleton pattern is used for ensuring that no more than one instance of a class type exists in an application system. The single instance can be created at the time of usage or at class loading. The former is known as lazy initialization and the later is eager initialization. The key point of the singleton pattern is that the constructor must be either private or protected, which means that the user can not directly call the constructor.
public class Test {
private static Test test = null;
//synchronized to ensure that no more than one instance is created at the same time
public static synchronized Test getInstance() {
if (test == null) {
test = new Test();
}
return test;
}
private Test() {
//constructing code
}
public static void main(String[] args) {
Test test = getInstance();
}
}
public class Test {
private static Test test = new Test();
public static Test getInstance() {
return test;
}
private Test() {
//constructing code
}
}
If the constructor throws exception or the initiation needs other processing, a static block can be used to instantiate the class.
public class Test {
private Test() throws Exception {
//constructing code
}
private static Test test;
static {
try {
test = new Test();
} catch (Exception e) {
//code to precess the exception
}
}
public static Test getInstance() {
return test;
}
}
-----------------------------------------------------------------------------------------------------------------
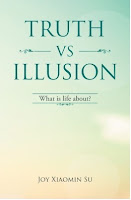
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
1. Lazy Initialization
public class Test {
private static Test test = null;
//synchronized to ensure that no more than one instance is created at the same time
public static synchronized Test getInstance() {
if (test == null) {
test = new Test();
}
return test;
}
private Test() {
//constructing code
}
public static void main(String[] args) {
Test test = getInstance();
}
}
2. Eager Initialization
public class Test {
private static Test test = new Test();
public static Test getInstance() {
return test;
}
private Test() {
//constructing code
}
}
If the constructor throws exception or the initiation needs other processing, a static block can be used to instantiate the class.
public class Test {
private Test() throws Exception {
//constructing code
}
private static Test test;
static {
try {
test = new Test();
} catch (Exception e) {
//code to precess the exception
}
}
public static Test getInstance() {
return test;
}
}
-----------------------------------------------------------------------------------------------------------------
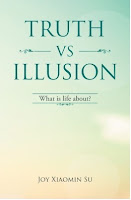
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment