The composite pattern is used when a hierarchy of objects have common characters recursively happening along the hierarchy.
For example, the United Nation has countries, a country has states, a state has cities as its jurisdiction units, all of which have a name, area, population, etc. This can be implemented using the composite pattern.
public abstract class JurisdictionUnit {
protected String name;
protected long area;
protected long population;
public JurisdictionUnit(String n, long a, long p){
name = n;
area = a;
population = p;
}
public abstract List<? extends JurisdictionUnit> getJurisdictionUnits();
public String toString() {
return ("Name: " + name + ", Area: " + area + ", Population: " + population);
}
}
public class City extends JurisdictionUnit {
public List<JurisdictionUnit> getJurisdictionUnits() {
return new ArrayList<JurisdictionUnit>();
}
}
public class State extends JurisdictionUnit{
private ArrayList<City> cities;
public State(String n, long a, long p, ArrayList<City> c) {
super (n, a, p);
cities = c;
}
public List<City> getJurisdictionUnits() {
return cities;
}
}
public class Country extends JurisdictionUnit{
private ArrayList<State> states;
public Country(String n, long a, long p, ArrayList<State> s) {
super (n, a, p);
states = s;
}
public List<State> getJurisdictionUnits() {
return states;
}
}
public class CountryCompositeDemo {
public static void main(String[] args) {
ArrayList<City> cityArray1 = new ArrayList<City>();
cityArray1.add (new City ("Edan", 100L, 50L));
cityArray1.add (new City ("Lincoln", 500L, 200L));
State state1 = new State("StateOne", 600L, 250L, cityArray1);
ArrayList<City> cityArray2 = new ArrayList<City>();
cityArray1.add (new City ("Haloo", 100L, 50L));
cityArray1.add (new City ("Orca", 500L, 200L));
State state2 = new State("StateTwo", 600L, 250L, cityArray2);
ArrayList<State> stateArray = new ArrayList<State>();
stateArray.add(state1);
stateArray.add(state2);
Country luckyCountry = new Country("Lucky", 1200L, 500L, stateArray);
System.out.println("The country: " + luckyCountry);
System.out.println("The States :");
for (State s : luckyCountry.getJurisdictionUnits()) {
System.out.println(s);
System.out.println("The Cities : ");
for (City c : s.getJurisdictionUnits()) {
System.out.println(c);
}
}
}
}
A family tree can be another example. The first generation, second, third,..... generations can all have children, and all of them are persons, share the characters and behaviors of a person. The administration hierarchy of an organization is surely another example.
---------------------------------------------------------------------------------------------------------------
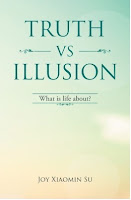
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
For example, the United Nation has countries, a country has states, a state has cities as its jurisdiction units, all of which have a name, area, population, etc. This can be implemented using the composite pattern.
public abstract class JurisdictionUnit {
protected String name;
protected long area;
protected long population;
public JurisdictionUnit(String n, long a, long p){
name = n;
area = a;
population = p;
}
public abstract List<? extends JurisdictionUnit> getJurisdictionUnits();
public String toString() {
return ("Name: " + name + ", Area: " + area + ", Population: " + population);
}
}
public class City extends JurisdictionUnit {
public List<JurisdictionUnit> getJurisdictionUnits() {
return new ArrayList<JurisdictionUnit>();
}
}
public class State extends JurisdictionUnit{
private ArrayList<City> cities;
public State(String n, long a, long p, ArrayList<City> c) {
super (n, a, p);
cities = c;
}
public List<City> getJurisdictionUnits() {
return cities;
}
}
public class Country extends JurisdictionUnit{
private ArrayList<State> states;
public Country(String n, long a, long p, ArrayList<State> s) {
super (n, a, p);
states = s;
}
public List<State> getJurisdictionUnits() {
return states;
}
}
public class CountryCompositeDemo {
public static void main(String[] args) {
ArrayList<City> cityArray1 = new ArrayList<City>();
cityArray1.add (new City ("Edan", 100L, 50L));
cityArray1.add (new City ("Lincoln", 500L, 200L));
State state1 = new State("StateOne", 600L, 250L, cityArray1);
ArrayList<City> cityArray2 = new ArrayList<City>();
cityArray1.add (new City ("Haloo", 100L, 50L));
cityArray1.add (new City ("Orca", 500L, 200L));
State state2 = new State("StateTwo", 600L, 250L, cityArray2);
ArrayList<State> stateArray = new ArrayList<State>();
stateArray.add(state1);
stateArray.add(state2);
Country luckyCountry = new Country("Lucky", 1200L, 500L, stateArray);
System.out.println("The country: " + luckyCountry);
System.out.println("The States :");
for (State s : luckyCountry.getJurisdictionUnits()) {
System.out.println(s);
System.out.println("The Cities : ");
for (City c : s.getJurisdictionUnits()) {
System.out.println(c);
}
}
}
}
A family tree can be another example. The first generation, second, third,..... generations can all have children, and all of them are persons, share the characters and behaviors of a person. The administration hierarchy of an organization is surely another example.
---------------------------------------------------------------------------------------------------------------
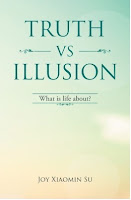
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
good explanation and example..
ReplyDeleteYour blog is great, keep up the good work.
ReplyDeleteThank you very much for your comment.
Delete