The Java factory pattern is based on polymorphic method call of type inheritance to create instance of the appropriate type. The purpose of using the factory pattern is to provide user a common user interface across a group of sub types that share a common super type. Following sample code is an implementation of the factory pattern.
//super class can be an interface or abstract class
public class CarFactory {
public Car createCar() {
return new Car();
}
}
public class SportCarFactory extends CarFactory {
public Car createCar() {
return new SportCar();
}
}
public class SedanCarFactory extends CarFactory {
public Car createCar() {
return new SedanCar();
}
}
//call factory.createCar() will automatically create the proper type of car
public class CarShop {
private CarFactory factory;
public Car createSportCar() {
factory = new SportCarFactory();
factory.createCar();
}
public Car createSedanCar() {
factory = new SedanCarFactory();
facotry.createCar();
}
}
References:
1. Factory method pattern
-------------------------------------------------------------------------------------------------------------
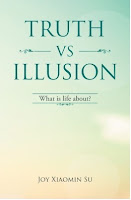
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
//super class can be an interface or abstract class
public class CarFactory {
public Car createCar() {
return new Car();
}
}
public class SportCarFactory extends CarFactory {
public Car createCar() {
return new SportCar();
}
}
public class SedanCarFactory extends CarFactory {
public Car createCar() {
return new SedanCar();
}
}
//call factory.createCar() will automatically create the proper type of car
public class CarShop {
private CarFactory factory;
public Car createSportCar() {
factory = new SportCarFactory();
factory.createCar();
}
public Car createSedanCar() {
factory = new SedanCarFactory();
facotry.createCar();
}
}
References:
1. Factory method pattern
-------------------------------------------------------------------------------------------------------------
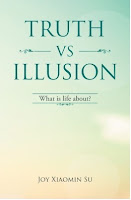
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment