The bridge pattern is used to separate interface from implementation so that when the implementation is modified, users don't have to change their code.
//The bridge interface
public interface Employee {
public long getId(String name);
}
//Implementations - the bridges that hide the implementation details
public class Guard implements Employee {
public long getId(String name) {
//return guardId;
}
}
public class Executive implements Employee {
public long getId(String name) {
//return executiveId;
}
}
//User
public abstract class EmployeeOnDuty {
protected Employee emp;
protected EmployeeOnDuty (Employee emp) {
this.emp = emp;
}
public abstract boolean checkEmployeeOnDuty();
}
public class GuardOnDuty extends EmployeeOnDuty {
String name = "";
public GuardOnDuty(Employee emp, String name) {
super (emp);
this.name = name;
}
public boolean checkEmployeeOnDuty() {
long id = emp.getId(name);
//if id is on schedule
return true;
}
}
public class ExecutiveOnDuty extends EmployeeOnDuty {
String name = "";
String title = "";
public ExecutiveOnDuty (Employee emp, String name, String title) {
super (emp);
this.name = name;
this.title = title;
}
public boolean checkEmployeeOnDuty() {
long id = emp.getId(name);
//if title is the right one and id is scheduled on duty
return true;
}
}
In case that the getId() needs an update due to database structure change, you only need to update the implementation classes, the user classes require no modification. On the other hand if the users change the way of using Employee, e.g. passing Employee to the checkEmployeeOnDuty method instead of passing it to the constructor, the Employee and its implementations do not need modification.
---------------------------------------------------------------------------------------------------------------
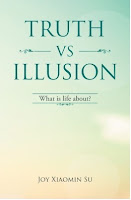
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
//The bridge interface
public interface Employee {
public long getId(String name);
}
//Implementations - the bridges that hide the implementation details
public class Guard implements Employee {
public long getId(String name) {
//return guardId;
}
}
public class Executive implements Employee {
public long getId(String name) {
//return executiveId;
}
}
//User
public abstract class EmployeeOnDuty {
protected Employee emp;
protected EmployeeOnDuty (Employee emp) {
this.emp = emp;
}
public abstract boolean checkEmployeeOnDuty();
}
public class GuardOnDuty extends EmployeeOnDuty {
String name = "";
public GuardOnDuty(Employee emp, String name) {
super (emp);
this.name = name;
}
public boolean checkEmployeeOnDuty() {
long id = emp.getId(name);
//if id is on schedule
return true;
}
}
public class ExecutiveOnDuty extends EmployeeOnDuty {
String name = "";
String title = "";
public ExecutiveOnDuty (Employee emp, String name, String title) {
super (emp);
this.name = name;
this.title = title;
}
public boolean checkEmployeeOnDuty() {
long id = emp.getId(name);
//if title is the right one and id is scheduled on duty
return true;
}
}
In case that the getId() needs an update due to database structure change, you only need to update the implementation classes, the user classes require no modification. On the other hand if the users change the way of using Employee, e.g. passing Employee to the checkEmployeeOnDuty method instead of passing it to the constructor, the Employee and its implementations do not need modification.
---------------------------------------------------------------------------------------------------------------
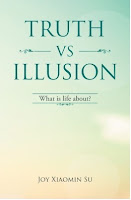
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment